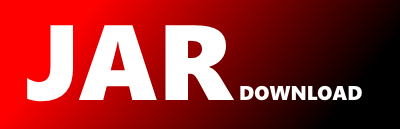
com.natpryce.result.kt Maven / Gradle / Ivy
package com.natpryce
/**
* A result of a computation that can succeed or fail.
*/
sealed class Result
data class Ok(val value: T) : Result()
data class Err(val reason: E) : Result()
/**
* Call a function and wrap the result in a Result, catching any Exception and returning it as Err value.
*/
inline fun resultFrom(block: () -> T): Result =
try {
Ok(block())
}
catch (x: Exception) {
Err(x)
}
/**
* Map a function over the _value_ of a successful Result.
*/
inline fun Result.map(f: (T) -> U): Result = when (this) {
is Ok -> Ok(f(value))
is Err -> this
}
/**
* Flat-map a function over the _value_ of a successful Result.
*/
inline fun Result.flatMap(f: (T) -> Result): Result = when (this) {
is Ok -> f(value)
is Err -> this
}
/**
* Map a function over the _reason_ of an unsuccessful Result.
*/
inline fun Result.mapError(f: (E) -> F): Result = when (this) {
is Ok -> this
is Err -> Err(f(reason))
}
/**
* Flat-map a function over the _reason_ of a unsuccessful Result.
*/
inline fun Result.flatMapError(f: (E) -> Result): Result = when (this) {
is Ok -> this
is Err -> f(reason)
}
/**
* Unwrap a Result in which both the success and error values have the same type, returning a plain value.
*/
fun Result.get() = when (this) {
is Ok -> value
is Err -> reason
}
/**
* Unwrap a Result, by returning the success value or calling _block_ on error to abort from the current function.
*/
inline fun Result.onError(block: (Err) -> Nothing): T = when (this) {
is Ok -> value
is Err -> block(this)
}
/**
* Unwrap a Result by returning the success value or calling _errorToValue_ to mapping the error reason to a plain value.
*/
inline fun Result.recover(errorToValue: (E) -> U): S = when (this) {
is Ok -> value
is Err -> errorToValue(reason)
}
/**
* Perform a side effect with the success value.
*/
inline fun Result.peek(f: (T) -> Unit) =
apply { if (this is Ok) f(value) }
/**
* Perform a side effect with the error reason.
*/
inline fun Result.peekError(f: (E) -> Unit) =
apply { if (this is Err) f(reason) }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy