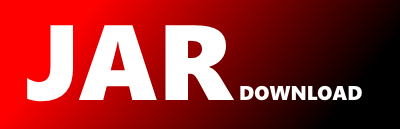
com.natpryce.snodge.internal.JsonFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snodge-standalone Show documentation
Show all versions of snodge-standalone Show documentation
A small, extensible Java library to randomly mutate JSON documents. Useful for fuzz testing.
package com.natpryce.snodge.internal;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import java.util.AbstractMap;
import java.util.Map;
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class JsonFunctions {
public static Map.Entry entry(K key, V value) {
return new AbstractMap.SimpleEntry(key, value);
}
public static IntStream indices(JsonArray array) {
return IntStream.range(0, array.size());
}
public static Stream> arrayEntries(final JsonArray array) {
return indices(array).mapToObj(index -> entry(index, array.get(index)));
}
public static JsonElement removeArrayElement(JsonArray original, int indexToRemove) {
JsonArray mutant = new JsonArray();
indices(original)
.filter(i -> i != indexToRemove)
.forEach(i -> mutant.add(original.get(i)));
return mutant;
}
public static JsonElement removeObjectProperty(JsonObject original, String nameToRemove) {
JsonObject mutant = new JsonObject();
original.entrySet().stream()
.filter(e -> !e.getKey().equals(nameToRemove))
.forEach(e -> mutant.add(e.getKey(), e.getValue()));
return mutant;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy