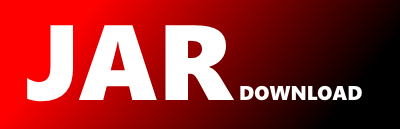
com.nequi.api.client.NequiGatewayClient Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.nequi.api.client;
import com.google.gson.JsonObject;
@com.amazonaws.mobileconnectors.apigateway.annotation.Service(endpoint = "https://kksofdo2af.execute-api.us-east-1.amazonaws.com/prod")
public interface NequiGatewayClient {
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-clientservice-validateclient", method = "POST")
JsonObject servicesClientserviceValidateclientPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-clientservice-validateclient", method = "OPTIONS")
JsonObject servicesClientserviceValidateclientOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-daysalesservice-getdaysales", method = "POST")
JsonObject servicesDaysalesserviceGetdaysalesPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-daysalesservice-getdaysales", method = "OPTIONS")
JsonObject servicesDaysalesserviceGetdaysalesOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-cashinservice-cashin", method = "POST")
JsonObject servicesCashinserviceCashinPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-cashinservice-cashin", method = "OPTIONS")
JsonObject servicesCashinserviceCashinOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-cashoutservice-cashout", method = "POST")
JsonObject servicesCashoutserviceCashoutPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-cashoutservice-cashout", method = "OPTIONS")
JsonObject servicesCashoutserviceCashoutOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-cashoutservice-cashoutconsult", method = "POST")
JsonObject servicesCashoutserviceCashoutconsultPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-cashoutservice-cashoutconsult", method = "OPTIONS")
JsonObject servicesCashoutserviceCashoutconsultOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-nequipointservice-getnequipoints", method = "POST")
JsonObject servicesNequipointserviceGetnequipointsPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-nequipointservice-getnequipoints", method = "OPTIONS")
JsonObject servicesNequipointserviceGetnequipointsOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-nequipointservice-infonequipoint", method = "POST")
JsonObject servicesNequipointserviceInfonequipointPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-nequipointservice-infonequipoint", method = "OPTIONS")
JsonObject servicesNequipointserviceInfonequipointOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-paymentservice-generatecodeqr", method = "POST")
JsonObject servicesPaymentserviceGeneratecodeqrPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-paymentservice-generatecodeqr", method = "OPTIONS")
JsonObject servicesPaymentserviceGeneratecodeqrOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-paymentservice-getstatuspayment", method = "POST")
JsonObject servicesPaymentserviceGetstatuspaymentPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-paymentservice-getstatuspayment", method = "OPTIONS")
JsonObject servicesPaymentserviceGetstatuspaymentOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-paymentservice-unregisteredpayment", method = "POST")
JsonObject servicesPaymentserviceUnregisteredpaymentPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-paymentservice-unregisteredpayment", method = "OPTIONS")
JsonObject servicesPaymentserviceUnregisteredpaymentOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-keysservice-getpublic", method = "POST")
String servicesKeysserviceGetpublicPost();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-keysservice-getpublic", method = "OPTIONS")
JsonObject servicesKeysserviceGetpublicOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-pocketservices-getpocket", method = "POST")
JsonObject servicesPocketservicesGetpocketPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-pocketservices-getpocket", method = "OPTIONS")
JsonObject servicesPocketservicesGetpocketOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-transferservices-transfer", method = "POST")
JsonObject servicesTransferservicesTransferPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-transferservices-transfer", method = "OPTIONS")
JsonObject servicesTransferservicesTransferOptions();
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-reverseservices-reversetransaction", method = "POST")
JsonObject servicesReverseservicesReversetransactionPost(JsonObject body);
@com.amazonaws.mobileconnectors.apigateway.annotation.Operation(path = "/-services-reverseservices-reversetransaction", method = "OPTIONS")
JsonObject servicesReverseservicesReversetransactionOptions();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy