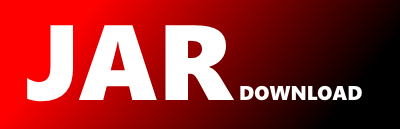
com.netapp.santricity.api.symbol.GApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of santricity-java-client Show documentation
Show all versions of santricity-java-client Show documentation
The NetApp SANtricity WebAPI - Java SDK client library is a open source SDK that facilitate access to the
NetApp E-Series storage system for automation and integration into third-party web or script-based management tools.
The newest version!
/**************************************************************************************************************************************************************
* The Clear BSD License
*
* Copyright (c) – 2016, NetApp, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted (subject to the limitations in the disclaimer below) provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
*
* * Neither the name of NetApp, Inc. nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
*
* NO EXPRESS OR IMPLIED LICENSES TO ANY PARTY'S PATENT RIGHTS ARE GRANTED BY THIS LICENSE. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*************************************************************************************************************************************************************/
package com.netapp.santricity.api.symbol;
import com.netapp.santricity.ApiException;
import com.netapp.santricity.ApiClient;
import com.netapp.santricity.Configuration;
import com.netapp.santricity.models.v2.*;
import com.netapp.santricity.models.symbol.*;
import com.netapp.santricity.models.utils.*;
import com.netapp.santricity.Pair;
import com.netapp.santricity.StringUtil;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import javax.ws.rs.core.GenericType;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@javax.annotation.Generated(value = "class com.ni.aa.client.codegen.lang.JavaNetappClientCodegen", date = "2017-10-04T15:05:55.769-05:00")
public class GApi {
private ApiClient apiClient;
public GApi() {
this(Configuration.getDefaultApiClient());
}
public GApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Generates a Key Management Server (KMS) Client Certificate Signing Request (CSR) that needs to be signed by a Certificate Authority (CA). The resulting signed certificate or client certificate is installed on the storage array for authenticating with the KMIP server.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return KMSCertificateFileReturn
* @throws ApiException if fails to make API call
*/
public KMSCertificateFileReturn symbolGenerateCertificateSigningRequest(String systemId, KMSClientCSRDescriptor body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGenerateCertificateSigningRequest");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGenerateCertificateSigningRequest");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/generateCertificateSigningRequest".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Allows a client to fetch a set of entries that have the alertable, needs attention, or collect support bundle flag set from the array's MEL for analysis or display.
* Documented return codes: ok, error.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body The range of MEL entries. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return MelEntryList
* @throws ApiException if fails to make API call
*/
public MelEntryList symbolGetAlertableMelEntries(String systemId, MelExtent body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAlertableMelEntries");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetAlertableMelEntries");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAlertableMelEntries".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure is used to get the current Autosupport status values.
* Documented return codes: ok, notImplemented.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return AsupStatusReturned
* @throws ApiException if fails to make API call
*/
public AsupStatusReturned symbolGetAsupStatus(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAsupStatus");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAsupStatus".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure returns information on the connections established to a remote array for an existing AsyncMirrorGroup. This will return connection information for both controllers.
* Documented return codes: ok, notImplemented.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ScsiRemoteConnectionsReturned
* @throws ApiException if fails to make API call
*/
public ScsiRemoteConnectionsReturned symbolGetAsyncMirrorGroupRemoteConnections(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAsyncMirrorGroupRemoteConnections");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetAsyncMirrorGroupRemoteConnections");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAsyncMirrorGroupRemoteConnections".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure will return sync progress information for Async Mirror Groups and their associated members. Calls to this procedure are valid even when a sync is not running.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A list of Asynchronous Mirror Group references. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return AsyncMirrorGroupSyncProgressListReturned
* @throws ApiException if fails to make API call
*/
public AsyncMirrorGroupSyncProgressListReturned symbolGetAsyncMirrorGroupSyncProgress(String systemId, AsyncMirrorGroupRefList body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAsyncMirrorGroupSyncProgress");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetAsyncMirrorGroupSyncProgress");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAsyncMirrorGroupSyncProgress".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure will return repository utilization information for async mirror members.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A list of Asynchronous Mirror Group member references. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return AsyncMirrorRepositoryUtilizationListReturned
* @throws ApiException if fails to make API call
*/
public AsyncMirrorRepositoryUtilizationListReturned symbolGetAsyncMirrorRepositoryUtilization(String systemId, AsyncMirrorGroupMemberRefList body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAsyncMirrorRepositoryUtilization");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetAsyncMirrorRepositoryUtilization");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAsyncMirrorRepositoryUtilization".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure will return synchronization time statistics for a list of mirror members.
* Documented return codes: ok, notImplemented.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return AsyncMirrorSyncStatisticsListReturned
* @throws ApiException if fails to make API call
*/
public AsyncMirrorSyncStatisticsListReturned symbolGetAsyncMirrorSyncStatistics(String systemId, AsyncMirrorSyncStatisticsRequestDescriptor body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAsyncMirrorSyncStatistics");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetAsyncMirrorSyncStatistics");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAsyncMirrorSyncStatistics".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure automatically gets the configuration candidates.
* Documented return codes: ok, illegalParam, noHeap, driveNotExist, internalError, invalidSegmentsize, raid6FeatureUnsupported, raid6FeatureDisabled.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body This object contains a list of automatic configuration templates. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return AutoConfigCandidateList
* @throws ApiException if fails to make API call
*/
public AutoConfigCandidateList symbolGetAutoConfigCandidates(String systemId, AutoConfigTemplateList body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetAutoConfigCandidates");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetAutoConfigCandidates");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getAutoConfigCandidates".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieves the status of a running, interrupted, or completed base controller diagnostic test.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return FruDiagReturn
* @throws ApiException if fails to make API call
*/
public FruDiagReturn symbolGetBaseControllerDiagnosticStatus(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetBaseControllerDiagnosticStatus");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getBaseControllerDiagnosticStatus".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieves the status of a running, interrupted, or completed cache backup device diagnostic test.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return FruDiagReturn
* @throws ApiException if fails to make API call
*/
public FruDiagReturn symbolGetCacheBackupDeviceDiagnosticStatus(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCacheBackupDeviceDiagnosticStatus");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCacheBackupDeviceDiagnosticStatus".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieves the status of a running, interrupted, or completed cache memory diagnostic test.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return FruDiagReturn
* @throws ApiException if fails to make API call
*/
public FruDiagReturn symbolGetCacheMemoryDiagnosticStatus(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCacheMemoryDiagnosticStatus");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCacheMemoryDiagnosticStatus".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure is used by clients to query the controller for indications of state changes that have occurred and which would necessitate that the client refresh its view of the storage array's state. If no state changes need to be reported, the controller will generally hold this request, without responding, for up to the amount of time specified in the argument. If, at any time during the hold period, a state change occurs, the controller will return immediately with the new configuration generation number and/or MEL sequence number. By using this hanging poll approach, the amount of traffic between the client and the server is reduced to an insignificant level, and yet near-immediate notification of changes is still possible.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A ChangeQueryDescriptor object that identifies the caller's current understanding of the storage array's state, along with an indication of the maximum hold time for this request. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ChangeState
* @throws ApiException if fails to make API call
*/
public ChangeState symbolGetChangeState(String systemId, ChangeQueryDescriptor body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetChangeState");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetChangeState");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getChangeState".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure reports the results of drive channel fault isolation diagnostic tests that were initiated by the startChannelDIagnostics procedure.
* Documented return codes: ok, channelDiagsRunning, channelDiagsResultsPartial, channelDiagsResultsNotAvailable.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ChannelDiagResultsReturned
* @throws ApiException if fails to make API call
*/
public ChannelDiagResultsReturned symbolGetChannelDiagnosticsResults(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetChannelDiagnosticsResults");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getChannelDiagnosticsResults".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedures returns the performance limitation values for a host cluster. Those values include the limits on the IOPs and throughput (MB/s). The number of IOPs impacted by the performance limits is also returned.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body This procedure takes a cluster reference as an input parameter. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ClusterPerformanceLimitationValuesReturned
* @throws ApiException if fails to make API call
*/
public ClusterPerformanceLimitationValuesReturned symbolGetClusterPerformanceLimitationValues(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetClusterPerformanceLimitationValues");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetClusterPerformanceLimitationValues");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getClusterPerformanceLimitationValues".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure replaces the deprecated stateCapture procedure. It returns a single \"chunk\" of debug information and must be used in a series of like calls in order to retrieve the complete set of debug information.
* Documented return codes: ok, illegalParam, tryAlternate, noSuchDebugChunk, debugInfoConfigChanged.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A DebugInfoExtent structure containing three pieces of information: a reference to the controller that is to return the data, a \"handle\" identifying a single mutually-consistent set of chunks, and a chunk number that identifies the specific chunk to be returned. On the request for the initial chunk (chunk zero), the handle is not significant. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DebugInfoReturned
* @throws ApiException if fails to make API call
*/
public DebugInfoReturned symbolGetControllerDebugInformation(String systemId, DebugInfoExtent body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetControllerDebugInformation");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetControllerDebugInformation");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getControllerDebugInformation".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure is used by clients to query the referenced controller for up-to-date information about its host-side I/O interfaces. Since this interface information is highly volatile, the object graph data contains only a point-in-time snapshot of the interface data. This procedure can be used to obtain current information that may not be reflected in the most recent object graph.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return IOInterfaceTypeDataList
* @throws ApiException if fails to make API call
*/
public IOInterfaceTypeDataList symbolGetControllerHostInterfaces(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetControllerHostInterfaces");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getControllerHostInterfaces".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* NOTE: object graph data may not be the most recent.
* Documented return codes: ok, tryAlternate.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A reference to the controller for which the I/O interface information is desired. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ControllerHostIoInterfacesReturned
* @throws ApiException if fails to make API call
*/
public ControllerHostIoInterfacesReturned symbolGetControllerHostIoInterfaces(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetControllerHostIoInterfaces");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetControllerHostIoInterfaces");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getControllerHostIoInterfaces".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure allows a client to fetch the data that resides within a designated region of the controller's NVSRAM. Note that the NVSRAM data is obtained only from the controller to which this request is directed.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body An NVSRAMRegionId value that identifies the region whose data is to be retrieved. The caller is allowed to specify that all NVSRAM regions be obtained, if desired. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return NVSRAMRegionList
* @throws ApiException if fails to make API call
*/
public NVSRAMRegionList symbolGetControllerNVSRAM(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetControllerNVSRAM");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetControllerNVSRAM");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getControllerNVSRAM".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure gets the internal clock from the controllers. The time is expressed in seconds since midnight (GMT) on 1/1/1970.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ControllerTime
* @throws ApiException if fails to make API call
*/
public ControllerTime symbolGetControllerTime(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetControllerTime");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getControllerTime".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure allows a client to fetch a set of entries with a priority value of EVENT_PRIORITY_CRITICAL from the storage array's Major Event Log for analysis or display. If there are less critical MEL events than MEL_MAX_XFER_COUNT, all the critical events will be displayed on the first call. If the client receives a number of critical MEL events that is equal to MEL_MAX_XFER_COUNT, then the user must increment the first sequence number to be the highest sequence number in the list of entries returned and repeat the procedure. This process must be repeated until the number of entries returned is less than MEL_MAX_XFER_COUNT.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A MelExtent object that provides the sequence number of the first and last MEL entries to be retrieved. All critical entries between these two numbers (inclusive) will be transferred to the caller as the result of the operation. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return MelEntryList
* @throws ApiException if fails to make API call
*/
public MelEntryList symbolGetCriticalMelEntries(String systemId, MelExtent body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCriticalMelEntries");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetCriticalMelEntries");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCriticalMelEntries".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Returns a structure containing information about the controller if it is locked down.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return LockDownInfoReturned
* @throws ApiException if fails to make API call
*/
public LockDownInfoReturned symbolGetCtlLockDownInfo(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCtlLockDownInfo");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCtlLockDownInfo".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure returns up to two groups of statistical counters, one per controller, bundled together. A counter group is a set of related statistical counters, accumulated over a period of time, along with the associated base time.
* Documented return codes: ok, error, illegalParam, invalidRequest.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A CumulativeStatisticsDescriptor structure describing the statistics of interest. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return CumulativeStatisticsBundleReturned
* @throws ApiException if fails to make API call
*/
public CumulativeStatisticsBundleReturned symbolGetCumulativeStatisticsBundle(String systemId, CumulativeStatisticsDescriptor body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCumulativeStatisticsBundle");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetCumulativeStatisticsBundle");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCumulativeStatisticsBundle".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure is used to request a list of bundles of statistical data. The argument describes the statistics being requested.
* Documented return codes: ok, error, illegalParam, invalidRequest.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body An object containing all of the required elements for the procedure. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return CumulativeStatisticsBundleListReturned
* @throws ApiException if fails to make API call
*/
public CumulativeStatisticsBundleListReturned symbolGetCumulativeStatisticsBundles(String systemId, CumulativeStatisticsListDescriptor body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCumulativeStatisticsBundles");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetCumulativeStatisticsBundles");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCumulativeStatisticsBundles".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure collects the wear life statistic information for the requested devices and returns this up to date information.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body Descriptor specifying details of the get current SSD wear life statistics request. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return CurrentSSDWearLifeStatsResults
* @throws ApiException if fails to make API call
*/
public CurrentSSDWearLifeStatsResults symbolGetCurrentSSDWearLifeStats(String systemId, CurrentSSDWearLifeStatsDescriptor body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetCurrentSSDWearLifeStats");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetCurrentSSDWearLifeStats");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getCurrentSSDWearLifeStats".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure is used to request the information about a DPL core dump. The parameter is a DPL Core Dump Tag. The value of the tag is unique for each core dump and may be obtained from the DPLCoreDumpData structure in StorageArray.
* Documented return codes: ok, dplCoreDumpInvalidTag.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body The integer value of the dplCoreDumpTag for which you are requesting information. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DPLCoreDumpInfoReturn
* @throws ApiException if fails to make API call
*/
public DPLCoreDumpInfoReturn symbolGetDPLCoreDumpInformation(String systemId, Integer body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDPLCoreDumpInformation");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDPLCoreDumpInformation");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDPLCoreDumpInformation".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure gets a list of volumes encroaching the requested Dacstore area.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A number representing the size of a Dacstore in bytes. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return AbstractVolRefList
* @throws ApiException if fails to make API call
*/
public AbstractVolRefList symbolGetDacstoreIncompatibleVolumes(String systemId, Long body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDacstoreIncompatibleVolumes");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDacstoreIncompatibleVolumes");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDacstoreIncompatibleVolumes".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure is used to retrieve stable store database metadata.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DatabaseMetadataReturned
* @throws ApiException if fails to make API call
*/
public DatabaseMetadataReturned symbolGetDatabaseMetadata(String systemId, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDatabaseMetadata");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDatabaseMetadata".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure allows a client to retrieve diagnostic information from the storage array's diagnostic data capture. The client must specify a correct tag and appropriate chunk number. In case of failure, the client must either (1) retry the current chunk number or (2) discard any partially-received log data and retry from the first chunk number (which is one - zero is not a valid chunk number).
* Documented return codes: ok, illegalParam, ddcUnavail, invalidDdcTag.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A DdcExtent structure specifying the chunk to be retrieved. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DdcLogDataReturned
* @throws ApiException if fails to make API call
*/
public DdcLogDataReturned symbolGetDdcLog(String systemId, DdcExtent body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDdcLog");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDdcLog");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDdcLog".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This returns up to two groups of discrete time series statistics, one per controller
* Documented return codes: ok, error, illegalParam, invalidRequest.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A StatStreamId object that identifies the discrete time series statistics to return. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DiscreteTimeSeriesBundleReturned
* @throws ApiException if fails to make API call
*/
public DiscreteTimeSeriesBundleReturned symbolGetDiscreteTimeSeriesBundle(String systemId, Integer body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDiscreteTimeSeriesBundle");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDiscreteTimeSeriesBundle");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDiscreteTimeSeriesBundle".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure returns a list of drive sets that are candidates for use in expanding the given Disk Pool.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A reference to the Disk Pool to be expanded. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return ExpansionCandidatesReturned
* @throws ApiException if fails to make API call
*/
public ExpansionCandidatesReturned symbolGetDiskPoolExpansionCandidates(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDiskPoolExpansionCandidates");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDiskPoolExpansionCandidates");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDiskPoolExpansionCandidates".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure will return the maximum Reserved Drive Count possible on a disk pool.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body A reference to the Disk Pool for which you want the maximum reserved drive count. (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DiskPoolMaxReservedDriveCountReturn
* @throws ApiException if fails to make API call
*/
public DiskPoolMaxReservedDriveCountReturn symbolGetDiskPoolMaxReservedDriveCount(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDiskPoolMaxReservedDriveCount");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDiskPoolMaxReservedDriveCount");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDiskPoolMaxReservedDriveCount".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "controller", controller));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "verboseErrorResponse", verboseErrorResponse));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* This procedure calculates the maximum number of drives that can be removed from a disk pool.
* Documented return codes: ok.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (required)
* @param controller Controller selection (optional, default to auto)
* @param verboseErrorResponse (optional, default to true)
* @return DiskPoolReductionDriveCountReturn
* @throws ApiException if fails to make API call
*/
public DiskPoolReductionDriveCountReturn symbolGetDiskPoolReductionDriveCount(String systemId, String body, String controller, Boolean verboseErrorResponse) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling symbolGetDiskPoolReductionDriveCount");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling symbolGetDiskPoolReductionDriveCount");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/symbol/getDiskPoolReductionDriveCount".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map