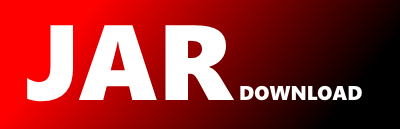
com.netapp.santricity.api.v2.VolumesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of santricity-java-client Show documentation
Show all versions of santricity-java-client Show documentation
The NetApp SANtricity WebAPI - Java SDK client library is a open source SDK that facilitate access to the
NetApp E-Series storage system for automation and integration into third-party web or script-based management tools.
The newest version!
/**************************************************************************************************************************************************************
* The Clear BSD License
*
* Copyright (c) – 2016, NetApp, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted (subject to the limitations in the disclaimer below) provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
*
* * Neither the name of NetApp, Inc. nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
*
* NO EXPRESS OR IMPLIED LICENSES TO ANY PARTY'S PATENT RIGHTS ARE GRANTED BY THIS LICENSE. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*************************************************************************************************************************************************************/
package com.netapp.santricity.api.v2;
import com.netapp.santricity.ApiException;
import com.netapp.santricity.ApiClient;
import com.netapp.santricity.Configuration;
import com.netapp.santricity.models.v2.*;
import com.netapp.santricity.models.symbol.*;
import com.netapp.santricity.models.utils.*;
import com.netapp.santricity.Pair;
import com.netapp.santricity.StringUtil;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import javax.ws.rs.core.GenericType;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@javax.annotation.Generated(value = "class com.ni.aa.client.codegen.lang.JavaNetappClientCodegen", date = "2017-10-04T15:05:52.333-05:00")
public class VolumesApi {
private ApiClient apiClient;
public VolumesApi() {
this(Configuration.getDefaultApiClient());
}
public VolumesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Perform a RAID type migration on a storage pool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @param body (optional)
* @return VolumeGroupEx
* @throws ApiException if fails to make API call
*/
public VolumeGroupEx changeRAIDType(String systemId, String id, RaidMigrationRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling changeRAIDType");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling changeRAIDType");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{id}/raid-type-migration".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Expand the capacity of a StoragePool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param poolId Storage pool id (required)
* @param body (optional)
* @return VolumeGroupEx
* @throws ApiException if fails to make API call
*/
public VolumeGroupEx expandStoragePoolCapacity(String systemId, String poolId, StoragePoolExpansionRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling expandStoragePoolCapacity");
}
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling expandStoragePoolCapacity");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{poolId}/expand".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Expand a ThinVolume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @param body (optional)
* @return ThinVolumeEx
* @throws ApiException if fails to make API call
*/
public ThinVolumeEx expandThinVolume(String systemId, String id, ThinVolumeExpansionRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling expandThinVolume");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling expandThinVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/thin-volumes/{id}/expand".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get the access volume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @return AccessVolumeEx
* @throws ApiException if fails to make API call
*/
public AccessVolumeEx getAccessVolume(String systemId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getAccessVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/access-volume".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve the list of LunMappings
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getAllLunMappings(String systemId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getAllLunMappings");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volume-mappings".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get a list of all mappable objects
* Mode: Both Embedded and Proxy. Provides a simplified way to discover the type of mappable object from an id/reference.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getAllMappableObjects(String systemId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getAllMappableObjects");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/mappable-objects".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get list of storage pools
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getAllStoragePools(String systemId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getAllStoragePools");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get the list of ThinVolumes
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getAllThinVolumes(String systemId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getAllThinVolumes");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/thin-volumes".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get the list of volumes
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getAllVolumes(String systemId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getAllVolumes");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a LunMapping
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return LUNMapping
* @throws ApiException if fails to make API call
*/
public LUNMapping getLunMapping(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getLunMapping");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getLunMapping");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volume-mappings/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get a specific mappable object
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return MappableObject
* @throws ApiException if fails to make API call
*/
public MappableObject getMappableObject(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getMappableObject");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getMappableObject");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/mappable-objects/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get a storage pool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return VolumeGroupEx
* @throws ApiException if fails to make API call
*/
public VolumeGroupEx getStoragePool(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getStoragePool");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getStoragePool");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Retrieve a list of expansion candidates for a StoragePool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param poolId Storage pool id (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getStoragePoolExpansionCandidates(String systemId, String poolId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getStoragePoolExpansionCandidates");
}
// verify the required parameter 'poolId' is set
if (poolId == null) {
throw new ApiException(400, "Missing the required parameter 'poolId' when calling getStoragePoolExpansionCandidates");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{poolId}/expand".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "poolId" + "\\}", apiClient.escapeString(poolId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Check the progress of a long-running action on a storage pool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getStoragePoolProgress(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getStoragePoolProgress");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getStoragePoolProgress");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{id}/action-progress".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get maximum number of drives that can be removed from a StoragePool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return RemovableDriveResponse
* @throws ApiException if fails to make API call
*/
public RemovableDriveResponse getStoragePoolRemovableDrives(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getStoragePoolRemovableDrives");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getStoragePoolRemovableDrives");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{id}/reduction".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get a ThinVolume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return ThinVolumeEx
* @throws ApiException if fails to make API call
*/
public ThinVolumeEx getThinVolume(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getThinVolume");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getThinVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/thin-volumes/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get a specific volume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return VolumeEx
* @throws ApiException if fails to make API call
*/
public VolumeEx getVolume(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getVolume");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get the volume expansion progress
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @return VolumeActionProgressResponse
* @throws ApiException if fails to make API call
*/
public VolumeActionProgressResponse getVolumeExpansionProgress(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling getVolumeExpansionProgress");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getVolumeExpansionProgress");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes/{id}/expand".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Initialize a ThinVolume
* Mode: Both Embedded and Proxy. Reinitialize the target thin volume, wiping out all existing data!
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param thinVolumeId (required)
* @return ThinVolumeEx
* @throws ApiException if fails to make API call
*/
public ThinVolumeEx initializeThinVolume(String systemId, String thinVolumeId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling initializeThinVolume");
}
// verify the required parameter 'thinVolumeId' is set
if (thinVolumeId == null) {
throw new ApiException(400, "Missing the required parameter 'thinVolumeId' when calling initializeThinVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/thin-volumes/{thinVolumeId}/initialize".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "thinVolumeId" + "\\}", apiClient.escapeString(thinVolumeId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Initialize a volume
* Mode: Both Embedded and Proxy. This procedure causes the specified volume to be re-initialized. All data that is currently present on the volume will be irretrievably lost as a result of this operation. Once a format operation starts, the volume's action field will be changed to 'initializing'; the getVolumeActionProgress procedure can then be used to monitor the progress of the operation. Volume format operations are typically required only when reviving a volume that has been marked failed for some reason. Newly-created volumes need not be explicitly formatted using this procedure.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param volumeId (required)
* @return VolumeEx
* @throws ApiException if fails to make API call
*/
public VolumeEx initializeVolume(String systemId, String volumeId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling initializeVolume");
}
// verify the required parameter 'volumeId' is set
if (volumeId == null) {
throw new ApiException(400, "Missing the required parameter 'volumeId' when calling initializeVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes/{volumeId}/initialize".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "volumeId" + "\\}", apiClient.escapeString(volumeId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Move a LunMapping to a different host or host group
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param mappingId (required)
* @param body (optional)
* @return LUNMapping
* @throws ApiException if fails to make API call
*/
public LUNMapping moveLunMapping(String systemId, String mappingId, VolumeMappingMoveRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling moveLunMapping");
}
// verify the required parameter 'mappingId' is set
if (mappingId == null) {
throw new ApiException(400, "Missing the required parameter 'mappingId' when calling moveLunMapping");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volume-mappings/{mappingId}/move".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "mappingId" + "\\}", apiClient.escapeString(mappingId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new LunMapping
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (optional)
* @return LUNMapping
* @throws ApiException if fails to make API call
*/
public LUNMapping newLunMapping(String systemId, VolumeMappingCreateRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling newLunMapping");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volume-mappings".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a storage pool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (optional)
* @return VolumeGroupEx
* @throws ApiException if fails to make API call
*/
public VolumeGroupEx newStoragePool(String systemId, StoragePoolCreateRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling newStoragePool");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a ThinVolume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (optional)
* @return ThinVolumeEx
* @throws ApiException if fails to make API call
*/
public ThinVolumeEx newThinVolume(String systemId, ThinVolumeCreateRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling newThinVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/thin-volumes".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new volume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param body (optional)
* @return VolumeEx
* @throws ApiException if fails to make API call
*/
public VolumeEx newVolume(String systemId, VolumeCreateRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling newVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Remove a LunMapping
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @throws ApiException if fails to make API call
*/
public void removeLunMapping(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling removeLunMapping");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling removeLunMapping");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volume-mappings/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete a storage pool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @param deleteVolumes Automatically delete all owned volumes when a delete request is received. (optional, default to false)
* @throws ApiException if fails to make API call
*/
public void removeStoragePool(String systemId, String id, Boolean deleteVolumes) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling removeStoragePool");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling removeStoragePool");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "delete-volumes", deleteVolumes));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Reduce the number of drives of a StoragePool
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @param body (optional)
* @return VolumeGroupEx
* @throws ApiException if fails to make API call
*/
public VolumeGroupEx removeStoragePoolDrive(String systemId, String id, DiskPoolReductionRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling removeStoragePoolDrive");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling removeStoragePoolDrive");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/storage-pools/{id}/reduction".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete a ThinVolume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @throws ApiException if fails to make API call
*/
public void removeThinVolume(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling removeThinVolume");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling removeThinVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/thin-volumes/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete a volume
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @throws ApiException if fails to make API call
*/
public void removeVolume(String systemId, String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling removeVolume");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling removeVolume");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes/{id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "basicAuth" };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Start the volume expansion
* Mode: Both Embedded and Proxy.
* @param systemId The unique identifier of the storage-system. This may be the id or the WWN. (required)
* @param id (required)
* @param body (optional)
* @return VolumeActionProgressResponse
* @throws ApiException if fails to make API call
*/
public VolumeActionProgressResponse startVolumeExpansion(String systemId, String id, VolumeExpansionRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'systemId' is set
if (systemId == null) {
throw new ApiException(400, "Missing the required parameter 'systemId' when calling startVolumeExpansion");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling startVolumeExpansion");
}
// create path and map variables
String localVarPath = "/storage-systems/{system-id}/volumes/{id}/expand".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "system-id" + "\\}", apiClient.escapeString(systemId.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map