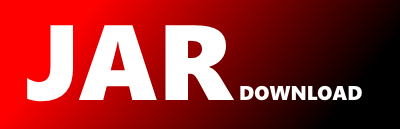
com.netapp.santricity.models.v2.HardwareInventoryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of santricity-java-client Show documentation
Show all versions of santricity-java-client Show documentation
The NetApp SANtricity WebAPI - Java SDK client library is a open source SDK that facilitate access to the
NetApp E-Series storage system for automation and integration into third-party web or script-based management tools.
The newest version!
/**************************************************************************************************************************************************************
* The Clear BSD License
*
* Copyright (c) – 2016, NetApp, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted (subject to the limitations in the disclaimer below) provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
*
* * Neither the name of NetApp, Inc. nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
*
* NO EXPRESS OR IMPLIED LICENSES TO ANY PARTY'S PATENT RIGHTS ARE GRANTED BY THIS LICENSE. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*************************************************************************************************************************************************************/
package com.netapp.santricity.models.v2;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Objects;
import com.netapp.santricity.models.v2.*;
import com.netapp.santricity.models.symbol.*;
import com.netapp.santricity.models.utils.*;
import com.netapp.santricity.Pair;
import com.netapp.santricity.StringUtil;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.io.File;
import java.util.Date;
import java.util.Objects;
/**
* Provides summary information for the physical components of the storage system.
*/
@ApiModel(description = "Provides summary information for the physical components of the storage system.")
@javax.annotation.Generated(value = "class com.ni.aa.client.codegen.lang.JavaNetappClientCodegen", date = "2017-10-04T15:05:52.333-05:00")
public class HardwareInventoryResponse {
private List drives;
private List ibPorts;
private List iscsiPorts;
private List fibrePorts;
private List sasPorts;
private List sasExpanders;
private List channelPorts;
private List trays;
private List drawers;
private List controllers;
private List batteries;
private List fans;
private List hostBoards;
private List powerSupplies;
private String nvsramVersion;
private List cacheMemoryDimms;
private List cacheBackupDevices;
private List supportCRUs;
private List esms;
private List sfps;
private List thermalSensors;
/**
* A list of the disk drives in the storage system.
**/
public HardwareInventoryResponse drives(List drives) {
this.drives = drives;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the disk drives in the storage system.")
@JsonProperty("drives")
public List getDrives() {
return drives;
}
public void setDrives(List drives) {
this.drives = drives;
}
/**
* IB Ports
**/
public HardwareInventoryResponse ibPorts(List ibPorts) {
this.ibPorts = ibPorts;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "IB Ports")
@JsonProperty("ibPorts")
public List getIbPorts() {
return ibPorts;
}
public void setIbPorts(List ibPorts) {
this.ibPorts = ibPorts;
}
/**
* ISCSI Port
**/
public HardwareInventoryResponse iscsiPorts(List iscsiPorts) {
this.iscsiPorts = iscsiPorts;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "ISCSI Port")
@JsonProperty("iscsiPorts")
public List getIscsiPorts() {
return iscsiPorts;
}
public void setIscsiPorts(List iscsiPorts) {
this.iscsiPorts = iscsiPorts;
}
/**
* Fibre Ports
**/
public HardwareInventoryResponse fibrePorts(List fibrePorts) {
this.fibrePorts = fibrePorts;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "Fibre Ports")
@JsonProperty("fibrePorts")
public List getFibrePorts() {
return fibrePorts;
}
public void setFibrePorts(List fibrePorts) {
this.fibrePorts = fibrePorts;
}
/**
* SAS Ports
**/
public HardwareInventoryResponse sasPorts(List sasPorts) {
this.sasPorts = sasPorts;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "SAS Ports")
@JsonProperty("sasPorts")
public List getSasPorts() {
return sasPorts;
}
public void setSasPorts(List sasPorts) {
this.sasPorts = sasPorts;
}
/**
* SAS Expanders
**/
public HardwareInventoryResponse sasExpanders(List sasExpanders) {
this.sasExpanders = sasExpanders;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "SAS Expanders")
@JsonProperty("sasExpanders")
public List getSasExpanders() {
return sasExpanders;
}
public void setSasExpanders(List sasExpanders) {
this.sasExpanders = sasExpanders;
}
/**
* Channel Ports
**/
public HardwareInventoryResponse channelPorts(List channelPorts) {
this.channelPorts = channelPorts;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "Channel Ports")
@JsonProperty("channelPorts")
public List getChannelPorts() {
return channelPorts;
}
public void setChannelPorts(List channelPorts) {
this.channelPorts = channelPorts;
}
/**
* A list of the disk trays in the storage system.
**/
public HardwareInventoryResponse trays(List trays) {
this.trays = trays;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the disk trays in the storage system.")
@JsonProperty("trays")
public List getTrays() {
return trays;
}
public void setTrays(List trays) {
this.trays = trays;
}
/**
* A list of the disk drawers
**/
public HardwareInventoryResponse drawers(List drawers) {
this.drawers = drawers;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the disk drawers")
@JsonProperty("drawers")
public List getDrawers() {
return drawers;
}
public void setDrawers(List drawers) {
this.drawers = drawers;
}
/**
* A list of the controllers in the storage system.
**/
public HardwareInventoryResponse controllers(List controllers) {
this.controllers = controllers;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the controllers in the storage system.")
@JsonProperty("controllers")
public List getControllers() {
return controllers;
}
public void setControllers(List controllers) {
this.controllers = controllers;
}
/**
* A list of the batteries in the storage system.
**/
public HardwareInventoryResponse batteries(List batteries) {
this.batteries = batteries;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the batteries in the storage system.")
@JsonProperty("batteries")
public List getBatteries() {
return batteries;
}
public void setBatteries(List batteries) {
this.batteries = batteries;
}
/**
* A list of the fans in the storage system.
**/
public HardwareInventoryResponse fans(List fans) {
this.fans = fans;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the fans in the storage system.")
@JsonProperty("fans")
public List getFans() {
return fans;
}
public void setFans(List fans) {
this.fans = fans;
}
/**
* A list of the host interface cards in the storage system.
**/
public HardwareInventoryResponse hostBoards(List hostBoards) {
this.hostBoards = hostBoards;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the host interface cards in the storage system.")
@JsonProperty("hostBoards")
public List getHostBoards() {
return hostBoards;
}
public void setHostBoards(List hostBoards) {
this.hostBoards = hostBoards;
}
/**
* A list of the power supplies in the storage system.
**/
public HardwareInventoryResponse powerSupplies(List powerSupplies) {
this.powerSupplies = powerSupplies;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the power supplies in the storage system.")
@JsonProperty("powerSupplies")
public List getPowerSupplies() {
return powerSupplies;
}
public void setPowerSupplies(List powerSupplies) {
this.powerSupplies = powerSupplies;
}
/**
* The version of NVSRAM settings installed on the storage system.
**/
public HardwareInventoryResponse nvsramVersion(String nvsramVersion) {
this.nvsramVersion = nvsramVersion;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "The version of NVSRAM settings installed on the storage system.")
@JsonProperty("nvsramVersion")
public String getNvsramVersion() {
return nvsramVersion;
}
public void setNvsramVersion(String nvsramVersion) {
this.nvsramVersion = nvsramVersion;
}
/**
* The list of processor memory DIMMs installed on the storage system.
**/
public HardwareInventoryResponse cacheMemoryDimms(List cacheMemoryDimms) {
this.cacheMemoryDimms = cacheMemoryDimms;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "The list of processor memory DIMMs installed on the storage system.")
@JsonProperty("cacheMemoryDimms")
public List getCacheMemoryDimms() {
return cacheMemoryDimms;
}
public void setCacheMemoryDimms(List cacheMemoryDimms) {
this.cacheMemoryDimms = cacheMemoryDimms;
}
/**
* The list of cache backup modules installed on the storage system.
**/
public HardwareInventoryResponse cacheBackupDevices(List cacheBackupDevices) {
this.cacheBackupDevices = cacheBackupDevices;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "The list of cache backup modules installed on the storage system.")
@JsonProperty("cacheBackupDevices")
public List getCacheBackupDevices() {
return cacheBackupDevices;
}
public void setCacheBackupDevices(List cacheBackupDevices) {
this.cacheBackupDevices = cacheBackupDevices;
}
/**
* A list of the support CRUs in the storage system.
**/
public HardwareInventoryResponse supportCRUs(List supportCRUs) {
this.supportCRUs = supportCRUs;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the support CRUs in the storage system.")
@JsonProperty("supportCRUs")
public List getSupportCRUs() {
return supportCRUs;
}
public void setSupportCRUs(List supportCRUs) {
this.supportCRUs = supportCRUs;
}
/**
* A list of the ESMs in the storage system.
**/
public HardwareInventoryResponse esms(List esms) {
this.esms = esms;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the ESMs in the storage system.")
@JsonProperty("esms")
public List getEsms() {
return esms;
}
public void setEsms(List esms) {
this.esms = esms;
}
/**
* A list of the SFPs in the storage system.
**/
public HardwareInventoryResponse sfps(List sfps) {
this.sfps = sfps;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the SFPs in the storage system.")
@JsonProperty("sfps")
public List getSfps() {
return sfps;
}
public void setSfps(List sfps) {
this.sfps = sfps;
}
/**
* A list of the thermal sensors in the storage system.
**/
public HardwareInventoryResponse thermalSensors(List thermalSensors) {
this.thermalSensors = thermalSensors;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "A list of the thermal sensors in the storage system.")
@JsonProperty("thermalSensors")
public List getThermalSensors() {
return thermalSensors;
}
public void setThermalSensors(List thermalSensors) {
this.thermalSensors = thermalSensors;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HardwareInventoryResponse hardwareInventoryResponse = (HardwareInventoryResponse) o;
return Objects.equals(this.drives, hardwareInventoryResponse.drives) &&
Objects.equals(this.ibPorts, hardwareInventoryResponse.ibPorts) &&
Objects.equals(this.iscsiPorts, hardwareInventoryResponse.iscsiPorts) &&
Objects.equals(this.fibrePorts, hardwareInventoryResponse.fibrePorts) &&
Objects.equals(this.sasPorts, hardwareInventoryResponse.sasPorts) &&
Objects.equals(this.sasExpanders, hardwareInventoryResponse.sasExpanders) &&
Objects.equals(this.channelPorts, hardwareInventoryResponse.channelPorts) &&
Objects.equals(this.trays, hardwareInventoryResponse.trays) &&
Objects.equals(this.drawers, hardwareInventoryResponse.drawers) &&
Objects.equals(this.controllers, hardwareInventoryResponse.controllers) &&
Objects.equals(this.batteries, hardwareInventoryResponse.batteries) &&
Objects.equals(this.fans, hardwareInventoryResponse.fans) &&
Objects.equals(this.hostBoards, hardwareInventoryResponse.hostBoards) &&
Objects.equals(this.powerSupplies, hardwareInventoryResponse.powerSupplies) &&
Objects.equals(this.nvsramVersion, hardwareInventoryResponse.nvsramVersion) &&
Objects.equals(this.cacheMemoryDimms, hardwareInventoryResponse.cacheMemoryDimms) &&
Objects.equals(this.cacheBackupDevices, hardwareInventoryResponse.cacheBackupDevices) &&
Objects.equals(this.supportCRUs, hardwareInventoryResponse.supportCRUs) &&
Objects.equals(this.esms, hardwareInventoryResponse.esms) &&
Objects.equals(this.sfps, hardwareInventoryResponse.sfps) &&
Objects.equals(this.thermalSensors, hardwareInventoryResponse.thermalSensors);
}
@Override
public int hashCode() {
return Objects.hash(drives, ibPorts, iscsiPorts, fibrePorts, sasPorts, sasExpanders, channelPorts, trays, drawers, controllers, batteries, fans, hostBoards, powerSupplies, nvsramVersion, cacheMemoryDimms, cacheBackupDevices, supportCRUs, esms, sfps, thermalSensors);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HardwareInventoryResponse {\n");
sb.append(" drives: ").append(toIndentedString(drives)).append("\n");
sb.append(" ibPorts: ").append(toIndentedString(ibPorts)).append("\n");
sb.append(" iscsiPorts: ").append(toIndentedString(iscsiPorts)).append("\n");
sb.append(" fibrePorts: ").append(toIndentedString(fibrePorts)).append("\n");
sb.append(" sasPorts: ").append(toIndentedString(sasPorts)).append("\n");
sb.append(" sasExpanders: ").append(toIndentedString(sasExpanders)).append("\n");
sb.append(" channelPorts: ").append(toIndentedString(channelPorts)).append("\n");
sb.append(" trays: ").append(toIndentedString(trays)).append("\n");
sb.append(" drawers: ").append(toIndentedString(drawers)).append("\n");
sb.append(" controllers: ").append(toIndentedString(controllers)).append("\n");
sb.append(" batteries: ").append(toIndentedString(batteries)).append("\n");
sb.append(" fans: ").append(toIndentedString(fans)).append("\n");
sb.append(" hostBoards: ").append(toIndentedString(hostBoards)).append("\n");
sb.append(" powerSupplies: ").append(toIndentedString(powerSupplies)).append("\n");
sb.append(" nvsramVersion: ").append(toIndentedString(nvsramVersion)).append("\n");
sb.append(" cacheMemoryDimms: ").append(toIndentedString(cacheMemoryDimms)).append("\n");
sb.append(" cacheBackupDevices: ").append(toIndentedString(cacheBackupDevices)).append("\n");
sb.append(" supportCRUs: ").append(toIndentedString(supportCRUs)).append("\n");
sb.append(" esms: ").append(toIndentedString(esms)).append("\n");
sb.append(" sfps: ").append(toIndentedString(sfps)).append("\n");
sb.append(" thermalSensors: ").append(toIndentedString(thermalSensors)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy