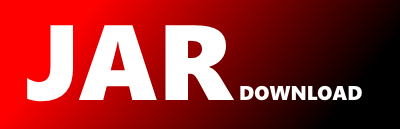
com.netease.arctic.ams.api.OptimizeManager Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Thrift Compiler (0.13.0)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.netease.arctic.ams.api;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.13.0)", date = "2023-02-24")
public class OptimizeManager {
/**
* replace TableContainer
*
*/
public interface Iface {
public void ping() throws com.netease.arctic.shade.org.apache.thrift.TException;
public OptimizeTask pollTask(int queueId, JobId jobId, java.lang.String attemptId, long waitTime) throws com.netease.arctic.ams.api.NoSuchObjectException, com.netease.arctic.shade.org.apache.thrift.TException;
public void reportOptimizeResult(OptimizeTaskStat optimizeTaskStat) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void reportOptimizerState(OptimizerStateReport reportData) throws com.netease.arctic.shade.org.apache.thrift.TException;
public OptimizerDescriptor registerOptimizer(OptimizerRegisterInfo registerInfo) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void stopOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier) throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException;
public void startOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier) throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException;
}
public interface AsyncIface {
public void ping(com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void pollTask(int queueId, JobId jobId, java.lang.String attemptId, long waitTime, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void reportOptimizeResult(OptimizeTaskStat optimizeTaskStat, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void reportOptimizerState(OptimizerStateReport reportData, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void registerOptimizer(OptimizerRegisterInfo registerInfo, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void stopOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
public void startOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException;
}
public static class Client extends com.netease.arctic.shade.org.apache.thrift.TServiceClient implements Iface {
public static class Factory implements com.netease.arctic.shade.org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public void ping() throws com.netease.arctic.shade.org.apache.thrift.TException
{
send_ping();
recv_ping();
}
public void send_ping() throws com.netease.arctic.shade.org.apache.thrift.TException
{
ping_args args = new ping_args();
sendBase("ping", args);
}
public void recv_ping() throws com.netease.arctic.shade.org.apache.thrift.TException
{
ping_result result = new ping_result();
receiveBase(result, "ping");
return;
}
public OptimizeTask pollTask(int queueId, JobId jobId, java.lang.String attemptId, long waitTime) throws com.netease.arctic.ams.api.NoSuchObjectException, com.netease.arctic.shade.org.apache.thrift.TException
{
send_pollTask(queueId, jobId, attemptId, waitTime);
return recv_pollTask();
}
public void send_pollTask(int queueId, JobId jobId, java.lang.String attemptId, long waitTime) throws com.netease.arctic.shade.org.apache.thrift.TException
{
pollTask_args args = new pollTask_args();
args.setQueueId(queueId);
args.setJobId(jobId);
args.setAttemptId(attemptId);
args.setWaitTime(waitTime);
sendBase("pollTask", args);
}
public OptimizeTask recv_pollTask() throws com.netease.arctic.ams.api.NoSuchObjectException, com.netease.arctic.shade.org.apache.thrift.TException
{
pollTask_result result = new pollTask_result();
receiveBase(result, "pollTask");
if (result.isSetSuccess()) {
return result.success;
}
if (result.e1 != null) {
throw result.e1;
}
throw new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.MISSING_RESULT, "pollTask failed: unknown result");
}
public void reportOptimizeResult(OptimizeTaskStat optimizeTaskStat) throws com.netease.arctic.shade.org.apache.thrift.TException
{
send_reportOptimizeResult(optimizeTaskStat);
recv_reportOptimizeResult();
}
public void send_reportOptimizeResult(OptimizeTaskStat optimizeTaskStat) throws com.netease.arctic.shade.org.apache.thrift.TException
{
reportOptimizeResult_args args = new reportOptimizeResult_args();
args.setOptimizeTaskStat(optimizeTaskStat);
sendBase("reportOptimizeResult", args);
}
public void recv_reportOptimizeResult() throws com.netease.arctic.shade.org.apache.thrift.TException
{
reportOptimizeResult_result result = new reportOptimizeResult_result();
receiveBase(result, "reportOptimizeResult");
return;
}
public void reportOptimizerState(OptimizerStateReport reportData) throws com.netease.arctic.shade.org.apache.thrift.TException
{
send_reportOptimizerState(reportData);
recv_reportOptimizerState();
}
public void send_reportOptimizerState(OptimizerStateReport reportData) throws com.netease.arctic.shade.org.apache.thrift.TException
{
reportOptimizerState_args args = new reportOptimizerState_args();
args.setReportData(reportData);
sendBase("reportOptimizerState", args);
}
public void recv_reportOptimizerState() throws com.netease.arctic.shade.org.apache.thrift.TException
{
reportOptimizerState_result result = new reportOptimizerState_result();
receiveBase(result, "reportOptimizerState");
return;
}
public OptimizerDescriptor registerOptimizer(OptimizerRegisterInfo registerInfo) throws com.netease.arctic.shade.org.apache.thrift.TException
{
send_registerOptimizer(registerInfo);
return recv_registerOptimizer();
}
public void send_registerOptimizer(OptimizerRegisterInfo registerInfo) throws com.netease.arctic.shade.org.apache.thrift.TException
{
registerOptimizer_args args = new registerOptimizer_args();
args.setRegisterInfo(registerInfo);
sendBase("registerOptimizer", args);
}
public OptimizerDescriptor recv_registerOptimizer() throws com.netease.arctic.shade.org.apache.thrift.TException
{
registerOptimizer_result result = new registerOptimizer_result();
receiveBase(result, "registerOptimizer");
if (result.isSetSuccess()) {
return result.success;
}
throw new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.MISSING_RESULT, "registerOptimizer failed: unknown result");
}
public void stopOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier) throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException
{
send_stopOptimize(tableIdentifier);
recv_stopOptimize();
}
public void send_stopOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier) throws com.netease.arctic.shade.org.apache.thrift.TException
{
stopOptimize_args args = new stopOptimize_args();
args.setTableIdentifier(tableIdentifier);
sendBase("stopOptimize", args);
}
public void recv_stopOptimize() throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException
{
stopOptimize_result result = new stopOptimize_result();
receiveBase(result, "stopOptimize");
if (result.e != null) {
throw result.e;
}
return;
}
public void startOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier) throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException
{
send_startOptimize(tableIdentifier);
recv_startOptimize();
}
public void send_startOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier) throws com.netease.arctic.shade.org.apache.thrift.TException
{
startOptimize_args args = new startOptimize_args();
args.setTableIdentifier(tableIdentifier);
sendBase("startOptimize", args);
}
public void recv_startOptimize() throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException
{
startOptimize_result result = new startOptimize_result();
receiveBase(result, "startOptimize");
if (result.e != null) {
throw result.e;
}
return;
}
}
public static class AsyncClient extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient implements AsyncIface {
public static class Factory implements com.netease.arctic.shade.org.apache.thrift.async.TAsyncClientFactory {
private com.netease.arctic.shade.org.apache.thrift.async.TAsyncClientManager clientManager;
private com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(com.netease.arctic.shade.org.apache.thrift.async.TAsyncClientManager clientManager, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClientManager clientManager, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void ping(com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
ping_call method_call = new ping_call(resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class ping_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
public ping_call(com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("ping", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
ping_args args = new ping_args();
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
public void pollTask(int queueId, JobId jobId, java.lang.String attemptId, long waitTime, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
pollTask_call method_call = new pollTask_call(queueId, jobId, attemptId, waitTime, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class pollTask_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
private int queueId;
private JobId jobId;
private java.lang.String attemptId;
private long waitTime;
public pollTask_call(int queueId, JobId jobId, java.lang.String attemptId, long waitTime, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.queueId = queueId;
this.jobId = jobId;
this.attemptId = attemptId;
this.waitTime = waitTime;
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("pollTask", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
pollTask_args args = new pollTask_args();
args.setQueueId(queueId);
args.setJobId(jobId);
args.setAttemptId(attemptId);
args.setWaitTime(waitTime);
args.write(prot);
prot.writeMessageEnd();
}
public OptimizeTask getResult() throws com.netease.arctic.ams.api.NoSuchObjectException, com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_pollTask();
}
}
public void reportOptimizeResult(OptimizeTaskStat optimizeTaskStat, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
reportOptimizeResult_call method_call = new reportOptimizeResult_call(optimizeTaskStat, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class reportOptimizeResult_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
private OptimizeTaskStat optimizeTaskStat;
public reportOptimizeResult_call(OptimizeTaskStat optimizeTaskStat, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.optimizeTaskStat = optimizeTaskStat;
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("reportOptimizeResult", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
reportOptimizeResult_args args = new reportOptimizeResult_args();
args.setOptimizeTaskStat(optimizeTaskStat);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
public void reportOptimizerState(OptimizerStateReport reportData, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
reportOptimizerState_call method_call = new reportOptimizerState_call(reportData, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class reportOptimizerState_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
private OptimizerStateReport reportData;
public reportOptimizerState_call(OptimizerStateReport reportData, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.reportData = reportData;
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("reportOptimizerState", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
reportOptimizerState_args args = new reportOptimizerState_args();
args.setReportData(reportData);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
public void registerOptimizer(OptimizerRegisterInfo registerInfo, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
registerOptimizer_call method_call = new registerOptimizer_call(registerInfo, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class registerOptimizer_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
private OptimizerRegisterInfo registerInfo;
public registerOptimizer_call(OptimizerRegisterInfo registerInfo, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.registerInfo = registerInfo;
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("registerOptimizer", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
registerOptimizer_args args = new registerOptimizer_args();
args.setRegisterInfo(registerInfo);
args.write(prot);
prot.writeMessageEnd();
}
public OptimizerDescriptor getResult() throws com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_registerOptimizer();
}
}
public void stopOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
stopOptimize_call method_call = new stopOptimize_call(tableIdentifier, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class stopOptimize_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
private com.netease.arctic.ams.api.TableIdentifier tableIdentifier;
public stopOptimize_call(com.netease.arctic.ams.api.TableIdentifier tableIdentifier, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.tableIdentifier = tableIdentifier;
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("stopOptimize", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
stopOptimize_args args = new stopOptimize_args();
args.setTableIdentifier(tableIdentifier);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
public void startOptimize(com.netease.arctic.ams.api.TableIdentifier tableIdentifier, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
checkReady();
startOptimize_call method_call = new startOptimize_call(tableIdentifier, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class startOptimize_call extends com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall {
private com.netease.arctic.ams.api.TableIdentifier tableIdentifier;
public startOptimize_call(com.netease.arctic.ams.api.TableIdentifier tableIdentifier, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler, com.netease.arctic.shade.org.apache.thrift.async.TAsyncClient client, com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolFactory protocolFactory, com.netease.arctic.shade.org.apache.thrift.transport.TNonblockingTransport transport) throws com.netease.arctic.shade.org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.tableIdentifier = tableIdentifier;
}
public void write_args(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot) throws com.netease.arctic.shade.org.apache.thrift.TException {
prot.writeMessageBegin(new com.netease.arctic.shade.org.apache.thrift.protocol.TMessage("startOptimize", com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.CALL, 0));
startOptimize_args args = new startOptimize_args();
args.setTableIdentifier(tableIdentifier);
args.write(prot);
prot.writeMessageEnd();
}
public Void getResult() throws com.netease.arctic.ams.api.OperationErrorException, com.netease.arctic.shade.org.apache.thrift.TException {
if (getState() != com.netease.arctic.shade.org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new com.netease.arctic.shade.org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return null;
}
}
}
public static class Processor extends com.netease.arctic.shade.org.apache.thrift.TBaseProcessor implements com.netease.arctic.shade.org.apache.thrift.TProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected Processor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("ping", new ping());
processMap.put("pollTask", new pollTask());
processMap.put("reportOptimizeResult", new reportOptimizeResult());
processMap.put("reportOptimizerState", new reportOptimizerState());
processMap.put("registerOptimizer", new registerOptimizer());
processMap.put("stopOptimize", new stopOptimize());
processMap.put("startOptimize", new startOptimize());
return processMap;
}
public static class ping extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public ping() {
super("ping");
}
public ping_args getEmptyArgsInstance() {
return new ping_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public ping_result getResult(I iface, ping_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
ping_result result = new ping_result();
iface.ping();
return result;
}
}
public static class pollTask extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public pollTask() {
super("pollTask");
}
public pollTask_args getEmptyArgsInstance() {
return new pollTask_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public pollTask_result getResult(I iface, pollTask_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
pollTask_result result = new pollTask_result();
try {
result.success = iface.pollTask(args.queueId, args.jobId, args.attemptId, args.waitTime);
} catch (com.netease.arctic.ams.api.NoSuchObjectException e1) {
result.e1 = e1;
}
return result;
}
}
public static class reportOptimizeResult extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public reportOptimizeResult() {
super("reportOptimizeResult");
}
public reportOptimizeResult_args getEmptyArgsInstance() {
return new reportOptimizeResult_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public reportOptimizeResult_result getResult(I iface, reportOptimizeResult_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
reportOptimizeResult_result result = new reportOptimizeResult_result();
iface.reportOptimizeResult(args.optimizeTaskStat);
return result;
}
}
public static class reportOptimizerState extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public reportOptimizerState() {
super("reportOptimizerState");
}
public reportOptimizerState_args getEmptyArgsInstance() {
return new reportOptimizerState_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public reportOptimizerState_result getResult(I iface, reportOptimizerState_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
reportOptimizerState_result result = new reportOptimizerState_result();
iface.reportOptimizerState(args.reportData);
return result;
}
}
public static class registerOptimizer extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public registerOptimizer() {
super("registerOptimizer");
}
public registerOptimizer_args getEmptyArgsInstance() {
return new registerOptimizer_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public registerOptimizer_result getResult(I iface, registerOptimizer_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
registerOptimizer_result result = new registerOptimizer_result();
result.success = iface.registerOptimizer(args.registerInfo);
return result;
}
}
public static class stopOptimize extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public stopOptimize() {
super("stopOptimize");
}
public stopOptimize_args getEmptyArgsInstance() {
return new stopOptimize_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public stopOptimize_result getResult(I iface, stopOptimize_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
stopOptimize_result result = new stopOptimize_result();
try {
iface.stopOptimize(args.tableIdentifier);
} catch (com.netease.arctic.ams.api.OperationErrorException e) {
result.e = e;
}
return result;
}
}
public static class startOptimize extends com.netease.arctic.shade.org.apache.thrift.ProcessFunction {
public startOptimize() {
super("startOptimize");
}
public startOptimize_args getEmptyArgsInstance() {
return new startOptimize_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public startOptimize_result getResult(I iface, startOptimize_args args) throws com.netease.arctic.shade.org.apache.thrift.TException {
startOptimize_result result = new startOptimize_result();
try {
iface.startOptimize(args.tableIdentifier);
} catch (com.netease.arctic.ams.api.OperationErrorException e) {
result.e = e;
}
return result;
}
}
}
public static class AsyncProcessor extends com.netease.arctic.shade.org.apache.thrift.TBaseAsyncProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(AsyncProcessor.class.getName());
public AsyncProcessor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected AsyncProcessor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("ping", new ping());
processMap.put("pollTask", new pollTask());
processMap.put("reportOptimizeResult", new reportOptimizeResult());
processMap.put("reportOptimizerState", new reportOptimizerState());
processMap.put("registerOptimizer", new registerOptimizer());
processMap.put("stopOptimize", new stopOptimize());
processMap.put("startOptimize", new startOptimize());
return processMap;
}
public static class ping extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public ping() {
super("ping");
}
public ping_args getEmptyArgsInstance() {
return new ping_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
ping_result result = new ping_result();
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
ping_result result = new ping_result();
if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, ping_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.ping(resultHandler);
}
}
public static class pollTask extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public pollTask() {
super("pollTask");
}
public pollTask_args getEmptyArgsInstance() {
return new pollTask_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(OptimizeTask o) {
pollTask_result result = new pollTask_result();
result.success = o;
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
pollTask_result result = new pollTask_result();
if (e instanceof com.netease.arctic.ams.api.NoSuchObjectException) {
result.e1 = (com.netease.arctic.ams.api.NoSuchObjectException) e;
result.setE1IsSet(true);
msg = result;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, pollTask_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.pollTask(args.queueId, args.jobId, args.attemptId, args.waitTime,resultHandler);
}
}
public static class reportOptimizeResult extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public reportOptimizeResult() {
super("reportOptimizeResult");
}
public reportOptimizeResult_args getEmptyArgsInstance() {
return new reportOptimizeResult_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
reportOptimizeResult_result result = new reportOptimizeResult_result();
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
reportOptimizeResult_result result = new reportOptimizeResult_result();
if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, reportOptimizeResult_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.reportOptimizeResult(args.optimizeTaskStat,resultHandler);
}
}
public static class reportOptimizerState extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public reportOptimizerState() {
super("reportOptimizerState");
}
public reportOptimizerState_args getEmptyArgsInstance() {
return new reportOptimizerState_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
reportOptimizerState_result result = new reportOptimizerState_result();
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
reportOptimizerState_result result = new reportOptimizerState_result();
if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, reportOptimizerState_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.reportOptimizerState(args.reportData,resultHandler);
}
}
public static class registerOptimizer extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public registerOptimizer() {
super("registerOptimizer");
}
public registerOptimizer_args getEmptyArgsInstance() {
return new registerOptimizer_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(OptimizerDescriptor o) {
registerOptimizer_result result = new registerOptimizer_result();
result.success = o;
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
registerOptimizer_result result = new registerOptimizer_result();
if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, registerOptimizer_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.registerOptimizer(args.registerInfo,resultHandler);
}
}
public static class stopOptimize extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public stopOptimize() {
super("stopOptimize");
}
public stopOptimize_args getEmptyArgsInstance() {
return new stopOptimize_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
stopOptimize_result result = new stopOptimize_result();
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
stopOptimize_result result = new stopOptimize_result();
if (e instanceof com.netease.arctic.ams.api.OperationErrorException) {
result.e = (com.netease.arctic.ams.api.OperationErrorException) e;
result.setEIsSet(true);
msg = result;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, stopOptimize_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.stopOptimize(args.tableIdentifier,resultHandler);
}
}
public static class startOptimize extends com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction {
public startOptimize() {
super("startOptimize");
}
public startOptimize_args getEmptyArgsInstance() {
return new startOptimize_args();
}
public com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback getResultHandler(final com.netease.arctic.shade.org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final com.netease.arctic.shade.org.apache.thrift.AsyncProcessFunction fcall = this;
return new com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(Void o) {
startOptimize_result result = new startOptimize_result();
try {
fcall.sendResponse(fb, result, com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (com.netease.arctic.shade.org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.REPLY;
com.netease.arctic.shade.org.apache.thrift.TSerializable msg;
startOptimize_result result = new startOptimize_result();
if (e instanceof com.netease.arctic.ams.api.OperationErrorException) {
result.e = (com.netease.arctic.ams.api.OperationErrorException) e;
result.setEIsSet(true);
msg = result;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof com.netease.arctic.shade.org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (com.netease.arctic.shade.org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = com.netease.arctic.shade.org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new com.netease.arctic.shade.org.apache.thrift.TApplicationException(com.netease.arctic.shade.org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, startOptimize_args args, com.netease.arctic.shade.org.apache.thrift.async.AsyncMethodCallback resultHandler) throws com.netease.arctic.shade.org.apache.thrift.TException {
iface.startOptimize(args.tableIdentifier,resultHandler);
}
}
}
public static class ping_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("ping_args");
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new ping_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new ping_argsTupleSchemeFactory();
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
;
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ping_args.class, metaDataMap);
}
public ping_args() {
}
/**
* Performs a deep copy on other.
*/
public ping_args(ping_args other) {
}
public ping_args deepCopy() {
return new ping_args(this);
}
@Override
public void clear() {
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof ping_args)
return this.equals((ping_args)that);
return false;
}
public boolean equals(ping_args that) {
if (that == null)
return false;
if (this == that)
return true;
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
return hashCode;
}
@Override
public int compareTo(ping_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("ping_args(");
boolean first = true;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class ping_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public ping_argsStandardScheme getScheme() {
return new ping_argsStandardScheme();
}
}
private static class ping_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, ping_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, ping_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class ping_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public ping_argsTupleScheme getScheme() {
return new ping_argsTupleScheme();
}
}
private static class ping_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, ping_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, ping_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class ping_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("ping_result");
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new ping_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new ping_resultTupleSchemeFactory();
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
;
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ping_result.class, metaDataMap);
}
public ping_result() {
}
/**
* Performs a deep copy on other.
*/
public ping_result(ping_result other) {
}
public ping_result deepCopy() {
return new ping_result(this);
}
@Override
public void clear() {
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof ping_result)
return this.equals((ping_result)that);
return false;
}
public boolean equals(ping_result that) {
if (that == null)
return false;
if (this == that)
return true;
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
return hashCode;
}
@Override
public int compareTo(ping_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("ping_result(");
boolean first = true;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class ping_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public ping_resultStandardScheme getScheme() {
return new ping_resultStandardScheme();
}
}
private static class ping_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, ping_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, ping_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class ping_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public ping_resultTupleScheme getScheme() {
return new ping_resultTupleScheme();
}
}
private static class ping_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, ping_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, ping_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class pollTask_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("pollTask_args");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField QUEUE_ID_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("queueId", com.netease.arctic.shade.org.apache.thrift.protocol.TType.I32, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField JOB_ID_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("jobId", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)2);
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField ATTEMPT_ID_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("attemptId", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRING, (short)3);
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField WAIT_TIME_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("waitTime", com.netease.arctic.shade.org.apache.thrift.protocol.TType.I64, (short)4);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new pollTask_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new pollTask_argsTupleSchemeFactory();
public int queueId; // required
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable JobId jobId; // required
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.String attemptId; // required
public long waitTime; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
QUEUE_ID((short)1, "queueId"),
JOB_ID((short)2, "jobId"),
ATTEMPT_ID((short)3, "attemptId"),
WAIT_TIME((short)4, "waitTime");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // QUEUE_ID
return QUEUE_ID;
case 2: // JOB_ID
return JOB_ID;
case 3: // ATTEMPT_ID
return ATTEMPT_ID;
case 4: // WAIT_TIME
return WAIT_TIME;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __QUEUEID_ISSET_ID = 0;
private static final int __WAITTIME_ISSET_ID = 1;
private byte __isset_bitfield = 0;
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.QUEUE_ID, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("queueId", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldValueMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.JOB_ID, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("jobId", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, JobId.class)));
tmpMap.put(_Fields.ATTEMPT_ID, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("attemptId", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldValueMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WAIT_TIME, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("waitTime", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldValueMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.I64)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(pollTask_args.class, metaDataMap);
}
public pollTask_args() {
}
public pollTask_args(
int queueId,
JobId jobId,
java.lang.String attemptId,
long waitTime)
{
this();
this.queueId = queueId;
setQueueIdIsSet(true);
this.jobId = jobId;
this.attemptId = attemptId;
this.waitTime = waitTime;
setWaitTimeIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public pollTask_args(pollTask_args other) {
__isset_bitfield = other.__isset_bitfield;
this.queueId = other.queueId;
if (other.isSetJobId()) {
this.jobId = new JobId(other.jobId);
}
if (other.isSetAttemptId()) {
this.attemptId = other.attemptId;
}
this.waitTime = other.waitTime;
}
public pollTask_args deepCopy() {
return new pollTask_args(this);
}
@Override
public void clear() {
setQueueIdIsSet(false);
this.queueId = 0;
this.jobId = null;
this.attemptId = null;
setWaitTimeIsSet(false);
this.waitTime = 0;
}
public int getQueueId() {
return this.queueId;
}
public pollTask_args setQueueId(int queueId) {
this.queueId = queueId;
setQueueIdIsSet(true);
return this;
}
public void unsetQueueId() {
__isset_bitfield = com.netease.arctic.shade.org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __QUEUEID_ISSET_ID);
}
/** Returns true if field queueId is set (has been assigned a value) and false otherwise */
public boolean isSetQueueId() {
return com.netease.arctic.shade.org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __QUEUEID_ISSET_ID);
}
public void setQueueIdIsSet(boolean value) {
__isset_bitfield = com.netease.arctic.shade.org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __QUEUEID_ISSET_ID, value);
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public JobId getJobId() {
return this.jobId;
}
public pollTask_args setJobId(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable JobId jobId) {
this.jobId = jobId;
return this;
}
public void unsetJobId() {
this.jobId = null;
}
/** Returns true if field jobId is set (has been assigned a value) and false otherwise */
public boolean isSetJobId() {
return this.jobId != null;
}
public void setJobIdIsSet(boolean value) {
if (!value) {
this.jobId = null;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.String getAttemptId() {
return this.attemptId;
}
public pollTask_args setAttemptId(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.String attemptId) {
this.attemptId = attemptId;
return this;
}
public void unsetAttemptId() {
this.attemptId = null;
}
/** Returns true if field attemptId is set (has been assigned a value) and false otherwise */
public boolean isSetAttemptId() {
return this.attemptId != null;
}
public void setAttemptIdIsSet(boolean value) {
if (!value) {
this.attemptId = null;
}
}
public long getWaitTime() {
return this.waitTime;
}
public pollTask_args setWaitTime(long waitTime) {
this.waitTime = waitTime;
setWaitTimeIsSet(true);
return this;
}
public void unsetWaitTime() {
__isset_bitfield = com.netease.arctic.shade.org.apache.thrift.EncodingUtils.clearBit(__isset_bitfield, __WAITTIME_ISSET_ID);
}
/** Returns true if field waitTime is set (has been assigned a value) and false otherwise */
public boolean isSetWaitTime() {
return com.netease.arctic.shade.org.apache.thrift.EncodingUtils.testBit(__isset_bitfield, __WAITTIME_ISSET_ID);
}
public void setWaitTimeIsSet(boolean value) {
__isset_bitfield = com.netease.arctic.shade.org.apache.thrift.EncodingUtils.setBit(__isset_bitfield, __WAITTIME_ISSET_ID, value);
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case QUEUE_ID:
if (value == null) {
unsetQueueId();
} else {
setQueueId((java.lang.Integer)value);
}
break;
case JOB_ID:
if (value == null) {
unsetJobId();
} else {
setJobId((JobId)value);
}
break;
case ATTEMPT_ID:
if (value == null) {
unsetAttemptId();
} else {
setAttemptId((java.lang.String)value);
}
break;
case WAIT_TIME:
if (value == null) {
unsetWaitTime();
} else {
setWaitTime((java.lang.Long)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case QUEUE_ID:
return getQueueId();
case JOB_ID:
return getJobId();
case ATTEMPT_ID:
return getAttemptId();
case WAIT_TIME:
return getWaitTime();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case QUEUE_ID:
return isSetQueueId();
case JOB_ID:
return isSetJobId();
case ATTEMPT_ID:
return isSetAttemptId();
case WAIT_TIME:
return isSetWaitTime();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof pollTask_args)
return this.equals((pollTask_args)that);
return false;
}
public boolean equals(pollTask_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_queueId = true;
boolean that_present_queueId = true;
if (this_present_queueId || that_present_queueId) {
if (!(this_present_queueId && that_present_queueId))
return false;
if (this.queueId != that.queueId)
return false;
}
boolean this_present_jobId = true && this.isSetJobId();
boolean that_present_jobId = true && that.isSetJobId();
if (this_present_jobId || that_present_jobId) {
if (!(this_present_jobId && that_present_jobId))
return false;
if (!this.jobId.equals(that.jobId))
return false;
}
boolean this_present_attemptId = true && this.isSetAttemptId();
boolean that_present_attemptId = true && that.isSetAttemptId();
if (this_present_attemptId || that_present_attemptId) {
if (!(this_present_attemptId && that_present_attemptId))
return false;
if (!this.attemptId.equals(that.attemptId))
return false;
}
boolean this_present_waitTime = true;
boolean that_present_waitTime = true;
if (this_present_waitTime || that_present_waitTime) {
if (!(this_present_waitTime && that_present_waitTime))
return false;
if (this.waitTime != that.waitTime)
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + queueId;
hashCode = hashCode * 8191 + ((isSetJobId()) ? 131071 : 524287);
if (isSetJobId())
hashCode = hashCode * 8191 + jobId.hashCode();
hashCode = hashCode * 8191 + ((isSetAttemptId()) ? 131071 : 524287);
if (isSetAttemptId())
hashCode = hashCode * 8191 + attemptId.hashCode();
hashCode = hashCode * 8191 + com.netease.arctic.shade.org.apache.thrift.TBaseHelper.hashCode(waitTime);
return hashCode;
}
@Override
public int compareTo(pollTask_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetQueueId()).compareTo(other.isSetQueueId());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetQueueId()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.queueId, other.queueId);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.valueOf(isSetJobId()).compareTo(other.isSetJobId());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetJobId()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.jobId, other.jobId);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.valueOf(isSetAttemptId()).compareTo(other.isSetAttemptId());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetAttemptId()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.attemptId, other.attemptId);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.valueOf(isSetWaitTime()).compareTo(other.isSetWaitTime());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetWaitTime()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.waitTime, other.waitTime);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("pollTask_args(");
boolean first = true;
sb.append("queueId:");
sb.append(this.queueId);
first = false;
if (!first) sb.append(", ");
sb.append("jobId:");
if (this.jobId == null) {
sb.append("null");
} else {
sb.append(this.jobId);
}
first = false;
if (!first) sb.append(", ");
sb.append("attemptId:");
if (this.attemptId == null) {
sb.append("null");
} else {
sb.append(this.attemptId);
}
first = false;
if (!first) sb.append(", ");
sb.append("waitTime:");
sb.append(this.waitTime);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (jobId != null) {
jobId.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
// it doesn't seem like you should have to do this, but java serialization is wacky, and doesn't call the default constructor.
__isset_bitfield = 0;
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class pollTask_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public pollTask_argsStandardScheme getScheme() {
return new pollTask_argsStandardScheme();
}
}
private static class pollTask_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, pollTask_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // QUEUE_ID
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.I32) {
struct.queueId = iprot.readI32();
struct.setQueueIdIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 2: // JOB_ID
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.jobId = new JobId();
struct.jobId.read(iprot);
struct.setJobIdIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 3: // ATTEMPT_ID
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRING) {
struct.attemptId = iprot.readString();
struct.setAttemptIdIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 4: // WAIT_TIME
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.I64) {
struct.waitTime = iprot.readI64();
struct.setWaitTimeIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, pollTask_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldBegin(QUEUE_ID_FIELD_DESC);
oprot.writeI32(struct.queueId);
oprot.writeFieldEnd();
if (struct.jobId != null) {
oprot.writeFieldBegin(JOB_ID_FIELD_DESC);
struct.jobId.write(oprot);
oprot.writeFieldEnd();
}
if (struct.attemptId != null) {
oprot.writeFieldBegin(ATTEMPT_ID_FIELD_DESC);
oprot.writeString(struct.attemptId);
oprot.writeFieldEnd();
}
oprot.writeFieldBegin(WAIT_TIME_FIELD_DESC);
oprot.writeI64(struct.waitTime);
oprot.writeFieldEnd();
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class pollTask_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public pollTask_argsTupleScheme getScheme() {
return new pollTask_argsTupleScheme();
}
}
private static class pollTask_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, pollTask_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetQueueId()) {
optionals.set(0);
}
if (struct.isSetJobId()) {
optionals.set(1);
}
if (struct.isSetAttemptId()) {
optionals.set(2);
}
if (struct.isSetWaitTime()) {
optionals.set(3);
}
oprot.writeBitSet(optionals, 4);
if (struct.isSetQueueId()) {
oprot.writeI32(struct.queueId);
}
if (struct.isSetJobId()) {
struct.jobId.write(oprot);
}
if (struct.isSetAttemptId()) {
oprot.writeString(struct.attemptId);
}
if (struct.isSetWaitTime()) {
oprot.writeI64(struct.waitTime);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, pollTask_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(4);
if (incoming.get(0)) {
struct.queueId = iprot.readI32();
struct.setQueueIdIsSet(true);
}
if (incoming.get(1)) {
struct.jobId = new JobId();
struct.jobId.read(iprot);
struct.setJobIdIsSet(true);
}
if (incoming.get(2)) {
struct.attemptId = iprot.readString();
struct.setAttemptIdIsSet(true);
}
if (incoming.get(3)) {
struct.waitTime = iprot.readI64();
struct.setWaitTimeIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class pollTask_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("pollTask_result");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("success", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField E1_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("e1", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new pollTask_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new pollTask_resultTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizeTask success; // required
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.NoSuchObjectException e1; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success"),
E1((short)1, "e1");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
case 1: // E1
return E1;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("success", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, OptimizeTask.class)));
tmpMap.put(_Fields.E1, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("e1", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, com.netease.arctic.ams.api.NoSuchObjectException.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(pollTask_result.class, metaDataMap);
}
public pollTask_result() {
}
public pollTask_result(
OptimizeTask success,
com.netease.arctic.ams.api.NoSuchObjectException e1)
{
this();
this.success = success;
this.e1 = e1;
}
/**
* Performs a deep copy on other.
*/
public pollTask_result(pollTask_result other) {
if (other.isSetSuccess()) {
this.success = new OptimizeTask(other.success);
}
if (other.isSetE1()) {
this.e1 = new com.netease.arctic.ams.api.NoSuchObjectException(other.e1);
}
}
public pollTask_result deepCopy() {
return new pollTask_result(this);
}
@Override
public void clear() {
this.success = null;
this.e1 = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public OptimizeTask getSuccess() {
return this.success;
}
public pollTask_result setSuccess(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizeTask success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public com.netease.arctic.ams.api.NoSuchObjectException getE1() {
return this.e1;
}
public pollTask_result setE1(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.NoSuchObjectException e1) {
this.e1 = e1;
return this;
}
public void unsetE1() {
this.e1 = null;
}
/** Returns true if field e1 is set (has been assigned a value) and false otherwise */
public boolean isSetE1() {
return this.e1 != null;
}
public void setE1IsSet(boolean value) {
if (!value) {
this.e1 = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((OptimizeTask)value);
}
break;
case E1:
if (value == null) {
unsetE1();
} else {
setE1((com.netease.arctic.ams.api.NoSuchObjectException)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
case E1:
return getE1();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
case E1:
return isSetE1();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof pollTask_result)
return this.equals((pollTask_result)that);
return false;
}
public boolean equals(pollTask_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
boolean this_present_e1 = true && this.isSetE1();
boolean that_present_e1 = true && that.isSetE1();
if (this_present_e1 || that_present_e1) {
if (!(this_present_e1 && that_present_e1))
return false;
if (!this.e1.equals(that.e1))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
hashCode = hashCode * 8191 + ((isSetE1()) ? 131071 : 524287);
if (isSetE1())
hashCode = hashCode * 8191 + e1.hashCode();
return hashCode;
}
@Override
public int compareTo(pollTask_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetSuccess()).compareTo(other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = java.lang.Boolean.valueOf(isSetE1()).compareTo(other.isSetE1());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetE1()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.e1, other.e1);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("pollTask_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
if (!first) sb.append(", ");
sb.append("e1:");
if (this.e1 == null) {
sb.append("null");
} else {
sb.append(this.e1);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class pollTask_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public pollTask_resultStandardScheme getScheme() {
return new pollTask_resultStandardScheme();
}
}
private static class pollTask_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, pollTask_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new OptimizeTask();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
case 1: // E1
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.e1 = new com.netease.arctic.ams.api.NoSuchObjectException();
struct.e1.read(iprot);
struct.setE1IsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, pollTask_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
if (struct.e1 != null) {
oprot.writeFieldBegin(E1_FIELD_DESC);
struct.e1.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class pollTask_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public pollTask_resultTupleScheme getScheme() {
return new pollTask_resultTupleScheme();
}
}
private static class pollTask_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, pollTask_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
if (struct.isSetE1()) {
optionals.set(1);
}
oprot.writeBitSet(optionals, 2);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
if (struct.isSetE1()) {
struct.e1.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, pollTask_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(2);
if (incoming.get(0)) {
struct.success = new OptimizeTask();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
if (incoming.get(1)) {
struct.e1 = new com.netease.arctic.ams.api.NoSuchObjectException();
struct.e1.read(iprot);
struct.setE1IsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class reportOptimizeResult_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("reportOptimizeResult_args");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField OPTIMIZE_TASK_STAT_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("optimizeTaskStat", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new reportOptimizeResult_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new reportOptimizeResult_argsTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizeTaskStat optimizeTaskStat; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
OPTIMIZE_TASK_STAT((short)1, "optimizeTaskStat");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // OPTIMIZE_TASK_STAT
return OPTIMIZE_TASK_STAT;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.OPTIMIZE_TASK_STAT, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("optimizeTaskStat", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, OptimizeTaskStat.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(reportOptimizeResult_args.class, metaDataMap);
}
public reportOptimizeResult_args() {
}
public reportOptimizeResult_args(
OptimizeTaskStat optimizeTaskStat)
{
this();
this.optimizeTaskStat = optimizeTaskStat;
}
/**
* Performs a deep copy on other.
*/
public reportOptimizeResult_args(reportOptimizeResult_args other) {
if (other.isSetOptimizeTaskStat()) {
this.optimizeTaskStat = new OptimizeTaskStat(other.optimizeTaskStat);
}
}
public reportOptimizeResult_args deepCopy() {
return new reportOptimizeResult_args(this);
}
@Override
public void clear() {
this.optimizeTaskStat = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public OptimizeTaskStat getOptimizeTaskStat() {
return this.optimizeTaskStat;
}
public reportOptimizeResult_args setOptimizeTaskStat(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizeTaskStat optimizeTaskStat) {
this.optimizeTaskStat = optimizeTaskStat;
return this;
}
public void unsetOptimizeTaskStat() {
this.optimizeTaskStat = null;
}
/** Returns true if field optimizeTaskStat is set (has been assigned a value) and false otherwise */
public boolean isSetOptimizeTaskStat() {
return this.optimizeTaskStat != null;
}
public void setOptimizeTaskStatIsSet(boolean value) {
if (!value) {
this.optimizeTaskStat = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case OPTIMIZE_TASK_STAT:
if (value == null) {
unsetOptimizeTaskStat();
} else {
setOptimizeTaskStat((OptimizeTaskStat)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case OPTIMIZE_TASK_STAT:
return getOptimizeTaskStat();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case OPTIMIZE_TASK_STAT:
return isSetOptimizeTaskStat();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof reportOptimizeResult_args)
return this.equals((reportOptimizeResult_args)that);
return false;
}
public boolean equals(reportOptimizeResult_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_optimizeTaskStat = true && this.isSetOptimizeTaskStat();
boolean that_present_optimizeTaskStat = true && that.isSetOptimizeTaskStat();
if (this_present_optimizeTaskStat || that_present_optimizeTaskStat) {
if (!(this_present_optimizeTaskStat && that_present_optimizeTaskStat))
return false;
if (!this.optimizeTaskStat.equals(that.optimizeTaskStat))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetOptimizeTaskStat()) ? 131071 : 524287);
if (isSetOptimizeTaskStat())
hashCode = hashCode * 8191 + optimizeTaskStat.hashCode();
return hashCode;
}
@Override
public int compareTo(reportOptimizeResult_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetOptimizeTaskStat()).compareTo(other.isSetOptimizeTaskStat());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetOptimizeTaskStat()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.optimizeTaskStat, other.optimizeTaskStat);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("reportOptimizeResult_args(");
boolean first = true;
sb.append("optimizeTaskStat:");
if (this.optimizeTaskStat == null) {
sb.append("null");
} else {
sb.append(this.optimizeTaskStat);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (optimizeTaskStat != null) {
optimizeTaskStat.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class reportOptimizeResult_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizeResult_argsStandardScheme getScheme() {
return new reportOptimizeResult_argsStandardScheme();
}
}
private static class reportOptimizeResult_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, reportOptimizeResult_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // OPTIMIZE_TASK_STAT
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.optimizeTaskStat = new OptimizeTaskStat();
struct.optimizeTaskStat.read(iprot);
struct.setOptimizeTaskStatIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, reportOptimizeResult_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.optimizeTaskStat != null) {
oprot.writeFieldBegin(OPTIMIZE_TASK_STAT_FIELD_DESC);
struct.optimizeTaskStat.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class reportOptimizeResult_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizeResult_argsTupleScheme getScheme() {
return new reportOptimizeResult_argsTupleScheme();
}
}
private static class reportOptimizeResult_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizeResult_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetOptimizeTaskStat()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetOptimizeTaskStat()) {
struct.optimizeTaskStat.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizeResult_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.optimizeTaskStat = new OptimizeTaskStat();
struct.optimizeTaskStat.read(iprot);
struct.setOptimizeTaskStatIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class reportOptimizeResult_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("reportOptimizeResult_result");
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new reportOptimizeResult_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new reportOptimizeResult_resultTupleSchemeFactory();
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
;
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(reportOptimizeResult_result.class, metaDataMap);
}
public reportOptimizeResult_result() {
}
/**
* Performs a deep copy on other.
*/
public reportOptimizeResult_result(reportOptimizeResult_result other) {
}
public reportOptimizeResult_result deepCopy() {
return new reportOptimizeResult_result(this);
}
@Override
public void clear() {
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof reportOptimizeResult_result)
return this.equals((reportOptimizeResult_result)that);
return false;
}
public boolean equals(reportOptimizeResult_result that) {
if (that == null)
return false;
if (this == that)
return true;
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
return hashCode;
}
@Override
public int compareTo(reportOptimizeResult_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("reportOptimizeResult_result(");
boolean first = true;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class reportOptimizeResult_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizeResult_resultStandardScheme getScheme() {
return new reportOptimizeResult_resultStandardScheme();
}
}
private static class reportOptimizeResult_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, reportOptimizeResult_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, reportOptimizeResult_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class reportOptimizeResult_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizeResult_resultTupleScheme getScheme() {
return new reportOptimizeResult_resultTupleScheme();
}
}
private static class reportOptimizeResult_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizeResult_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizeResult_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class reportOptimizerState_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("reportOptimizerState_args");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField REPORT_DATA_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("reportData", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new reportOptimizerState_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new reportOptimizerState_argsTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizerStateReport reportData; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
REPORT_DATA((short)1, "reportData");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REPORT_DATA
return REPORT_DATA;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REPORT_DATA, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("reportData", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, OptimizerStateReport.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(reportOptimizerState_args.class, metaDataMap);
}
public reportOptimizerState_args() {
}
public reportOptimizerState_args(
OptimizerStateReport reportData)
{
this();
this.reportData = reportData;
}
/**
* Performs a deep copy on other.
*/
public reportOptimizerState_args(reportOptimizerState_args other) {
if (other.isSetReportData()) {
this.reportData = new OptimizerStateReport(other.reportData);
}
}
public reportOptimizerState_args deepCopy() {
return new reportOptimizerState_args(this);
}
@Override
public void clear() {
this.reportData = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public OptimizerStateReport getReportData() {
return this.reportData;
}
public reportOptimizerState_args setReportData(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizerStateReport reportData) {
this.reportData = reportData;
return this;
}
public void unsetReportData() {
this.reportData = null;
}
/** Returns true if field reportData is set (has been assigned a value) and false otherwise */
public boolean isSetReportData() {
return this.reportData != null;
}
public void setReportDataIsSet(boolean value) {
if (!value) {
this.reportData = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REPORT_DATA:
if (value == null) {
unsetReportData();
} else {
setReportData((OptimizerStateReport)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REPORT_DATA:
return getReportData();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REPORT_DATA:
return isSetReportData();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof reportOptimizerState_args)
return this.equals((reportOptimizerState_args)that);
return false;
}
public boolean equals(reportOptimizerState_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_reportData = true && this.isSetReportData();
boolean that_present_reportData = true && that.isSetReportData();
if (this_present_reportData || that_present_reportData) {
if (!(this_present_reportData && that_present_reportData))
return false;
if (!this.reportData.equals(that.reportData))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetReportData()) ? 131071 : 524287);
if (isSetReportData())
hashCode = hashCode * 8191 + reportData.hashCode();
return hashCode;
}
@Override
public int compareTo(reportOptimizerState_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetReportData()).compareTo(other.isSetReportData());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetReportData()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.reportData, other.reportData);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("reportOptimizerState_args(");
boolean first = true;
sb.append("reportData:");
if (this.reportData == null) {
sb.append("null");
} else {
sb.append(this.reportData);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (reportData != null) {
reportData.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class reportOptimizerState_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizerState_argsStandardScheme getScheme() {
return new reportOptimizerState_argsStandardScheme();
}
}
private static class reportOptimizerState_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, reportOptimizerState_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REPORT_DATA
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.reportData = new OptimizerStateReport();
struct.reportData.read(iprot);
struct.setReportDataIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, reportOptimizerState_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.reportData != null) {
oprot.writeFieldBegin(REPORT_DATA_FIELD_DESC);
struct.reportData.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class reportOptimizerState_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizerState_argsTupleScheme getScheme() {
return new reportOptimizerState_argsTupleScheme();
}
}
private static class reportOptimizerState_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizerState_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetReportData()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetReportData()) {
struct.reportData.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizerState_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.reportData = new OptimizerStateReport();
struct.reportData.read(iprot);
struct.setReportDataIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class reportOptimizerState_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("reportOptimizerState_result");
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new reportOptimizerState_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new reportOptimizerState_resultTupleSchemeFactory();
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
;
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(reportOptimizerState_result.class, metaDataMap);
}
public reportOptimizerState_result() {
}
/**
* Performs a deep copy on other.
*/
public reportOptimizerState_result(reportOptimizerState_result other) {
}
public reportOptimizerState_result deepCopy() {
return new reportOptimizerState_result(this);
}
@Override
public void clear() {
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof reportOptimizerState_result)
return this.equals((reportOptimizerState_result)that);
return false;
}
public boolean equals(reportOptimizerState_result that) {
if (that == null)
return false;
if (this == that)
return true;
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
return hashCode;
}
@Override
public int compareTo(reportOptimizerState_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("reportOptimizerState_result(");
boolean first = true;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class reportOptimizerState_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizerState_resultStandardScheme getScheme() {
return new reportOptimizerState_resultStandardScheme();
}
}
private static class reportOptimizerState_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, reportOptimizerState_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, reportOptimizerState_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class reportOptimizerState_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public reportOptimizerState_resultTupleScheme getScheme() {
return new reportOptimizerState_resultTupleScheme();
}
}
private static class reportOptimizerState_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizerState_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, reportOptimizerState_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class registerOptimizer_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("registerOptimizer_args");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField REGISTER_INFO_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("registerInfo", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new registerOptimizer_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new registerOptimizer_argsTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizerRegisterInfo registerInfo; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
REGISTER_INFO((short)1, "registerInfo");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REGISTER_INFO
return REGISTER_INFO;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REGISTER_INFO, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("registerInfo", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, OptimizerRegisterInfo.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(registerOptimizer_args.class, metaDataMap);
}
public registerOptimizer_args() {
}
public registerOptimizer_args(
OptimizerRegisterInfo registerInfo)
{
this();
this.registerInfo = registerInfo;
}
/**
* Performs a deep copy on other.
*/
public registerOptimizer_args(registerOptimizer_args other) {
if (other.isSetRegisterInfo()) {
this.registerInfo = new OptimizerRegisterInfo(other.registerInfo);
}
}
public registerOptimizer_args deepCopy() {
return new registerOptimizer_args(this);
}
@Override
public void clear() {
this.registerInfo = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public OptimizerRegisterInfo getRegisterInfo() {
return this.registerInfo;
}
public registerOptimizer_args setRegisterInfo(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizerRegisterInfo registerInfo) {
this.registerInfo = registerInfo;
return this;
}
public void unsetRegisterInfo() {
this.registerInfo = null;
}
/** Returns true if field registerInfo is set (has been assigned a value) and false otherwise */
public boolean isSetRegisterInfo() {
return this.registerInfo != null;
}
public void setRegisterInfoIsSet(boolean value) {
if (!value) {
this.registerInfo = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REGISTER_INFO:
if (value == null) {
unsetRegisterInfo();
} else {
setRegisterInfo((OptimizerRegisterInfo)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REGISTER_INFO:
return getRegisterInfo();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REGISTER_INFO:
return isSetRegisterInfo();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof registerOptimizer_args)
return this.equals((registerOptimizer_args)that);
return false;
}
public boolean equals(registerOptimizer_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_registerInfo = true && this.isSetRegisterInfo();
boolean that_present_registerInfo = true && that.isSetRegisterInfo();
if (this_present_registerInfo || that_present_registerInfo) {
if (!(this_present_registerInfo && that_present_registerInfo))
return false;
if (!this.registerInfo.equals(that.registerInfo))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRegisterInfo()) ? 131071 : 524287);
if (isSetRegisterInfo())
hashCode = hashCode * 8191 + registerInfo.hashCode();
return hashCode;
}
@Override
public int compareTo(registerOptimizer_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetRegisterInfo()).compareTo(other.isSetRegisterInfo());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRegisterInfo()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.registerInfo, other.registerInfo);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("registerOptimizer_args(");
boolean first = true;
sb.append("registerInfo:");
if (this.registerInfo == null) {
sb.append("null");
} else {
sb.append(this.registerInfo);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (registerInfo != null) {
registerInfo.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class registerOptimizer_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public registerOptimizer_argsStandardScheme getScheme() {
return new registerOptimizer_argsStandardScheme();
}
}
private static class registerOptimizer_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, registerOptimizer_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REGISTER_INFO
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.registerInfo = new OptimizerRegisterInfo();
struct.registerInfo.read(iprot);
struct.setRegisterInfoIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, registerOptimizer_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.registerInfo != null) {
oprot.writeFieldBegin(REGISTER_INFO_FIELD_DESC);
struct.registerInfo.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class registerOptimizer_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public registerOptimizer_argsTupleScheme getScheme() {
return new registerOptimizer_argsTupleScheme();
}
}
private static class registerOptimizer_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, registerOptimizer_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRegisterInfo()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRegisterInfo()) {
struct.registerInfo.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, registerOptimizer_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.registerInfo = new OptimizerRegisterInfo();
struct.registerInfo.read(iprot);
struct.setRegisterInfoIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class registerOptimizer_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("registerOptimizer_result");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("success", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new registerOptimizer_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new registerOptimizer_resultTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizerDescriptor success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("success", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, OptimizerDescriptor.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(registerOptimizer_result.class, metaDataMap);
}
public registerOptimizer_result() {
}
public registerOptimizer_result(
OptimizerDescriptor success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public registerOptimizer_result(registerOptimizer_result other) {
if (other.isSetSuccess()) {
this.success = new OptimizerDescriptor(other.success);
}
}
public registerOptimizer_result deepCopy() {
return new registerOptimizer_result(this);
}
@Override
public void clear() {
this.success = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public OptimizerDescriptor getSuccess() {
return this.success;
}
public registerOptimizer_result setSuccess(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable OptimizerDescriptor success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((OptimizerDescriptor)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof registerOptimizer_result)
return this.equals((registerOptimizer_result)that);
return false;
}
public boolean equals(registerOptimizer_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(registerOptimizer_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetSuccess()).compareTo(other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("registerOptimizer_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class registerOptimizer_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public registerOptimizer_resultStandardScheme getScheme() {
return new registerOptimizer_resultStandardScheme();
}
}
private static class registerOptimizer_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, registerOptimizer_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new OptimizerDescriptor();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, registerOptimizer_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class registerOptimizer_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public registerOptimizer_resultTupleScheme getScheme() {
return new registerOptimizer_resultTupleScheme();
}
}
private static class registerOptimizer_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, registerOptimizer_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, registerOptimizer_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new OptimizerDescriptor();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class stopOptimize_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("stopOptimize_args");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField TABLE_IDENTIFIER_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("tableIdentifier", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new stopOptimize_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new stopOptimize_argsTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.TableIdentifier tableIdentifier; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
TABLE_IDENTIFIER((short)1, "tableIdentifier");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TABLE_IDENTIFIER
return TABLE_IDENTIFIER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TABLE_IDENTIFIER, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("tableIdentifier", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, com.netease.arctic.ams.api.TableIdentifier.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(stopOptimize_args.class, metaDataMap);
}
public stopOptimize_args() {
}
public stopOptimize_args(
com.netease.arctic.ams.api.TableIdentifier tableIdentifier)
{
this();
this.tableIdentifier = tableIdentifier;
}
/**
* Performs a deep copy on other.
*/
public stopOptimize_args(stopOptimize_args other) {
if (other.isSetTableIdentifier()) {
this.tableIdentifier = new com.netease.arctic.ams.api.TableIdentifier(other.tableIdentifier);
}
}
public stopOptimize_args deepCopy() {
return new stopOptimize_args(this);
}
@Override
public void clear() {
this.tableIdentifier = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public com.netease.arctic.ams.api.TableIdentifier getTableIdentifier() {
return this.tableIdentifier;
}
public stopOptimize_args setTableIdentifier(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.TableIdentifier tableIdentifier) {
this.tableIdentifier = tableIdentifier;
return this;
}
public void unsetTableIdentifier() {
this.tableIdentifier = null;
}
/** Returns true if field tableIdentifier is set (has been assigned a value) and false otherwise */
public boolean isSetTableIdentifier() {
return this.tableIdentifier != null;
}
public void setTableIdentifierIsSet(boolean value) {
if (!value) {
this.tableIdentifier = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case TABLE_IDENTIFIER:
if (value == null) {
unsetTableIdentifier();
} else {
setTableIdentifier((com.netease.arctic.ams.api.TableIdentifier)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case TABLE_IDENTIFIER:
return getTableIdentifier();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case TABLE_IDENTIFIER:
return isSetTableIdentifier();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof stopOptimize_args)
return this.equals((stopOptimize_args)that);
return false;
}
public boolean equals(stopOptimize_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_tableIdentifier = true && this.isSetTableIdentifier();
boolean that_present_tableIdentifier = true && that.isSetTableIdentifier();
if (this_present_tableIdentifier || that_present_tableIdentifier) {
if (!(this_present_tableIdentifier && that_present_tableIdentifier))
return false;
if (!this.tableIdentifier.equals(that.tableIdentifier))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetTableIdentifier()) ? 131071 : 524287);
if (isSetTableIdentifier())
hashCode = hashCode * 8191 + tableIdentifier.hashCode();
return hashCode;
}
@Override
public int compareTo(stopOptimize_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetTableIdentifier()).compareTo(other.isSetTableIdentifier());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetTableIdentifier()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.tableIdentifier, other.tableIdentifier);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("stopOptimize_args(");
boolean first = true;
sb.append("tableIdentifier:");
if (this.tableIdentifier == null) {
sb.append("null");
} else {
sb.append(this.tableIdentifier);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (tableIdentifier != null) {
tableIdentifier.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class stopOptimize_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public stopOptimize_argsStandardScheme getScheme() {
return new stopOptimize_argsStandardScheme();
}
}
private static class stopOptimize_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, stopOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // TABLE_IDENTIFIER
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.tableIdentifier = new com.netease.arctic.ams.api.TableIdentifier();
struct.tableIdentifier.read(iprot);
struct.setTableIdentifierIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, stopOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.tableIdentifier != null) {
oprot.writeFieldBegin(TABLE_IDENTIFIER_FIELD_DESC);
struct.tableIdentifier.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class stopOptimize_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public stopOptimize_argsTupleScheme getScheme() {
return new stopOptimize_argsTupleScheme();
}
}
private static class stopOptimize_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, stopOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetTableIdentifier()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetTableIdentifier()) {
struct.tableIdentifier.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, stopOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.tableIdentifier = new com.netease.arctic.ams.api.TableIdentifier();
struct.tableIdentifier.read(iprot);
struct.setTableIdentifierIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class stopOptimize_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("stopOptimize_result");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField E_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("e", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new stopOptimize_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new stopOptimize_resultTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.OperationErrorException e; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
E((short)1, "e");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // E
return E;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.E, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("e", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, com.netease.arctic.ams.api.OperationErrorException.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(stopOptimize_result.class, metaDataMap);
}
public stopOptimize_result() {
}
public stopOptimize_result(
com.netease.arctic.ams.api.OperationErrorException e)
{
this();
this.e = e;
}
/**
* Performs a deep copy on other.
*/
public stopOptimize_result(stopOptimize_result other) {
if (other.isSetE()) {
this.e = new com.netease.arctic.ams.api.OperationErrorException(other.e);
}
}
public stopOptimize_result deepCopy() {
return new stopOptimize_result(this);
}
@Override
public void clear() {
this.e = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public com.netease.arctic.ams.api.OperationErrorException getE() {
return this.e;
}
public stopOptimize_result setE(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.OperationErrorException e) {
this.e = e;
return this;
}
public void unsetE() {
this.e = null;
}
/** Returns true if field e is set (has been assigned a value) and false otherwise */
public boolean isSetE() {
return this.e != null;
}
public void setEIsSet(boolean value) {
if (!value) {
this.e = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case E:
if (value == null) {
unsetE();
} else {
setE((com.netease.arctic.ams.api.OperationErrorException)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case E:
return getE();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case E:
return isSetE();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof stopOptimize_result)
return this.equals((stopOptimize_result)that);
return false;
}
public boolean equals(stopOptimize_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_e = true && this.isSetE();
boolean that_present_e = true && that.isSetE();
if (this_present_e || that_present_e) {
if (!(this_present_e && that_present_e))
return false;
if (!this.e.equals(that.e))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetE()) ? 131071 : 524287);
if (isSetE())
hashCode = hashCode * 8191 + e.hashCode();
return hashCode;
}
@Override
public int compareTo(stopOptimize_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetE()).compareTo(other.isSetE());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetE()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.e, other.e);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("stopOptimize_result(");
boolean first = true;
sb.append("e:");
if (this.e == null) {
sb.append("null");
} else {
sb.append(this.e);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class stopOptimize_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public stopOptimize_resultStandardScheme getScheme() {
return new stopOptimize_resultStandardScheme();
}
}
private static class stopOptimize_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, stopOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // E
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.e = new com.netease.arctic.ams.api.OperationErrorException();
struct.e.read(iprot);
struct.setEIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, stopOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.e != null) {
oprot.writeFieldBegin(E_FIELD_DESC);
struct.e.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class stopOptimize_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public stopOptimize_resultTupleScheme getScheme() {
return new stopOptimize_resultTupleScheme();
}
}
private static class stopOptimize_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, stopOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetE()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetE()) {
struct.e.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, stopOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.e = new com.netease.arctic.ams.api.OperationErrorException();
struct.e.read(iprot);
struct.setEIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class startOptimize_args implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("startOptimize_args");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField TABLE_IDENTIFIER_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("tableIdentifier", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new startOptimize_argsStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new startOptimize_argsTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.TableIdentifier tableIdentifier; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
TABLE_IDENTIFIER((short)1, "tableIdentifier");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TABLE_IDENTIFIER
return TABLE_IDENTIFIER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TABLE_IDENTIFIER, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("tableIdentifier", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, com.netease.arctic.ams.api.TableIdentifier.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(startOptimize_args.class, metaDataMap);
}
public startOptimize_args() {
}
public startOptimize_args(
com.netease.arctic.ams.api.TableIdentifier tableIdentifier)
{
this();
this.tableIdentifier = tableIdentifier;
}
/**
* Performs a deep copy on other.
*/
public startOptimize_args(startOptimize_args other) {
if (other.isSetTableIdentifier()) {
this.tableIdentifier = new com.netease.arctic.ams.api.TableIdentifier(other.tableIdentifier);
}
}
public startOptimize_args deepCopy() {
return new startOptimize_args(this);
}
@Override
public void clear() {
this.tableIdentifier = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public com.netease.arctic.ams.api.TableIdentifier getTableIdentifier() {
return this.tableIdentifier;
}
public startOptimize_args setTableIdentifier(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.TableIdentifier tableIdentifier) {
this.tableIdentifier = tableIdentifier;
return this;
}
public void unsetTableIdentifier() {
this.tableIdentifier = null;
}
/** Returns true if field tableIdentifier is set (has been assigned a value) and false otherwise */
public boolean isSetTableIdentifier() {
return this.tableIdentifier != null;
}
public void setTableIdentifierIsSet(boolean value) {
if (!value) {
this.tableIdentifier = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case TABLE_IDENTIFIER:
if (value == null) {
unsetTableIdentifier();
} else {
setTableIdentifier((com.netease.arctic.ams.api.TableIdentifier)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case TABLE_IDENTIFIER:
return getTableIdentifier();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case TABLE_IDENTIFIER:
return isSetTableIdentifier();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof startOptimize_args)
return this.equals((startOptimize_args)that);
return false;
}
public boolean equals(startOptimize_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_tableIdentifier = true && this.isSetTableIdentifier();
boolean that_present_tableIdentifier = true && that.isSetTableIdentifier();
if (this_present_tableIdentifier || that_present_tableIdentifier) {
if (!(this_present_tableIdentifier && that_present_tableIdentifier))
return false;
if (!this.tableIdentifier.equals(that.tableIdentifier))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetTableIdentifier()) ? 131071 : 524287);
if (isSetTableIdentifier())
hashCode = hashCode * 8191 + tableIdentifier.hashCode();
return hashCode;
}
@Override
public int compareTo(startOptimize_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetTableIdentifier()).compareTo(other.isSetTableIdentifier());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetTableIdentifier()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.tableIdentifier, other.tableIdentifier);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("startOptimize_args(");
boolean first = true;
sb.append("tableIdentifier:");
if (this.tableIdentifier == null) {
sb.append("null");
} else {
sb.append(this.tableIdentifier);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (tableIdentifier != null) {
tableIdentifier.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class startOptimize_argsStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public startOptimize_argsStandardScheme getScheme() {
return new startOptimize_argsStandardScheme();
}
}
private static class startOptimize_argsStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, startOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // TABLE_IDENTIFIER
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.tableIdentifier = new com.netease.arctic.ams.api.TableIdentifier();
struct.tableIdentifier.read(iprot);
struct.setTableIdentifierIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, startOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.tableIdentifier != null) {
oprot.writeFieldBegin(TABLE_IDENTIFIER_FIELD_DESC);
struct.tableIdentifier.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class startOptimize_argsTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public startOptimize_argsTupleScheme getScheme() {
return new startOptimize_argsTupleScheme();
}
}
private static class startOptimize_argsTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, startOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetTableIdentifier()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetTableIdentifier()) {
struct.tableIdentifier.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, startOptimize_args struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.tableIdentifier = new com.netease.arctic.ams.api.TableIdentifier();
struct.tableIdentifier.read(iprot);
struct.setTableIdentifierIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class startOptimize_result implements com.netease.arctic.shade.org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TStruct STRUCT_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TStruct("startOptimize_result");
private static final com.netease.arctic.shade.org.apache.thrift.protocol.TField E_FIELD_DESC = new com.netease.arctic.shade.org.apache.thrift.protocol.TField("e", com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new startOptimize_resultStandardSchemeFactory();
private static final com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new startOptimize_resultTupleSchemeFactory();
public @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.OperationErrorException e; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements com.netease.arctic.shade.org.apache.thrift.TFieldIdEnum {
E((short)1, "e");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // E
return E;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.E, new com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData("e", com.netease.arctic.shade.org.apache.thrift.TFieldRequirementType.DEFAULT,
new com.netease.arctic.shade.org.apache.thrift.meta_data.StructMetaData(com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT, com.netease.arctic.ams.api.OperationErrorException.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
com.netease.arctic.shade.org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(startOptimize_result.class, metaDataMap);
}
public startOptimize_result() {
}
public startOptimize_result(
com.netease.arctic.ams.api.OperationErrorException e)
{
this();
this.e = e;
}
/**
* Performs a deep copy on other.
*/
public startOptimize_result(startOptimize_result other) {
if (other.isSetE()) {
this.e = new com.netease.arctic.ams.api.OperationErrorException(other.e);
}
}
public startOptimize_result deepCopy() {
return new startOptimize_result(this);
}
@Override
public void clear() {
this.e = null;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public com.netease.arctic.ams.api.OperationErrorException getE() {
return this.e;
}
public startOptimize_result setE(@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable com.netease.arctic.ams.api.OperationErrorException e) {
this.e = e;
return this;
}
public void unsetE() {
this.e = null;
}
/** Returns true if field e is set (has been assigned a value) and false otherwise */
public boolean isSetE() {
return this.e != null;
}
public void setEIsSet(boolean value) {
if (!value) {
this.e = null;
}
}
public void setFieldValue(_Fields field, @com.netease.arctic.shade.org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case E:
if (value == null) {
unsetE();
} else {
setE((com.netease.arctic.ams.api.OperationErrorException)value);
}
break;
}
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case E:
return getE();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case E:
return isSetE();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that == null)
return false;
if (that instanceof startOptimize_result)
return this.equals((startOptimize_result)that);
return false;
}
public boolean equals(startOptimize_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_e = true && this.isSetE();
boolean that_present_e = true && that.isSetE();
if (this_present_e || that_present_e) {
if (!(this_present_e && that_present_e))
return false;
if (!this.e.equals(that.e))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetE()) ? 131071 : 524287);
if (isSetE())
hashCode = hashCode * 8191 + e.hashCode();
return hashCode;
}
@Override
public int compareTo(startOptimize_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.valueOf(isSetE()).compareTo(other.isSetE());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetE()) {
lastComparison = com.netease.arctic.shade.org.apache.thrift.TBaseHelper.compareTo(this.e, other.e);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@com.netease.arctic.shade.org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot) throws com.netease.arctic.shade.org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("startOptimize_result(");
boolean first = true;
sb.append("e:");
if (this.e == null) {
sb.append("null");
} else {
sb.append(this.e);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws com.netease.arctic.shade.org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new com.netease.arctic.shade.org.apache.thrift.protocol.TCompactProtocol(new com.netease.arctic.shade.org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (com.netease.arctic.shade.org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class startOptimize_resultStandardSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public startOptimize_resultStandardScheme getScheme() {
return new startOptimize_resultStandardScheme();
}
}
private static class startOptimize_resultStandardScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme {
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol iprot, startOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // E
if (schemeField.type == com.netease.arctic.shade.org.apache.thrift.protocol.TType.STRUCT) {
struct.e = new com.netease.arctic.ams.api.OperationErrorException();
struct.e.read(iprot);
struct.setEIsSet(true);
} else {
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
com.netease.arctic.shade.org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol oprot, startOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.e != null) {
oprot.writeFieldBegin(E_FIELD_DESC);
struct.e.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class startOptimize_resultTupleSchemeFactory implements com.netease.arctic.shade.org.apache.thrift.scheme.SchemeFactory {
public startOptimize_resultTupleScheme getScheme() {
return new startOptimize_resultTupleScheme();
}
}
private static class startOptimize_resultTupleScheme extends com.netease.arctic.shade.org.apache.thrift.scheme.TupleScheme {
@Override
public void write(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, startOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol oprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetE()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetE()) {
struct.e.write(oprot);
}
}
@Override
public void read(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol prot, startOptimize_result struct) throws com.netease.arctic.shade.org.apache.thrift.TException {
com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol iprot = (com.netease.arctic.shade.org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.e = new com.netease.arctic.ams.api.OperationErrorException();
struct.e.read(iprot);
struct.setEIsSet(true);
}
}
}
private static S scheme(com.netease.arctic.shade.org.apache.thrift.protocol.TProtocol proto) {
return (com.netease.arctic.shade.org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy