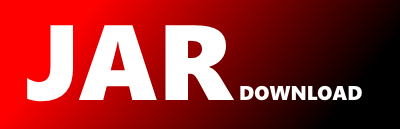
com.netease.arctic.utils.TablePropertyUtil Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.netease.arctic.utils;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.netease.arctic.table.KeyedTable;
import com.netease.arctic.table.TableProperties;
import com.netease.arctic.shade.org.apache.iceberg.PartitionSpec;
import com.netease.arctic.shade.org.apache.iceberg.Schema;
import com.netease.arctic.shade.org.apache.iceberg.StructLike;
import com.netease.arctic.shade.org.apache.iceberg.data.GenericRecord;
import com.netease.arctic.shade.org.apache.iceberg.relocated.com.google.common.collect.Maps;
import com.netease.arctic.shade.org.apache.iceberg.util.StructLikeMap;
import java.io.UncheckedIOException;
import java.util.Map;
/**
* Utils to handle table properties.
*/
public class TablePropertyUtil {
public static final StructLike EMPTY_STRUCT = GenericRecord.create(new Schema());
/**
* Decode string to max transaction id map of each partition.
*
* @param spec table partition spec
* @param value string value
* @return max transaction id map of each partition
*/
public static StructLikeMap decodePartitionMaxTxId(PartitionSpec spec, String value) {
try {
StructLikeMap results = StructLikeMap.create(spec.partitionType());
TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy