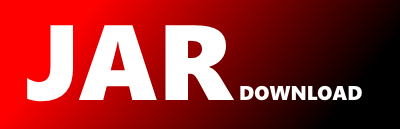
org.apache.spark.sql.catalyst.parser.extensions.IcebergSqlExtensionsListener Maven / Gradle / Ivy
The newest version!
// Generated from com.netease.arctic.shade.com.netease.arctic.shade.org.apache.spark.sql.catalyst.parser.extensions/IcebergSqlExtensions.g4 by ANTLR 4.7.1
package com.netease.arctic.shade.com.netease.arctic.shade.org.apache.spark.sql.catalyst.parser.extensions;
import org.antlr.v4.runtime.tree.ParseTreeListener;
/**
* This interface defines a complete listener for a parse tree produced by
* {@link IcebergSqlExtensionsParser}.
*/
public interface IcebergSqlExtensionsListener extends ParseTreeListener {
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#singleStatement}.
* @param ctx the parse tree
*/
void enterSingleStatement(IcebergSqlExtensionsParser.SingleStatementContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#singleStatement}.
* @param ctx the parse tree
*/
void exitSingleStatement(IcebergSqlExtensionsParser.SingleStatementContext ctx);
/**
* Enter a parse tree produced by the {@code call}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterCall(IcebergSqlExtensionsParser.CallContext ctx);
/**
* Exit a parse tree produced by the {@code call}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitCall(IcebergSqlExtensionsParser.CallContext ctx);
/**
* Enter a parse tree produced by the {@code addPartitionField}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterAddPartitionField(IcebergSqlExtensionsParser.AddPartitionFieldContext ctx);
/**
* Exit a parse tree produced by the {@code addPartitionField}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitAddPartitionField(IcebergSqlExtensionsParser.AddPartitionFieldContext ctx);
/**
* Enter a parse tree produced by the {@code dropPartitionField}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterDropPartitionField(IcebergSqlExtensionsParser.DropPartitionFieldContext ctx);
/**
* Exit a parse tree produced by the {@code dropPartitionField}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitDropPartitionField(IcebergSqlExtensionsParser.DropPartitionFieldContext ctx);
/**
* Enter a parse tree produced by the {@code replacePartitionField}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterReplacePartitionField(IcebergSqlExtensionsParser.ReplacePartitionFieldContext ctx);
/**
* Exit a parse tree produced by the {@code replacePartitionField}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitReplacePartitionField(IcebergSqlExtensionsParser.ReplacePartitionFieldContext ctx);
/**
* Enter a parse tree produced by the {@code setWriteDistributionAndOrdering}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterSetWriteDistributionAndOrdering(IcebergSqlExtensionsParser.SetWriteDistributionAndOrderingContext ctx);
/**
* Exit a parse tree produced by the {@code setWriteDistributionAndOrdering}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitSetWriteDistributionAndOrdering(IcebergSqlExtensionsParser.SetWriteDistributionAndOrderingContext ctx);
/**
* Enter a parse tree produced by the {@code setIdentifierFields}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterSetIdentifierFields(IcebergSqlExtensionsParser.SetIdentifierFieldsContext ctx);
/**
* Exit a parse tree produced by the {@code setIdentifierFields}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitSetIdentifierFields(IcebergSqlExtensionsParser.SetIdentifierFieldsContext ctx);
/**
* Enter a parse tree produced by the {@code dropIdentifierFields}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void enterDropIdentifierFields(IcebergSqlExtensionsParser.DropIdentifierFieldsContext ctx);
/**
* Exit a parse tree produced by the {@code dropIdentifierFields}
* labeled alternative in {@link IcebergSqlExtensionsParser#statement}.
* @param ctx the parse tree
*/
void exitDropIdentifierFields(IcebergSqlExtensionsParser.DropIdentifierFieldsContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#writeSpec}.
* @param ctx the parse tree
*/
void enterWriteSpec(IcebergSqlExtensionsParser.WriteSpecContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#writeSpec}.
* @param ctx the parse tree
*/
void exitWriteSpec(IcebergSqlExtensionsParser.WriteSpecContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#writeDistributionSpec}.
* @param ctx the parse tree
*/
void enterWriteDistributionSpec(IcebergSqlExtensionsParser.WriteDistributionSpecContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#writeDistributionSpec}.
* @param ctx the parse tree
*/
void exitWriteDistributionSpec(IcebergSqlExtensionsParser.WriteDistributionSpecContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#writeOrderingSpec}.
* @param ctx the parse tree
*/
void enterWriteOrderingSpec(IcebergSqlExtensionsParser.WriteOrderingSpecContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#writeOrderingSpec}.
* @param ctx the parse tree
*/
void exitWriteOrderingSpec(IcebergSqlExtensionsParser.WriteOrderingSpecContext ctx);
/**
* Enter a parse tree produced by the {@code positionalArgument}
* labeled alternative in {@link IcebergSqlExtensionsParser#callArgument}.
* @param ctx the parse tree
*/
void enterPositionalArgument(IcebergSqlExtensionsParser.PositionalArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code positionalArgument}
* labeled alternative in {@link IcebergSqlExtensionsParser#callArgument}.
* @param ctx the parse tree
*/
void exitPositionalArgument(IcebergSqlExtensionsParser.PositionalArgumentContext ctx);
/**
* Enter a parse tree produced by the {@code namedArgument}
* labeled alternative in {@link IcebergSqlExtensionsParser#callArgument}.
* @param ctx the parse tree
*/
void enterNamedArgument(IcebergSqlExtensionsParser.NamedArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code namedArgument}
* labeled alternative in {@link IcebergSqlExtensionsParser#callArgument}.
* @param ctx the parse tree
*/
void exitNamedArgument(IcebergSqlExtensionsParser.NamedArgumentContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#order}.
* @param ctx the parse tree
*/
void enterOrder(IcebergSqlExtensionsParser.OrderContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#order}.
* @param ctx the parse tree
*/
void exitOrder(IcebergSqlExtensionsParser.OrderContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#orderField}.
* @param ctx the parse tree
*/
void enterOrderField(IcebergSqlExtensionsParser.OrderFieldContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#orderField}.
* @param ctx the parse tree
*/
void exitOrderField(IcebergSqlExtensionsParser.OrderFieldContext ctx);
/**
* Enter a parse tree produced by the {@code identityTransform}
* labeled alternative in {@link IcebergSqlExtensionsParser#transform}.
* @param ctx the parse tree
*/
void enterIdentityTransform(IcebergSqlExtensionsParser.IdentityTransformContext ctx);
/**
* Exit a parse tree produced by the {@code identityTransform}
* labeled alternative in {@link IcebergSqlExtensionsParser#transform}.
* @param ctx the parse tree
*/
void exitIdentityTransform(IcebergSqlExtensionsParser.IdentityTransformContext ctx);
/**
* Enter a parse tree produced by the {@code applyTransform}
* labeled alternative in {@link IcebergSqlExtensionsParser#transform}.
* @param ctx the parse tree
*/
void enterApplyTransform(IcebergSqlExtensionsParser.ApplyTransformContext ctx);
/**
* Exit a parse tree produced by the {@code applyTransform}
* labeled alternative in {@link IcebergSqlExtensionsParser#transform}.
* @param ctx the parse tree
*/
void exitApplyTransform(IcebergSqlExtensionsParser.ApplyTransformContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#transformArgument}.
* @param ctx the parse tree
*/
void enterTransformArgument(IcebergSqlExtensionsParser.TransformArgumentContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#transformArgument}.
* @param ctx the parse tree
*/
void exitTransformArgument(IcebergSqlExtensionsParser.TransformArgumentContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#expression}.
* @param ctx the parse tree
*/
void enterExpression(IcebergSqlExtensionsParser.ExpressionContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#expression}.
* @param ctx the parse tree
*/
void exitExpression(IcebergSqlExtensionsParser.ExpressionContext ctx);
/**
* Enter a parse tree produced by the {@code numericLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void enterNumericLiteral(IcebergSqlExtensionsParser.NumericLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code numericLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void exitNumericLiteral(IcebergSqlExtensionsParser.NumericLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code booleanLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void enterBooleanLiteral(IcebergSqlExtensionsParser.BooleanLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code booleanLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void exitBooleanLiteral(IcebergSqlExtensionsParser.BooleanLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code stringLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void enterStringLiteral(IcebergSqlExtensionsParser.StringLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code stringLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void exitStringLiteral(IcebergSqlExtensionsParser.StringLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code typeConstructor}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void enterTypeConstructor(IcebergSqlExtensionsParser.TypeConstructorContext ctx);
/**
* Exit a parse tree produced by the {@code typeConstructor}
* labeled alternative in {@link IcebergSqlExtensionsParser#constant}.
* @param ctx the parse tree
*/
void exitTypeConstructor(IcebergSqlExtensionsParser.TypeConstructorContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#stringMap}.
* @param ctx the parse tree
*/
void enterStringMap(IcebergSqlExtensionsParser.StringMapContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#stringMap}.
* @param ctx the parse tree
*/
void exitStringMap(IcebergSqlExtensionsParser.StringMapContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#booleanValue}.
* @param ctx the parse tree
*/
void enterBooleanValue(IcebergSqlExtensionsParser.BooleanValueContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#booleanValue}.
* @param ctx the parse tree
*/
void exitBooleanValue(IcebergSqlExtensionsParser.BooleanValueContext ctx);
/**
* Enter a parse tree produced by the {@code exponentLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterExponentLiteral(IcebergSqlExtensionsParser.ExponentLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code exponentLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitExponentLiteral(IcebergSqlExtensionsParser.ExponentLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code decimalLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterDecimalLiteral(IcebergSqlExtensionsParser.DecimalLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code decimalLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitDecimalLiteral(IcebergSqlExtensionsParser.DecimalLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code integerLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterIntegerLiteral(IcebergSqlExtensionsParser.IntegerLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code integerLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitIntegerLiteral(IcebergSqlExtensionsParser.IntegerLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code bigIntLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterBigIntLiteral(IcebergSqlExtensionsParser.BigIntLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code bigIntLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitBigIntLiteral(IcebergSqlExtensionsParser.BigIntLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code smallIntLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterSmallIntLiteral(IcebergSqlExtensionsParser.SmallIntLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code smallIntLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitSmallIntLiteral(IcebergSqlExtensionsParser.SmallIntLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code tinyIntLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterTinyIntLiteral(IcebergSqlExtensionsParser.TinyIntLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code tinyIntLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitTinyIntLiteral(IcebergSqlExtensionsParser.TinyIntLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code doubleLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterDoubleLiteral(IcebergSqlExtensionsParser.DoubleLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code doubleLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitDoubleLiteral(IcebergSqlExtensionsParser.DoubleLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code floatLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterFloatLiteral(IcebergSqlExtensionsParser.FloatLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code floatLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitFloatLiteral(IcebergSqlExtensionsParser.FloatLiteralContext ctx);
/**
* Enter a parse tree produced by the {@code bigDecimalLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void enterBigDecimalLiteral(IcebergSqlExtensionsParser.BigDecimalLiteralContext ctx);
/**
* Exit a parse tree produced by the {@code bigDecimalLiteral}
* labeled alternative in {@link IcebergSqlExtensionsParser#number}.
* @param ctx the parse tree
*/
void exitBigDecimalLiteral(IcebergSqlExtensionsParser.BigDecimalLiteralContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#multipartIdentifier}.
* @param ctx the parse tree
*/
void enterMultipartIdentifier(IcebergSqlExtensionsParser.MultipartIdentifierContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#multipartIdentifier}.
* @param ctx the parse tree
*/
void exitMultipartIdentifier(IcebergSqlExtensionsParser.MultipartIdentifierContext ctx);
/**
* Enter a parse tree produced by the {@code unquotedIdentifier}
* labeled alternative in {@link IcebergSqlExtensionsParser#identifier}.
* @param ctx the parse tree
*/
void enterUnquotedIdentifier(IcebergSqlExtensionsParser.UnquotedIdentifierContext ctx);
/**
* Exit a parse tree produced by the {@code unquotedIdentifier}
* labeled alternative in {@link IcebergSqlExtensionsParser#identifier}.
* @param ctx the parse tree
*/
void exitUnquotedIdentifier(IcebergSqlExtensionsParser.UnquotedIdentifierContext ctx);
/**
* Enter a parse tree produced by the {@code quotedIdentifierAlternative}
* labeled alternative in {@link IcebergSqlExtensionsParser#identifier}.
* @param ctx the parse tree
*/
void enterQuotedIdentifierAlternative(IcebergSqlExtensionsParser.QuotedIdentifierAlternativeContext ctx);
/**
* Exit a parse tree produced by the {@code quotedIdentifierAlternative}
* labeled alternative in {@link IcebergSqlExtensionsParser#identifier}.
* @param ctx the parse tree
*/
void exitQuotedIdentifierAlternative(IcebergSqlExtensionsParser.QuotedIdentifierAlternativeContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#quotedIdentifier}.
* @param ctx the parse tree
*/
void enterQuotedIdentifier(IcebergSqlExtensionsParser.QuotedIdentifierContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#quotedIdentifier}.
* @param ctx the parse tree
*/
void exitQuotedIdentifier(IcebergSqlExtensionsParser.QuotedIdentifierContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#fieldList}.
* @param ctx the parse tree
*/
void enterFieldList(IcebergSqlExtensionsParser.FieldListContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#fieldList}.
* @param ctx the parse tree
*/
void exitFieldList(IcebergSqlExtensionsParser.FieldListContext ctx);
/**
* Enter a parse tree produced by {@link IcebergSqlExtensionsParser#nonReserved}.
* @param ctx the parse tree
*/
void enterNonReserved(IcebergSqlExtensionsParser.NonReservedContext ctx);
/**
* Exit a parse tree produced by {@link IcebergSqlExtensionsParser#nonReserved}.
* @param ctx the parse tree
*/
void exitNonReserved(IcebergSqlExtensionsParser.NonReservedContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy