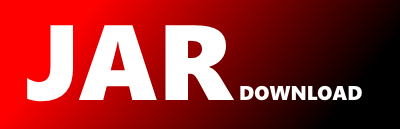
com.netease.cloud.services.nos.model.transform.Unmarshallers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nos-sdk-java-publiccloud Show documentation
Show all versions of nos-sdk-java-publiccloud Show documentation
nos java sdk to the Central Repository
The newest version!
package com.netease.cloud.services.nos.model.transform;
import java.io.InputStream;
import java.util.List;
import com.netease.cloud.services.nos.internal.DeleteObjectsResponse;
import com.netease.cloud.services.nos.model.Bucket;
import com.netease.cloud.services.nos.model.BucketLifecycleConfiguration;
import com.netease.cloud.services.nos.model.BucketVersioningConfiguration;
import com.netease.cloud.services.nos.model.DeduplicateResult;
import com.netease.cloud.services.nos.model.GetBucketDedupResult;
import com.netease.cloud.services.nos.model.GetBucketDefault404Result;
import com.netease.cloud.services.nos.model.GetBucketStatsResult;
import com.netease.cloud.services.nos.model.GetObjectVersionsResult;
import com.netease.cloud.services.nos.model.ImageMetadata;
import com.netease.cloud.services.nos.model.InitiateMultipartUploadResult;
import com.netease.cloud.services.nos.model.MultipartUploadListing;
import com.netease.cloud.services.nos.model.ObjectListing;
import com.netease.cloud.services.nos.model.PartListing;
import com.netease.cloud.services.nos.model.VersionListing;
import com.netease.cloud.services.nos.model.VideoMetadata;
import com.netease.cloud.services.nos.model.transform.XmlResponsesSaxParser.CompleteMultipartUploadHandler;
import com.netease.cloud.transform.Unmarshaller;
/**
* Collection of unmarshallers for Nos XML responses.
*/
public class Unmarshallers {
/**
* Unmarshaller for the ListBuckets XML response.
*/
public static final class ListBucketsUnmarshaller implements Unmarshaller, InputStream> {
public List unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListMyBucketsResponse(in).getBuckets();
}
}
/**
* Unmarshaller for the ListObjects XML response.
*/
public static final class ListObjectsUnmarshaller implements Unmarshaller {
public ObjectListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListBucketObjectsResponse(in).getObjectListing();
}
}
/**
* Unmarshaller for the BucketLocation XML response.
*/
public static final class BucketLocationUnmarshaller implements Unmarshaller {
public String unmarshall(InputStream in) throws Exception {
String location = new XmlResponsesSaxParser().parseBucketLocationResponse(in);
/*
* Nos treats the US location differently, and assumes that if the
* reported location is null, then it's a US bucket.
*/
if (location == null)
location = "HZ";
return location;
}
}
/**
* Unmarshaller for the BucketVersionConfiguration XML response.
*/
public static final class BucketVersioningConfigurationUnmarshaller implements
Unmarshaller {
public BucketVersioningConfiguration unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseVersioningConfigurationResponse(in).getConfiguration();
}
}
/**
* Unmarshaller for the a direct InputStream response.
*/
public static final class InputStreamUnmarshaller implements Unmarshaller {
public InputStream unmarshall(InputStream in) throws Exception {
return in;
}
}
public static final class CompleteMultipartUploadResultUnmarshaller implements
Unmarshaller {
public CompleteMultipartUploadHandler unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseCompleteMultipartUploadResponse(in);
}
}
public static final class InitiateMultipartUploadResultUnmarshaller implements
Unmarshaller {
public InitiateMultipartUploadResult unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseInitiateMultipartUploadResponse(in)
.getInitiateMultipartUploadResult();
}
}
public static final class ListMultipartUploadsResultUnmarshaller implements
Unmarshaller {
public MultipartUploadListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListMultipartUploadsResponse(in).getListMultipartUploadsResult();
}
}
public static final class ListPartsResultUnmarshaller implements Unmarshaller {
public PartListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListPartsResponse(in).getListPartsResult();
}
}
public static final class DeleteObjectsResultUnmarshaller implements
Unmarshaller {
public DeleteObjectsResponse unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseDeletedObjectsResult(in).getDeleteObjectResult();
}
}
public static final class GetBucketDedupResultUnmarshaller implements
Unmarshaller {
public GetBucketDedupResult unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseGetBucketDedupResponse(in).getResult();
}
}
public static final class GetBucketStatsUnmarshaller implements
Unmarshaller {
@Override
public GetBucketStatsResult unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseGetBucketStats(in).getResult();
}
}
public static final class GetBucketDefault404Unmarshaller implements
Unmarshaller {
public GetBucketDefault404Result unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseGetBucketDefault404Response(in).getResult();
}
}
public static final class DeduplicateResultUnmarshaller implements Unmarshaller {
public DeduplicateResult unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseDeduplicateResponse(in).getResult();
}
}
public static final class GetObejctVersionsResultUnmarshaller implements
Unmarshaller {
public GetObjectVersionsResult unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseGetObjectVersionsResponse(in).getObjectVersionsResult();
}
}
/**
* Unmarshaller for the ListVersions XML response.
*/
public static final class VersionListUnmarshaller implements
Unmarshaller {
public VersionListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser()
.parseListVersionsResponse(in).getListing();
}
}
public static final class BucketLifecycleConfigurationUnmarshaller implements
Unmarshaller {
public BucketLifecycleConfiguration unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseBucketLifecycleConfigurationResponse(in).getConfiguration();
}
}
/**
* Unmarshaller for the getImageMetaInfo XML response.
*/
public static final class GetImageMetaInfoUnmarshaller implements
Unmarshaller{
public ImageMetadata unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseGetImageMetaInfoResponse(in).getImageMetadata();
}
}
/**
* Unmarshaller for the GetVideoMetaInfo XML response.
*/
public static final class GetVideoMetaInfoUnmarshaller implements
Unmarshaller{
public VideoMetadata unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseGetVideoMetaInfoResponse(in).getVideoMetadata();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy