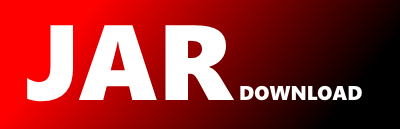
com.netflix.java.refactor.JavaSource.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-source-refactor Show documentation
Show all versions of java-source-refactor Show documentation
Pluggable and distributed refactoring tool for Java source code
package com.netflix.java.refactor
import com.netflix.java.refactor.find.*
import com.sun.tools.javac.code.Symbol
import com.sun.tools.javac.tree.JCTree
import java.nio.file.Files
import java.util.function.Consumer
import kotlin.concurrent.thread
class JavaSource(internal val cu: CompilationUnit) {
var changedFile = false
fun file() = cu.source()
fun text() = String(Files.readAllBytes(cu.source()))
fun classes() = cu.jcCompilationUnit.defs
.filterIsInstance()
.map { it.sym }
.filterIsInstance()
.map { it.toString() }
internal var lastCommitChangedFile = false
fun refactor(): RefactorTransaction {
val tx = RefactorTransaction(this)
if(lastCommitChangedFile) {
cu.reparse()
lastCommitChangedFile = false
}
return tx
}
fun hasType(clazz: Class<*>): Boolean = HasType(clazz.name).scanner().scan(cu)
fun hasType(clazz: String): Boolean = HasType(clazz).scanner().scan(cu)
/**
* Find fields defined on this class, but do not include inherited fields up the type hierarchy
*/
fun findFields(clazz: Class<*>): List = FindFields(clazz.name, false).scanner().scan(cu)
fun findFields(clazz: String): List = FindFields(clazz, false).scanner().scan(cu)
/**
* Find fields defined both on this class and visible inherited fields up the type hierarchy
*/
fun findFieldsIncludingInherited(clazz: Class<*>): List = FindFields(clazz.name, true).scanner().scan(cu)
fun findFieldsIncludingInherited(clazz: String): List = FindFields(clazz, true).scanner().scan(cu)
fun findMethodCalls(signature: String): List = FindMethods(signature).scanner().scan(cu)
fun diff(body: JavaSource.() -> Unit): String {
val before = text()
this.body()
val after = text()
return InMemoryDiffEntry(file().toString(), before, after).diff
}
fun beginDiff() = JavaSourceDiff(this)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy