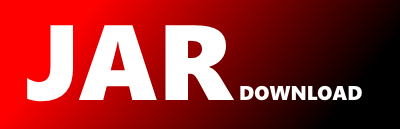
com.netflix.dyno.connectionpool.impl.HostSelectionStrategy Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2011 Netflix
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.netflix.dyno.connectionpool.impl;
import com.netflix.dyno.connectionpool.BaseOperation;
import com.netflix.dyno.connectionpool.Connection;
import com.netflix.dyno.connectionpool.Host;
import com.netflix.dyno.connectionpool.HostConnectionPool;
import com.netflix.dyno.connectionpool.exception.NoAvailableHostsException;
import com.netflix.dyno.connectionpool.impl.lb.HostToken;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* Interface that encapsulates a strategy for selecting a {@link Connection} to a {@link Host} for the given {@link BaseOperation}
*
* @param
* @author poberai
*/
public interface HostSelectionStrategy {
/**
* @param op
* @param hashtag
* @return
* @throws NoAvailableHostsException
*/
HostConnectionPool getPoolForOperation(BaseOperation op, String hashtag)
throws NoAvailableHostsException;
/**
* @param ops
* @return
* @throws NoAvailableHostsException
*/
Map, BaseOperation> getPoolsForOperationBatch(Collection> ops) throws NoAvailableHostsException;
/**
* @return
*/
List> getOrderedHostPools();
/**
* @param token
* @return
*/
HostConnectionPool getPoolForToken(Long token);
/**
* @param start
* @param end
* @return
*/
List> getPoolsForTokens(Long start, Long end);
/**
* Finds the server Host that owns the specified key.
*
* @param key
* @return {@link HostToken}
* @throws UnsupportedOperationException for non-token aware load balancing strategies
*/
HostToken getTokenForKey(String key) throws UnsupportedOperationException;
/**
* Finds the server Host that owns the specified binary key.
*
* @param key
* @return {@link HostToken}
* @throws UnsupportedOperationException for non-token aware load balancing strategies
*/
HostToken getTokenForKey(byte[] key) throws UnsupportedOperationException;
/**
* Init the connection pool with the set of hosts provided
*
* @param hostPools
*/
void initWithHosts(Map> hostPools);
/**
* Add a host to the selection strategy. This is useful when the underlying dynomite topology changes.
*
* @param {@link com.netflix.dyno.connectionpool.impl.lb.HostToken}
* @param hostPool
* @return true/false indicating whether the pool was indeed added
*/
boolean addHostPool(HostToken host, HostConnectionPool hostPool);
/**
* Remove a host from the selection strategy. This is useful when the underlying dynomite topology changes.
*
* @param {@link com.netflix.dyno.connectionpool.impl.lb.HostToken}
* @return true/false indicating whether the pool was indeed removed
*/
boolean removeHostPool(HostToken host);
boolean isTokenAware();
boolean isEmpty();
interface HostSelectionStrategyFactory {
/**
* Create/Return a HostSelectionStrategy
*
* @return HostSelectionStrategy
*/
public HostSelectionStrategy vendPoolSelectionStrategy();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy