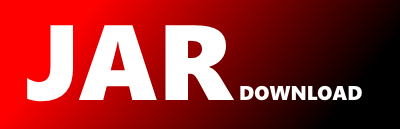
com.netflix.eureka2.interests.Interests Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eureka-core Show documentation
Show all versions of eureka-core Show documentation
eureka-core developed by Netflix
The newest version!
package com.netflix.eureka2.interests;
import com.netflix.eureka2.interests.Interest.Operator;
import com.netflix.eureka2.registry.InstanceInfo;
/**
* A factory to create instances of {@link Interest}.
*
* @author Nitesh Kant
*/
public final class Interests {
private Interests() {
}
public static Interest forVips(String... vips) {
return forVips(Operator.Equals, vips);
}
public static Interest forVips(Operator operator, String... vips) {
if (vips.length == 0) {
return EmptyRegistryInterest.getInstance();
}
if (vips.length == 1) {
return new VipInterest(vips[0], operator);
}
Interest[] interests = new Interest[vips.length];
for (int i = 0; i < interests.length; i++) {
interests[i] = new VipInterest(vips[i], operator);
}
return new MultipleInterests(interests);
}
public static Interest forApplications(String... applicationNames) {
return forApplications(Operator.Equals, applicationNames);
}
public static Interest forApplications(Operator operator, String... applicationNames) {
if (applicationNames.length == 0) {
return EmptyRegistryInterest.getInstance();
}
if (applicationNames.length == 1) {
return new ApplicationInterest(applicationNames[0], operator);
}
Interest[] interests = new Interest[applicationNames.length];
for (int i = 0; i < interests.length; i++) {
interests[i] = new ApplicationInterest(applicationNames[i], operator);
}
return new MultipleInterests(interests);
}
public static Interest forInstances(String... instanceIds) {
return forInstance(Operator.Equals, instanceIds);
}
public static Interest forInstance(Operator operator, String... instanceIds) {
if (instanceIds.length == 0) {
return EmptyRegistryInterest.getInstance();
}
if (instanceIds.length == 1) {
return new InstanceInterest(instanceIds[0], operator);
}
Interest[] interests = new Interest[instanceIds.length];
for (int i = 0; i < interests.length; i++) {
interests[i] = new InstanceInterest(instanceIds[i], operator);
}
return new MultipleInterests(interests);
}
public static Interest forFullRegistry() {
return FullRegistryInterest.getInstance();
}
public static Interest forNone() {
return EmptyRegistryInterest.getInstance();
}
public static Interest forSome(Interest... interests) {
return new MultipleInterests<>(interests);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy