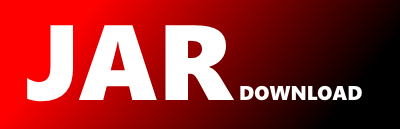
feign.Feign Maven / Gradle / Ivy
/*
* Copyright 2013 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package feign;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import javax.inject.Inject;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLSocketFactory;
import dagger.ObjectGraph;
import dagger.Provides;
import feign.Logger.NoOpLogger;
import feign.Request.Options;
import feign.Target.HardCodedTarget;
import feign.codec.Decoder;
import feign.codec.Encoder;
import feign.codec.ErrorDecoder;
/**
* Feign's purpose is to ease development against http apis that feign restfulness.
In
* implementation, Feign is a {@link Feign#newInstance factory} for generating {@link Target
* targeted} http apis.
*/
public abstract class Feign {
public static Builder builder() {
return new Builder();
}
public static T create(Class apiType, String url, Object... modules) {
return create(new HardCodedTarget(apiType, url), modules);
}
/**
* Shortcut to {@link #newInstance(Target) create} a single {@code targeted} http api using {@link
* ReflectiveFeign reflection}.
*/
public static T create(Target target, Object... modules) {
return create(modules).newInstance(target);
}
/**
* Returns a {@link ReflectiveFeign reflective} factory for generating {@link Target targeted}
* http apis.
*/
public static Feign create(Object... modules) {
return ObjectGraph.create(modulesForGraph(modules).toArray()).get(Feign.class);
}
/**
* Returns an {@link ObjectGraph Dagger ObjectGraph} that can inject a {@link ReflectiveFeign
* reflective} Feign.
*/
public static ObjectGraph createObjectGraph(Object... modules) {
return ObjectGraph.create(modulesForGraph(modules).toArray());
}
/**
*
Configuration keys are formatted as unresolved see tags.
For example. - {@code Route53}: would match a class such as {@code
* denominator.route53.Route53}
- {@code Route53#list()}: would match a method such as {@code
* denominator.route53.Route53#list()}
- {@code Route53#listAt(Marker)}: would match a method
* such as {@code denominator.route53.Route53#listAt(denominator.route53.Marker)}
- {@code
* Route53#listByNameAndType(String, String)}: would match a method such as {@code
* denominator.route53.Route53#listAt(String, String)}
Note that there is no whitespace
* expected in a key!
*/
public static String configKey(Method method) {
StringBuilder builder = new StringBuilder();
builder.append(method.getDeclaringClass().getSimpleName());
builder.append('#').append(method.getName()).append('(');
for (Class> param : method.getParameterTypes()) {
builder.append(param.getSimpleName()).append(',');
}
if (method.getParameterTypes().length > 0) {
builder.deleteCharAt(builder.length() - 1);
}
return builder.append(')').toString();
}
private static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy