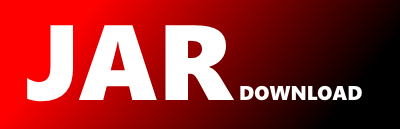
main.com.netflix.graphql.dgs.internal.DefaultDgsGraphQLContextBuilder.kt Maven / Gradle / Ivy
/*
* Copyright 2021 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.netflix.graphql.dgs.internal
import com.netflix.graphql.dgs.context.DgsContext
import com.netflix.graphql.dgs.context.DgsCustomContextBuilder
import com.netflix.graphql.dgs.context.DgsCustomContextBuilderWithRequest
import org.slf4j.Logger
import org.slf4j.LoggerFactory
import org.springframework.http.HttpHeaders
import org.springframework.web.context.request.WebRequest
import java.util.*
import kotlin.time.measureTimedValue
open class DefaultDgsGraphQLContextBuilder(
private val dgsCustomContextBuilder: Optional>,
private val dgsCustomContextBuilderWithRequest: Optional> = Optional.empty(),
) {
fun build(dgsRequestData: DgsWebMvcRequestData): DgsContext {
val (context, elapsed) = measureTimedValue { buildDgsContext(dgsRequestData) }
logger.debug("Created DGS context in {}ms", elapsed.inWholeMilliseconds)
return context
}
private fun buildDgsContext(dgsRequestData: DgsWebMvcRequestData?): DgsContext {
val customContext =
when {
dgsCustomContextBuilderWithRequest.isPresent ->
dgsCustomContextBuilderWithRequest.get().build(
dgsRequestData?.extensions ?: mapOf(),
HttpHeaders.readOnlyHttpHeaders(
dgsRequestData?.headers
?: HttpHeaders(),
),
dgsRequestData?.webRequest,
)
dgsCustomContextBuilder.isPresent -> dgsCustomContextBuilder.get().build()
else
// This is for backwards compatibility - we previously made DefaultRequestData the custom context if no custom context was provided.
-> dgsRequestData
}
return DgsContext(
customContext,
dgsRequestData,
)
}
companion object {
private val logger: Logger = LoggerFactory.getLogger(DefaultDgsGraphQLContextBuilder::class.java)
}
}
@Deprecated("Use DgsContext.requestData instead")
data class DefaultRequestData(
@Deprecated("Use DgsContext.requestData instead") val extensions: Map,
@Deprecated("Use DgsContext.requestData instead") val headers: HttpHeaders,
)
interface DgsRequestData {
val extensions: Map?
val headers: HttpHeaders?
}
/**
* @param extensions Optional map of extensions - useful for customized GraphQL interactions between for example a gateway and dgs.
* @param headers Http Headers
* @param webRequest Spring [WebRequest]. This will only be available when deployed in a WebMVC (Servlet based) environment.
*/
data class DgsWebMvcRequestData(
override val extensions: Map? = null,
override val headers: HttpHeaders? = null,
val webRequest: WebRequest? = null,
) : DgsRequestData
© 2015 - 2025 Weber Informatics LLC | Privacy Policy