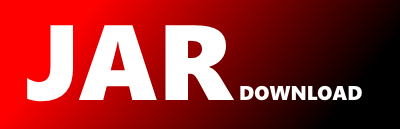
com.netflix.graphql.dgs.DgsDataFetchingEnvironment.kt Maven / Gradle / Ivy
/*
* Copyright 2021 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.netflix.graphql.dgs
import com.netflix.graphql.dgs.context.DgsContext
import com.netflix.graphql.dgs.exceptions.MultipleDataLoadersDefinedException
import com.netflix.graphql.dgs.exceptions.NoDataLoaderFoundException
import graphql.GraphQLContext
import graphql.cachecontrol.CacheControl
import graphql.execution.ExecutionId
import graphql.execution.ExecutionStepInfo
import graphql.execution.MergedField
import graphql.execution.directives.QueryDirectives
import graphql.language.Document
import graphql.language.Field
import graphql.language.FragmentDefinition
import graphql.language.OperationDefinition
import graphql.schema.*
import org.dataloader.DataLoader
import org.dataloader.DataLoaderRegistry
import java.util.*
class DgsDataFetchingEnvironment(private val dfe: DataFetchingEnvironment) : DataFetchingEnvironment {
fun getDgsContext(): DgsContext {
val context = dfe.getContext()
if (context is DgsContext) {
return context
} else {
throw RuntimeException("""Context object of type '${context::class.java.name}' is not a DgsContext. This method does not work if you have a custom implementation of DgsContextBuilder""")
}
}
fun getDataLoader(loaderClass: Class<*>): DataLoader {
val annotation = loaderClass.getAnnotation(DgsDataLoader::class.java)
return if (annotation != null) {
dfe.getDataLoader(annotation.name)
} else {
val loaders = loaderClass.fields.filter { it.isAnnotationPresent(DgsDataLoader::class.java) }
if (loaders.size > 1) throw MultipleDataLoadersDefinedException(loaderClass)
val loaderName = loaders
.firstOrNull()?.getAnnotation(DgsDataLoader::class.java)?.name
?: throw NoDataLoaderFoundException(loaderClass)
dfe.getDataLoader(loaderName)
}
}
override fun getSource(): T {
return dfe.getSource()
}
override fun getArguments(): MutableMap {
return dfe.arguments
}
override fun containsArgument(name: String?): Boolean {
return dfe.containsArgument(name)
}
override fun getArgument(name: String?): T {
return dfe.getArgument(name)
}
override fun getArgumentOrDefault(name: String?, defaultValue: T): T {
return dfe.getArgumentOrDefault(name, defaultValue)
}
@Deprecated(message = "Use getGraphQLContext() instead.", replaceWith = ReplaceWith(expression = "getGraphQLContext().get()"))
override fun getContext(): T {
return dfe.getContext()
}
override fun getGraphQlContext(): GraphQLContext {
return dfe.graphQlContext
}
override fun getLocalContext(): T {
return dfe.getLocalContext()
}
override fun getRoot(): T {
return dfe.getRoot()
}
override fun getFieldDefinition(): GraphQLFieldDefinition {
return dfe.fieldDefinition
}
@Deprecated("Use getMergedField()")
override fun getFields(): MutableList {
@Suppress("DEPRECATION")
return dfe.fields
}
override fun getMergedField(): MergedField {
return dfe.mergedField
}
override fun getField(): Field {
return dfe.field
}
override fun getFieldType(): GraphQLOutputType {
return dfe.fieldType
}
override fun getExecutionStepInfo(): ExecutionStepInfo {
return dfe.executionStepInfo
}
override fun getParentType(): GraphQLType {
return dfe.parentType
}
override fun getGraphQLSchema(): GraphQLSchema {
return dfe.graphQLSchema
}
override fun getFragmentsByName(): MutableMap {
return dfe.fragmentsByName
}
override fun getExecutionId(): ExecutionId {
return dfe.executionId
}
override fun getSelectionSet(): DataFetchingFieldSelectionSet {
return dfe.selectionSet
}
override fun getQueryDirectives(): QueryDirectives {
return dfe.queryDirectives
}
override fun getDataLoader(dataLoaderName: String?): DataLoader {
return dfe.getDataLoader(dataLoaderName)
}
override fun getDataLoaderRegistry(): DataLoaderRegistry {
return dfe.dataLoaderRegistry
}
override fun getCacheControl(): CacheControl {
return dfe.cacheControl
}
override fun getLocale(): Locale {
return dfe.locale
}
override fun getOperationDefinition(): OperationDefinition {
return dfe.operationDefinition
}
override fun getDocument(): Document {
return dfe.document
}
override fun getVariables(): MutableMap {
return dfe.variables
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy