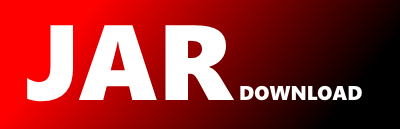
com.netflix.hollow.tools.util.ObjectInternPool Maven / Gradle / Ivy
package com.netflix.hollow.tools.util;
import com.netflix.hollow.core.memory.ByteArrayOrdinalMap;
import com.netflix.hollow.core.memory.ByteDataArray;
import com.netflix.hollow.core.memory.ByteData;
import com.netflix.hollow.core.memory.encoding.VarInt;
import com.netflix.hollow.core.schema.HollowObjectSchema.FieldType;
import java.util.HashSet;
import java.util.Optional;
// This class memoizes types by returning references to existing objects, or storing
// Objects if they are not currently in the pool
public class ObjectInternPool {
final private ByteArrayOrdinalMap ordinalMap;
private boolean isReadyToRead = false;
HashSet ordinalsInCycle;
public ObjectInternPool() {
this.ordinalMap = new ByteArrayOrdinalMap(1024);
this.ordinalsInCycle = new HashSet<>();
}
public void prepareForRead() {
if(!isReadyToRead) {
ordinalMap.prepareForWrite();
}
ordinalsInCycle.clear();
isReadyToRead = true;
}
public boolean ordinalInCurrentCycle(int ordinal) {
return ordinalsInCycle.contains(ordinal);
}
public Object getObject(int ordinal, FieldType type) {
long pointer = ordinalMap.getPointerForData(ordinal);
switch (type) {
case BOOLEAN:
return getBoolean(pointer);
case FLOAT:
return getFloat(pointer);
case DOUBLE:
return getDouble(pointer);
case INT:
return getInt(pointer);
case LONG:
return getLong(pointer);
case STRING:
return getString(pointer);
default:
throw new IllegalArgumentException("Unknown type " + type);
}
}
public boolean getBoolean(long pointer) {
ByteData byteData = ordinalMap.getByteData().getUnderlyingArray();
return byteData.get(pointer) == 1;
}
public float getFloat(long pointer) {
ByteData byteData = ordinalMap.getByteData().getUnderlyingArray();
int intBytes = VarInt.readVInt(byteData, pointer);
return Float.intBitsToFloat(intBytes);
}
public double getDouble(long pointer) {
ByteData byteData = ordinalMap.getByteData().getUnderlyingArray();
long longBytes = VarInt.readVLong(byteData, pointer);
return Double.longBitsToDouble(longBytes);
}
public int getInt(long pointer) {
ByteData byteData = ordinalMap.getByteData().getUnderlyingArray();
return VarInt.readVInt(byteData, pointer);
}
public long getLong(long pointer) {
ByteData byteData = ordinalMap.getByteData().getUnderlyingArray();
return VarInt.readVLong(byteData, pointer);
}
public String getString(long pointer) {
ByteData byteData = ordinalMap.getByteData().getUnderlyingArray();
int length = VarInt.readVInt(byteData, pointer);
byte[] bytes = new byte[length];
for(int i=0;i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy