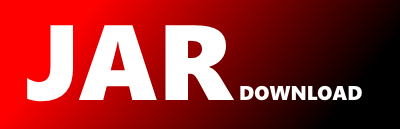
com.netflix.karyon.admin.rest.Interpolator Maven / Gradle / Ivy
package com.netflix.karyon.admin.rest;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.text.StrLookup;
import org.apache.commons.lang3.text.StrSubstitutor;
import com.netflix.archaius.Config;
public class Interpolator {
public Interpolator create(StrLookup lookup) {
return new Interpolator(lookup);
}
private List> lookups;
public Interpolator(StrLookup lookup) {
this.lookups = Arrays.asList(lookup);
}
public Interpolator(Map valueMap) {
this.lookups = Arrays.asList(StrLookup.mapLookup(valueMap));
}
public Interpolator(List> lookups) {
this.lookups = new ArrayList<>(lookups);
}
private static StrLookup fromConfig(final Config config) {
return new StrLookup() {
@Override
public String lookup(String key) {
return config.getString(key);
}
};
}
public Interpolator(Config config) {
this.lookups = Arrays.asList(fromConfig(config));
}
public Interpolator withFallback(StrLookup lookup) {
List> lookups = new ArrayList<>(this.lookups);
lookups.add(lookup);
return new Interpolator(lookups);
}
public Interpolator withFallback(Map valueMap) {
List> lookups = new ArrayList<>(this.lookups);
lookups.add(StrLookup.mapLookup(valueMap));
return new Interpolator(lookups);
}
public Interpolator withFallback(Config config) {
List> lookups = new ArrayList<>(this.lookups);
lookups.add(fromConfig(config));
return new Interpolator(lookups);
}
public String interpolate(String value) {
return new StrSubstitutor(
new StrLookup() {
@Override
public String lookup(String key) {
for (StrLookup lookup : lookups) {
String value = lookup.lookup(key);
if (value != null) {
return value;
}
}
return null;
}
}, "${", "}", '$')
.replace(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy