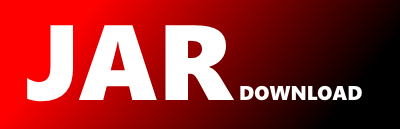
com.netflix.client.http.CaseInsensitiveMultiMap Maven / Gradle / Ivy
package com.netflix.client.http;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.AbstractMap.SimpleEntry;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.Lists;
import com.google.common.collect.Multimap;
public class CaseInsensitiveMultiMap implements HttpHeaders {
Multimap> map = ArrayListMultimap.create();
@Override
public String getFirstValue(String headerName) {
Collection> entries = map.get(headerName.toLowerCase());
if (entries == null || entries.isEmpty()) {
return null;
}
return entries.iterator().next().getValue();
}
@Override
public List getAllValues(String headerName) {
Collection> entries = map.get(headerName.toLowerCase());
List values = Lists.newArrayList();
if (entries != null) {
for (Entry entry: entries) {
values.add(entry.getValue());
}
}
return values;
}
@Override
public List> getAllHeaders() {
Collection> all = map.values();
return new ArrayList>(all);
}
@Override
public boolean containsHeader(String name) {
return map.containsKey(name.toLowerCase());
}
public void addHeader(String name, String value) {
if (getAllValues(name).contains(value)) {
return;
}
SimpleEntry entry = new SimpleEntry(name, value);
map.put(name.toLowerCase(), entry);
}
Map> asMap() {
Multimap result = ArrayListMultimap.create();
Collection> all = map.values();
for (Entry entry: all) {
result.put(entry.getKey(), entry.getValue());
}
return result.asMap();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy