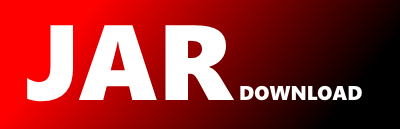
com.netflix.loadbalancer.reactive.ExecutionContext Maven / Gradle / Ivy
/*
*
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.netflix.loadbalancer.reactive;
import com.netflix.client.RetryHandler;
import com.netflix.client.config.IClientConfig;
import com.netflix.client.config.IClientConfigKey;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* A context object that is created at start of each load balancer execution
* and contains certain meta data of the load balancer and mutable state data of
* execution per listener per request. Each listener will get its own context
* to work with. But it can also call {@link ExecutionContext#getGlobalContext()} to
* get the shared context between all listeners.
*
* @author Allen Wang
*
*/
public class ExecutionContext {
private final Map context;
private final ConcurrentHashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy