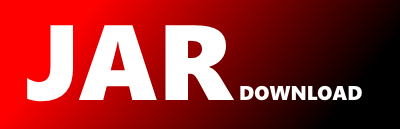
rx.Notification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rxjava-core Show documentation
Show all versions of rxjava-core Show documentation
rxjava-core developed by Netflix
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx;
/**
* An object representing a notification sent to an {@link Observable}.
*
* For the Microsoft Rx equivalent see: http://msdn.microsoft.com/en-us/library/hh229462(v=vs.103).aspx
*/
public class Notification {
private final Kind kind;
private final Throwable throwable;
private final T value;
private static final Notification ON_COMPLETED = new Notification(Kind.OnCompleted, null, null);
public static Notification createOnNext(T t) {
return new Notification(Kind.OnNext, t, null);
}
public static Notification createOnError(Throwable e) {
return new Notification(Kind.OnError, null, e);
}
@SuppressWarnings("unchecked")
public static Notification createOnCompleted() {
return (Notification) ON_COMPLETED;
}
@SuppressWarnings("unchecked")
public static Notification createOnCompleted(Class type) {
return (Notification) ON_COMPLETED;
}
private Notification(Kind kind, T value, Throwable e) {
this.value = value;
this.throwable = e;
this.kind = kind;
}
/**
* A constructor used to represent an onNext notification.
*
* @param value
* The data passed to the onNext method.
*/
@Deprecated
public Notification(T value) {
this.value = value;
this.throwable = null;
this.kind = Kind.OnNext;
}
/**
* A constructor used to represent an onError notification.
*
* @param exception
* The exception passed to the onError notification.
* @deprecated Because type Throwable can't disambiguate the constructors if both onNext and onError are type "Throwable"
*/
@Deprecated
public Notification(Throwable exception) {
this.throwable = exception;
this.value = null;
this.kind = Kind.OnError;
}
/**
* A constructor used to represent an onCompleted notification.
*/
@Deprecated
public Notification() {
this.throwable = null;
this.value = null;
this.kind = Kind.OnCompleted;
}
/**
* Retrieves the exception associated with an onError notification.
*
* @return Throwable associated with an onError notification.
*/
public Throwable getThrowable() {
return throwable;
}
/**
* Retrieves the data associated with an onNext notification.
*
* @return The data associated with an onNext notification.
*/
public T getValue() {
return value;
}
/**
* Retrieves a value indicating whether this notification has a value.
*
* @return a value indicating whether this notification has a value.
*/
public boolean hasValue() {
return isOnNext() && value != null;
}
/**
* Retrieves a value indicating whether this notification has an exception.
*
* @return a value indicating whether this notification has an exception.
*/
public boolean hasThrowable() {
return isOnError() && throwable != null;
}
/**
* Retrieves the kind of the notification: OnNext, OnError, OnCompleted
*
* @return the kind of the notification: OnNext, OnError, OnCompleted
*/
public Kind getKind() {
return kind;
}
public boolean isOnError() {
return getKind() == Kind.OnError;
}
public boolean isOnCompleted() {
return getKind() == Kind.OnCompleted;
}
public boolean isOnNext() {
return getKind() == Kind.OnNext;
}
public void accept(Observer super T> observer) {
if (isOnNext()) {
observer.onNext(getValue());
} else if (isOnCompleted()) {
observer.onCompleted();
} else if (isOnError()) {
observer.onError(getThrowable());
}
}
public static enum Kind {
OnNext, OnError, OnCompleted
}
@Override
public String toString() {
StringBuilder str = new StringBuilder("[").append(super.toString()).append(" ").append(getKind());
if (hasValue())
str.append(" ").append(getValue());
if (hasThrowable())
str.append(" ").append(getThrowable().getMessage());
str.append("]");
return str.toString();
}
@Override
public int hashCode() {
int hash = getKind().hashCode();
if (hasValue())
hash = hash * 31 + getValue().hashCode();
if (hasThrowable())
hash = hash * 31 + getThrowable().hashCode();
return hash;
}
@Override
public boolean equals(Object obj) {
if (obj == null)
return false;
if (this == obj)
return true;
if (obj.getClass() != getClass())
return false;
Notification> notification = (Notification>) obj;
if (notification.getKind() != getKind())
return false;
if (hasValue() && !getValue().equals(notification.getValue()))
return false;
if (hasThrowable() && !getThrowable().equals(notification.getThrowable()))
return false;
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy