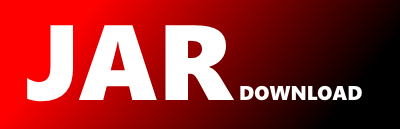
rx.operators.OperationMinMax Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rxjava-core Show documentation
Show all versions of rxjava-core Show documentation
rxjava-core developed by Netflix
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.operators;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import rx.Observable;
import rx.functions.Func1;
import rx.functions.Func2;
/**
* Returns the minimum element in an observable sequence.
*/
public class OperationMinMax {
public static > Observable min(
Observable source) {
return minMax(source, -1L);
}
public static Observable min(Observable source,
final Comparator super T> comparator) {
return minMax(source, comparator, -1L);
}
public static > Observable> minBy(
Observable source, final Func1 selector) {
return minMaxBy(source, selector, -1L);
}
public static Observable> minBy(Observable source,
final Func1 selector, final Comparator super R> comparator) {
return minMaxBy(source, selector, comparator, -1L);
}
public static > Observable max(
Observable source) {
return minMax(source, 1L);
}
public static Observable max(Observable source,
final Comparator super T> comparator) {
return minMax(source, comparator, 1L);
}
public static > Observable> maxBy(
Observable source, final Func1 selector) {
return minMaxBy(source, selector, 1L);
}
public static Observable> maxBy(Observable source,
final Func1 selector, final Comparator super R> comparator) {
return minMaxBy(source, selector, comparator, 1L);
}
private static > Observable minMax(
Observable source, final long flag) {
return source.reduce(new Func2() {
@Override
public T call(T acc, T value) {
if (flag * acc.compareTo(value) > 0) {
return acc;
}
return value;
}
});
}
private static Observable minMax(Observable source,
final Comparator super T> comparator, final long flag) {
return source.reduce(new Func2() {
@Override
public T call(T acc, T value) {
if (flag * comparator.compare(acc, value) > 0) {
return acc;
}
return value;
}
});
}
private static > Observable> minMaxBy(
Observable source, final Func1 selector, final long flag) {
return source.reduce(new ArrayList(),
new Func2, T, List>() {
@Override
public List call(List acc, T value) {
if (acc.isEmpty()) {
acc.add(value);
} else {
int compareResult = selector.call(acc.get(0))
.compareTo(selector.call(value));
if (compareResult == 0) {
acc.add(value);
} else if (flag * compareResult < 0) {
acc.clear();
acc.add(value);
}
}
return acc;
}
});
}
private static Observable> minMaxBy(Observable source,
final Func1 selector, final Comparator super R> comparator,
final long flag) {
return source.reduce(new ArrayList(),
new Func2, T, List>() {
@Override
public List call(List acc, T value) {
if (acc.isEmpty()) {
acc.add(value);
} else {
int compareResult = comparator.compare(
selector.call(acc.get(0)),
selector.call(value));
if (compareResult == 0) {
acc.add(value);
} else if (flag * compareResult < 0) {
acc.clear();
acc.add(value);
}
}
return acc;
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy