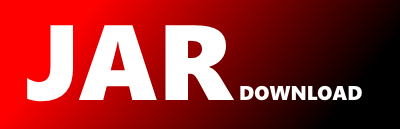
rx.operators.OperatorObserveOnBounded Maven / Gradle / Ivy
Show all versions of rxjava-core Show documentation
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.operators;
import java.util.concurrent.Semaphore;
import java.util.concurrent.atomic.AtomicLong;
import rx.Observable.Operator;
import rx.Scheduler;
import rx.Scheduler.Inner;
import rx.Subscriber;
import rx.functions.Action0;
import rx.functions.Action1;
import rx.schedulers.ImmediateScheduler;
import rx.schedulers.TestScheduler;
import rx.schedulers.TrampolineScheduler;
import rx.subscriptions.Subscriptions;
/**
* Delivers events on the specified Scheduler.
*
* This provides backpressure by blocking the incoming onNext when there is already one in the queue.
*
* This means that at any given time the max number of "onNext" in flight is 3:
* -> 1 being delivered on the Scheduler
* -> 1 in the queue waiting for the Scheduler
* -> 1 blocking on the queue waiting to deliver it
*
* I have chosen to allow 1 in the queue rather than using an Exchanger style process so that the Scheduler
* can loop and have something to do each time around to optimize for avoiding rescheduling when it
* can instead just loop. I'm avoiding having the Scheduler thread ever block as it could be an event-loop
* thus if the queue is empty it exits and next time something is added it will reschedule.
*
*
*/
public class OperatorObserveOnBounded implements Operator {
private final Scheduler scheduler;
private final int bufferSize;
/**
*
* @param scheduler
* @param bufferSize
* that will be rounded up to the next power of 2
*/
public OperatorObserveOnBounded(Scheduler scheduler, int bufferSize) {
this.scheduler = scheduler;
this.bufferSize = roundToNextPowerOfTwoIfNecessary(bufferSize);
}
public OperatorObserveOnBounded(Scheduler scheduler) {
this(scheduler, 1);
}
private static int roundToNextPowerOfTwoIfNecessary(int num) {
if ((num & -num) == num) {
return num;
} else {
int result = 1;
while (num != 0)
{
num >>= 1;
result <<= 1;
}
return result;
}
}
@Override
public Subscriber super T> call(Subscriber super T> child) {
if (scheduler instanceof ImmediateScheduler) {
// avoid overhead, execute directly
return child;
} else if (scheduler instanceof TrampolineScheduler) {
// avoid overhead, execute directly
return child;
} else if (scheduler instanceof TestScheduler) {
// this one will deadlock as it is single-threaded and won't run the scheduled
// work until it manually advances, which it won't be able to do as it will block
return child;
} else {
return new ObserveOnSubscriber(child);
}
}
private static Object NULL_SENTINEL = new Object();
private static Object COMPLETE_SENTINEL = new Object();
private static class ErrorSentinel {
final Throwable e;
ErrorSentinel(Throwable e) {
this.e = e;
}
}
/** Observe through individual queue per observer. */
private class ObserveOnSubscriber extends Subscriber {
final Subscriber super T> observer;
private volatile Scheduler.Inner recursiveScheduler;
private final InterruptibleBlockingQueue