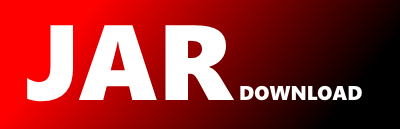
rx.internal.operators.OperatorConcat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rxjava-core Show documentation
Show all versions of rxjava-core Show documentation
rxjava-core developed by Netflix
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package rx.internal.operators;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.atomic.AtomicIntegerFieldUpdater;
import rx.Observable;
import rx.Observable.Operator;
import rx.Subscriber;
import rx.functions.Action0;
import rx.observers.SerializedSubscriber;
import rx.subscriptions.SerialSubscription;
import rx.subscriptions.Subscriptions;
/**
* Returns an Observable that emits the items emitted by two or more Observables, one after the
* other.
*
*
* @param the source and result value type
*/
public final class OperatorConcat implements Operator> {
@Override
public Subscriber super Observable extends T>> call(final Subscriber super T> child) {
final SerializedSubscriber s = new SerializedSubscriber(child);
final SerialSubscription current = new SerialSubscription();
child.add(current);
return new ConcatSubscriber(s, current);
}
static final class ConcatSubscriber extends Subscriber> {
final NotificationLite> nl = NotificationLite.instance();
private final Subscriber s;
private final SerialSubscription current;
final ConcurrentLinkedQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy