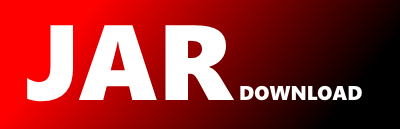
rx.observables.BlockingObservable Maven / Gradle / Ivy
Show all versions of rxjava-core Show documentation
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.observables;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.Future;
import java.util.concurrent.atomic.AtomicReference;
import rx.Observable;
import rx.Subscriber;
import rx.functions.Action1;
import rx.functions.Func1;
import rx.functions.Functions;
import rx.internal.operators.BlockingOperatorLatest;
import rx.internal.operators.BlockingOperatorMostRecent;
import rx.internal.operators.BlockingOperatorNext;
import rx.internal.operators.BlockingOperatorToFuture;
import rx.internal.operators.BlockingOperatorToIterator;
/**
* An extension of {@link Observable} that provides blocking operators.
*
* You construct a {@code BlockingObservable} from an {@code Observable} with {@link #from(Observable)} or
* {@link Observable#toBlocking()}.
*
* The documentation for this interface makes use of a form of marble diagram that has been modified to
* illustrate blocking operators. The following legend explains these marble diagrams:
*
*
*
* For more information see the
* Blocking
* Observable Operators page at the RxJava Wiki.
*
* @param
* the type of item emitted by the {@code BlockingObservable}
*/
public class BlockingObservable {
private final Observable extends T> o;
private BlockingObservable(Observable extends T> o) {
this.o = o;
}
/**
* Converts an {@link Observable} into a {@code BlockingObservable}.
*
* @param o
* the {@link Observable} you want to convert
* @return a {@code BlockingObservable} version of {@code o}
*/
public static BlockingObservable from(final Observable extends T> o) {
return new BlockingObservable(o);
}
/**
* Invokes a method on each item emitted by this {@code BlockingObservable} and blocks until the Observable
* completes.
*
* Note: This will block even if the underlying Observable is asynchronous.
*
* This is similar to {@link Observable#subscribe(Subscriber)}, but it blocks. Because it blocks it does not
* need the {@link Subscriber#onCompleted()} or {@link Subscriber#onError(Throwable)} methods.
*
*
*
* @param onNext
* the {@link Action1} to invoke for each item emitted by the {@code BlockingObservable}
* @throws RuntimeException
* if an error occurs
* @see RxJava Wiki: forEach()
*/
public void forEach(final Action1 super T> onNext) {
final CountDownLatch latch = new CountDownLatch(1);
final AtomicReference exceptionFromOnError = new AtomicReference();
/*
* Use 'subscribe' instead of 'unsafeSubscribe' for Rx contract behavior
* as this is the final subscribe in the chain.
*/
o.subscribe(new Subscriber() {
@Override
public void onCompleted() {
latch.countDown();
}
@Override
public void onError(Throwable e) {
/*
* If we receive an onError event we set the reference on the
* outer thread so we can git it and throw after the
* latch.await().
*
* We do this instead of throwing directly since this may be on
* a different thread and the latch is still waiting.
*/
exceptionFromOnError.set(e);
latch.countDown();
}
@Override
public void onNext(T args) {
onNext.call(args);
}
});
// block until the subscription completes and then return
try {
latch.await();
} catch (InterruptedException e) {
// set the interrupted flag again so callers can still get it
// for more information see https://github.com/Netflix/RxJava/pull/147#issuecomment-13624780
Thread.currentThread().interrupt();
// using Runtime so it is not checked
throw new RuntimeException("Interrupted while waiting for subscription to complete.", e);
}
if (exceptionFromOnError.get() != null) {
if (exceptionFromOnError.get() instanceof RuntimeException) {
throw (RuntimeException) exceptionFromOnError.get();
} else {
throw new RuntimeException(exceptionFromOnError.get());
}
}
}
/**
* Returns an {@link Iterator} that iterates over all items emitted by this {@code BlockingObservable}.
*
*
*
* @return an {@link Iterator} that can iterate over the items emitted by this {@code BlockingObservable}
* @see RxJava Wiki: getIterator()
*/
public Iterator getIterator() {
return BlockingOperatorToIterator.toIterator(o);
}
/**
* Returns the first item emitted by this {@code BlockingObservable}, or throws
* {@code NoSuchElementException} if it emits no items.
*
* @return the first item emitted by this {@code BlockingObservable}
* @throws NoSuchElementException
* if this {@code BlockingObservable} emits no items
* @see RxJava Wiki: first()
* @see MSDN: Observable.First
*/
public T first() {
return from(o.first()).single();
}
/**
* Returns the first item emitted by this {@code BlockingObservable} that matches a predicate, or throws
* {@code NoSuchElementException} if it emits no such item.
*
* @param predicate
* a predicate function to evaluate items emitted by this {@code BlockingObservable}
* @return the first item emitted by this {@code BlockingObservable} that matches the predicate
* @throws NoSuchElementException
* if this {@code BlockingObservable} emits no such items
* @see RxJava Wiki: first()
* @see MSDN: Observable.First
*/
public T first(Func1 super T, Boolean> predicate) {
return from(o.first(predicate)).single();
}
/**
* Returns the first item emitted by this {@code BlockingObservable}, or a default value if it emits no
* items.
*
* @param defaultValue
* a default value to return if this {@code BlockingObservable} emits no items
* @return the first item emitted by this {@code BlockingObservable}, or the default value if it emits no
* items
* @see RxJava Wiki: firstOrDefault()
* @see MSDN: Observable.FirstOrDefault
*/
public T firstOrDefault(T defaultValue) {
return from(o.take(1)).singleOrDefault(defaultValue);
}
/**
* Returns the first item emitted by this {@code BlockingObservable} that matches a predicate, or a default
* value if it emits no such items.
*
* @param defaultValue
* a default value to return if this {@code BlockingObservable} emits no matching items
* @param predicate
* a predicate function to evaluate items emitted by this {@code BlockingObservable}
* @return the first item emitted by this {@code BlockingObservable} that matches the predicate, or the
* default value if this {@code BlockingObservable} emits no matching items
* @see RxJava Wiki: firstOrDefault()
* @see MSDN: Observable.FirstOrDefault
*/
public T firstOrDefault(T defaultValue, Func1 super T, Boolean> predicate) {
return from(o.filter(predicate)).firstOrDefault(defaultValue);
}
/**
* Returns the last item emitted by this {@code BlockingObservable}, or throws
* {@code NoSuchElementException} if this {@code BlockingObservable} emits no items.
*
*
*
* @return the last item emitted by this {@code BlockingObservable}
* @throws NoSuchElementException
* if this {@code BlockingObservable} emits no items
* @see RxJava Wiki: last()
* @see MSDN: Observable.Last
*/
public T last() {
return from(o.last()).single();
}
/**
* Returns the last item emitted by this {@code BlockingObservable} that matches a predicate, or throws
* {@code NoSuchElementException} if it emits no such items.
*
*
*
* @param predicate
* a predicate function to evaluate items emitted by the {@code BlockingObservable}
* @return the last item emitted by the {@code BlockingObservable} that matches the predicate
* @throws NoSuchElementException
* if this {@code BlockingObservable} emits no items
* @see RxJava Wiki: last()
* @see MSDN: Observable.Last
*/
public T last(final Func1 super T, Boolean> predicate) {
return from(o.last(predicate)).single();
}
/**
* Returns the last item emitted by this {@code BlockingObservable}, or a default value if it emits no
* items.
*
*
*
* @param defaultValue
* a default value to return if this {@code BlockingObservable} emits no items
* @return the last item emitted by the {@code BlockingObservable}, or the default value if it emits no
* items
* @see RxJava Wiki: lastOrDefault()
* @see MSDN: Observable.LastOrDefault
*/
public T lastOrDefault(T defaultValue) {
return from(o.takeLast(1)).singleOrDefault(defaultValue);
}
/**
* Returns the last item emitted by this {@code BlockingObservable} that matches a predicate, or a default
* value if it emits no such items.
*
*
*
* @param defaultValue
* a default value to return if this {@code BlockingObservable} emits no matching items
* @param predicate
* a predicate function to evaluate items emitted by this {@code BlockingObservable}
* @return the last item emitted by this {@code BlockingObservable} that matches the predicate, or the
* default value if it emits no matching items
* @see RxJava Wiki: lastOrDefault()
* @see MSDN: Observable.LastOrDefault
*/
public T lastOrDefault(T defaultValue, Func1 super T, Boolean> predicate) {
return from(o.filter(predicate)).lastOrDefault(defaultValue);
}
/**
* Returns an {@link Iterable} that always returns the item most recently emitted by this
* {@code BlockingObservable}.
*
*
*
* @param initialValue
* the initial value that the {@link Iterable} sequence will yield if this
* {@code BlockingObservable} has not yet emitted an item
* @return an {@link Iterable} that on each iteration returns the item that this {@code BlockingObservable}
* has most recently emitted
* @see RxJava wiki: mostRecent()
* @see MSDN: Observable.MostRecent
*/
public Iterable mostRecent(T initialValue) {
return BlockingOperatorMostRecent.mostRecent(o, initialValue);
}
/**
* Returns an {@link Iterable} that blocks until this {@code BlockingObservable} emits another item, then
* returns that item.
*
*
*
* @return an {@link Iterable} that blocks upon each iteration until this {@code BlockingObservable} emits
* a new item, whereupon the Iterable returns that item
* @see RxJava Wiki: next()
* @see MSDN: Observable.Next
*/
public Iterable next() {
return BlockingOperatorNext.next(o);
}
/**
* Returns an {@link Iterable} that returns the latest item emitted by this {@code BlockingObservable},
* waiting if necessary for one to become available.
*
* If this {@code BlockingObservable} produces items faster than {@code Iterator.next} takes them,
* {@code onNext} events might be skipped, but {@code onError} or {@code onCompleted} events are not.
*
* Note also that an {@code onNext} directly followed by {@code onCompleted} might hide the {@code onNext}
* event.
*
* @return an Iterable that always returns the latest item emitted by this {@code BlockingObservable}
* @see RxJava wiki: latest()
* @see MSDN: Observable.Latest
*/
public Iterable latest() {
return BlockingOperatorLatest.latest(o);
}
/**
* If this {@code BlockingObservable} completes after emitting a single item, return that item, otherwise
* throw a {@code NoSuchElementException}.
*
*
*
* @return the single item emitted by this {@code BlockingObservable}
* @see RxJava Wiki: single()
* @see MSDN: Observable.Single
*/
public T single() {
return from(o.single()).toIterable().iterator().next();
}
/**
* If this {@code BlockingObservable} completes after emitting a single item that matches a given predicate,
* return that item, otherwise throw a {@code NoSuchElementException}.
*
*
*
* @param predicate
* a predicate function to evaluate items emitted by this {@link BlockingObservable}
* @return the single item emitted by this {@code BlockingObservable} that matches the predicate
* @see RxJava Wiki: single()
* @see MSDN: Observable.Single
*/
public T single(Func1 super T, Boolean> predicate) {
return from(o.single(predicate)).toIterable().iterator().next();
}
/**
* If this {@code BlockingObservable} completes after emitting a single item, return that item; if it emits
* more than one item, throw an {@code IllegalArgumentException}; if it emits no items, return a default
* value.
*
*
*
* @param defaultValue
* a default value to return if this {@code BlockingObservable} emits no items
* @return the single item emitted by this {@code BlockingObservable}, or the default value if it emits no
* items
* @see RxJava Wiki: singleOrDefault()
* @see MSDN: Observable.SingleOrDefault
*/
public T singleOrDefault(T defaultValue) {
return from(o.map(Functions.identity()).singleOrDefault(defaultValue)).single();
}
/**
* If this {@code BlockingObservable} completes after emitting a single item that matches a predicate,
* return that item; if it emits more than one such item, throw an {@code IllegalArgumentException}; if it
* emits no items, return a default value.
*
*
*
* @param defaultValue
* a default value to return if this {@code BlockingObservable} emits no matching items
* @param predicate
* a predicate function to evaluate items emitted by this {@code BlockingObservable}
* @return the single item emitted by the {@code BlockingObservable} that matches the predicate, or the
* default value if no such items are emitted
* @see RxJava Wiki: singleOrDefault()
* @see MSDN: Observable.SingleOrDefault
*/
public T singleOrDefault(T defaultValue, Func1 super T, Boolean> predicate) {
return from(o.filter(predicate)).singleOrDefault(defaultValue);
}
/**
* Returns a {@link Future} representing the single value emitted by this {@code BlockingObservable}.
*
* If {@link BlockingObservable} emits more than one item, {@link java.util.concurrent.Future} will receive an
* {@link java.lang.IllegalArgumentException}. If {@link BlockingObservable} is empty, {@link java.util.concurrent.Future}
* will receive an {@link java.util.NoSuchElementException}.
*
* If the {@code BlockingObservable} may emit more than one item, use {@code Observable.toList().toBlocking().toFuture()}.
*
*
*
* @return a {@link Future} that expects a single item to be emitted by this {@code BlockingObservable}
* @see RxJava Wiki: toFuture()
*/
public Future toFuture() {
return BlockingOperatorToFuture.toFuture(o);
}
/**
* Converts this {@code BlockingObservable} into an {@link Iterable}.
*
*
*
* @return an {@link Iterable} version of this {@code BlockingObservable}
* @see RxJava Wiki: toIterable()
*/
public Iterable toIterable() {
return new Iterable() {
@Override
public Iterator iterator() {
return getIterator();
}
};
}
}