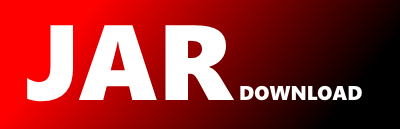
rx.observers.TestObserver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rxjava-core Show documentation
Show all versions of rxjava-core Show documentation
rxjava-core developed by Netflix
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.observers;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import rx.Notification;
import rx.Observer;
/**
* Observer usable for unit testing to perform assertions, inspect received events or wrap a mocked Observer.
*/
public class TestObserver implements Observer {
private final Observer delegate;
private final ArrayList onNextEvents = new ArrayList();
private final ArrayList onErrorEvents = new ArrayList();
private final ArrayList> onCompletedEvents = new ArrayList>();
public TestObserver(Observer delegate) {
this.delegate = delegate;
}
public TestObserver() {
this.delegate = Observers.empty();
}
@Override
public void onCompleted() {
onCompletedEvents.add(Notification. createOnCompleted());
delegate.onCompleted();
}
/**
* Get the {@link Notification}s representing each time this observer was notified of sequence completion
* via {@link #onCompleted}, as a {@link List}.
*
* @return a list of Notifications representing calls to this observer's {@link #onCompleted} method
*/
public List> getOnCompletedEvents() {
return Collections.unmodifiableList(onCompletedEvents);
}
@Override
public void onError(Throwable e) {
onErrorEvents.add(e);
delegate.onError(e);
}
/**
* Get the {@link Throwable}s this observer was notified of via {@link #onError} as a {@link List}.
*
* @return a list of Throwables passed to this observer's {@link #onError} method
*/
public List getOnErrorEvents() {
return Collections.unmodifiableList(onErrorEvents);
}
@Override
public void onNext(T t) {
onNextEvents.add(t);
delegate.onNext(t);
}
/**
* Get the sequence of items observed by this observer, as an ordered {@link List}.
*
* @return a list of items observed by this observer, in the order in which they were observed
*/
public List getOnNextEvents() {
return Collections.unmodifiableList(onNextEvents);
}
/**
* Get a list containing all of the items and notifications received by this observer, where the items
* will be given as-is, any error notifications will be represented by their {@code Throwable}s, and any
* sequence-complete notifications will be represented by their {@code Notification} objects.
*
* @return a {@link List} containing one item for each item or notification received by this observer, in
* the order in which they were observed or received
*/
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy