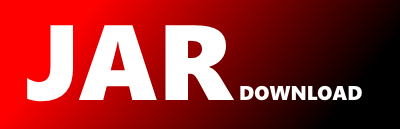
rx.Observable Maven / Gradle / Ivy
Show all versions of rxjava-core Show documentation
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package rx;
import static rx.functions.Functions.alwaysFalse;
import java.util.*;
import java.util.concurrent.*;
import rx.exceptions.*;
import rx.functions.*;
import rx.internal.operators.*;
import rx.internal.util.ScalarSynchronousObservable;
import rx.observables.*;
import rx.observers.SafeSubscriber;
import rx.plugins.*;
import rx.schedulers.*;
import rx.subjects.*;
import rx.subscriptions.Subscriptions;
/**
* The Observable class that implements the Reactive Pattern.
*
* This class provides methods for subscribing to the Observable as well as delegate methods to the various
* Observers.
*
* The documentation for this class makes use of marble diagrams. The following legend explains these diagrams:
*
*
*
* For more information see the RxJava wiki
*
* @param
* the type of the items emitted by the Observable
*/
public class Observable {
final OnSubscribe onSubscribe;
/**
* Creates an Observable with a Function to execute when it is subscribed to.
*
* Note: Use {@link #create(OnSubscribe)} to create an Observable, instead of this constructor,
* unless you specifically have a need for inheritance.
*
* @param f
* {@link OnSubscribe} to be executed when {@link #subscribe(Subscriber)} is called
*/
protected Observable(OnSubscribe f) {
this.onSubscribe = f;
}
private static final RxJavaObservableExecutionHook hook = RxJavaPlugins.getInstance().getObservableExecutionHook();
/**
* Returns an Observable that will execute the specified function when a {@link Subscriber} subscribes to
* it.
*
*
*
* Write the function you pass to {@code create} so that it behaves as an Observable: It should invoke the
* Subscriber's {@link Subscriber#onNext onNext}, {@link Subscriber#onError onError}, and
* {@link Subscriber#onCompleted onCompleted} methods appropriately.
*
* A well-formed Observable must invoke either the Subscriber's {@code onCompleted} method exactly once or
* its {@code onError} method exactly once.
*
* See Rx Design Guidelines (PDF) for detailed
* information.
*
* - Scheduler:
* - {@code create} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the type of the items that this Observable emits
* @param f
* a function that accepts an {@code Subscriber}, and invokes its {@code onNext},
* {@code onError}, and {@code onCompleted} methods as appropriate
* @return an Observable that, when a {@link Subscriber} subscribes to it, will execute the specified
* function
* @see RxJava wiki: create
* @see MSDN: Observable.Create
*/
public final static Observable create(OnSubscribe f) {
return new Observable(hook.onCreate(f));
}
/**
* Invoked when Obserable.subscribe is called.
*/
public static interface OnSubscribe extends Action1> {
// cover for generics insanity
}
/**
* Operator function for lifting into an Observable.
*/
public interface Operator extends Func1, Subscriber super T>> {
// cover for generics insanity
}
/**
* @deprecated use {@link #create(OnSubscribe)}
*/
@Deprecated
public final static Observable create(final OnSubscribeFunc f) {
return new Observable(new OnSubscribe() {
@Override
public void call(Subscriber super T> observer) {
Subscription s = f.onSubscribe(observer);
if (s != null && s != observer) {
observer.add(s);
}
}
});
}
/**
* @deprecated use {@link OnSubscribe}
*/
@Deprecated
public static interface OnSubscribeFunc extends Function {
public Subscription onSubscribe(Observer super T> op);
}
/**
* Lifts a function to the current Observable and returns a new Observable that when subscribed to will pass
* the values of the current Observable through the Operator function.
*
* In other words, this allows chaining Observers together on an Observable for acting on the values within
* the Observable.
*
{@code
* observable.map(...).filter(...).take(5).lift(new OperatorA()).lift(new OperatorB(...)).subscribe()
* }
*
* If the operator you are creating is designed to act on the individual items emitted by a source
* Observable, use {@code lift}. If your operator is designed to transform the source Observable as a whole
* (for instance, by applying a particular set of existing RxJava operators to it) use {@link #compose}.
*
* - Scheduler:
* - {@code lift} does not operate by default on a particular {@link Scheduler}.
*
*
* @param lift the Operator that implements the Observable-operating function to be applied to the source
* Observable
* @return an Observable that is the result of applying the lifted Operator to the source Observable
* @see RxJava wiki: Implementing Your Own Operators
* @since 0.17
*/
public final Observable lift(final Operator extends R, ? super T> lift) {
return new Observable(new OnSubscribe() {
@Override
public void call(Subscriber super R> o) {
try {
Subscriber super T> st = hook.onLift(lift).call(o);
// new Subscriber created and being subscribed with so 'onStart' it
st.onStart();
onSubscribe.call(st);
} catch (Throwable e) {
// localized capture of errors rather than it skipping all operators
// and ending up in the try/catch of the subscribe method which then
// prevents onErrorResumeNext and other similar approaches to error handling
if (e instanceof OnErrorNotImplementedException) {
throw (OnErrorNotImplementedException) e;
}
o.onError(e);
}
}
});
}
/**
* Transform an Observable by applying a particular Transformer function to it.
*
* This method operates on the Observable itself whereas {@link #lift} operates on the Observable's
* Subscribers or Observers.
*
* If the operator you are creating is designed to act on the individual items emitted by a source
* Observable, use {@link #lift}. If your operator is designed to transform the source Observable as a whole
* (for instance, by applying a particular set of existing RxJava operators to it) use {@code compose}.
*
* - Scheduler:
* - {@code compose} does not operate by default on a particular {@link Scheduler}.
*
*
* @param transformer implements the function that transforms the source Observable
* @return the source Observable, transformed by the transformer function
* @see RxJava wiki: Implementing Your Own Operators
* @since 0.20
*/
@SuppressWarnings("unchecked")
public Observable compose(Transformer super T, ? extends R> transformer) {
// Casting to Observable is type-safe because we know Observable is covariant.
return (Observable) transformer.call(this);
}
/**
* Transformer function used by {@link #compose}.
* @warn more complete description needed
* @since 0.20
*/
public static interface Transformer extends Func1, Observable extends R>> {
// cover for generics insanity
}
/* *********************************************************************************************************
* Operators Below Here
* *********************************************************************************************************
*/
/**
* Mirrors the one Observable in an Iterable of several Observables that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param sources
* an Iterable of Observable sources competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Iterable extends Observable extends T>> sources) {
return create(OnSubscribeAmb.amb(sources));
}
/**
* Given two Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2) {
return create(OnSubscribeAmb.amb(o1, o2));
}
/**
* Given three Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3) {
return create(OnSubscribeAmb.amb(o1, o2, o3));
}
/**
* Given four Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @param o4
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3, Observable extends T> o4) {
return create(OnSubscribeAmb.amb(o1, o2, o3, o4));
}
/**
* Given five Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @param o4
* an Observable competing to react first
* @param o5
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3, Observable extends T> o4, Observable extends T> o5) {
return create(OnSubscribeAmb.amb(o1, o2, o3, o4, o5));
}
/**
* Given six Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @param o4
* an Observable competing to react first
* @param o5
* an Observable competing to react first
* @param o6
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3, Observable extends T> o4, Observable extends T> o5, Observable extends T> o6) {
return create(OnSubscribeAmb.amb(o1, o2, o3, o4, o5, o6));
}
/**
* Given seven Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @param o4
* an Observable competing to react first
* @param o5
* an Observable competing to react first
* @param o6
* an Observable competing to react first
* @param o7
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3, Observable extends T> o4, Observable extends T> o5, Observable extends T> o6, Observable extends T> o7) {
return create(OnSubscribeAmb.amb(o1, o2, o3, o4, o5, o6, o7));
}
/**
* Given eight Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @param o4
* an Observable competing to react first
* @param o5
* an Observable competing to react first
* @param o6
* an Observable competing to react first
* @param o7
* an Observable competing to react first
* @param o8
* an observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3, Observable extends T> o4, Observable extends T> o5, Observable extends T> o6, Observable extends T> o7, Observable extends T> o8) {
return create(OnSubscribeAmb.amb(o1, o2, o3, o4, o5, o6, o7, o8));
}
/**
* Given nine Observables, mirrors the one that first emits an item.
*
*
*
* - Scheduler:
* - {@code amb} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* an Observable competing to react first
* @param o2
* an Observable competing to react first
* @param o3
* an Observable competing to react first
* @param o4
* an Observable competing to react first
* @param o5
* an Observable competing to react first
* @param o6
* an Observable competing to react first
* @param o7
* an Observable competing to react first
* @param o8
* an Observable competing to react first
* @param o9
* an Observable competing to react first
* @return an Observable that emits the same sequence of items as whichever of the source Observables first
* emitted an item
* @see RxJava wiki: amb
* @see MSDN: Observable.Amb
*/
public final static Observable amb(Observable extends T> o1, Observable extends T> o2, Observable extends T> o3, Observable extends T> o4, Observable extends T> o5, Observable extends T> o6, Observable extends T> o7, Observable extends T> o8, Observable extends T> o9) {
return create(OnSubscribeAmb.amb(o1, o2, o3, o4, o5, o6, o7, o8, o9));
}
/**
* Combines two source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from either of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Func2 super T1, ? super T2, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2), Functions.fromFunc(combineFunction));
}
/**
* Combines three source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Func3 super T1, ? super T2, ? super T3, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3), Functions.fromFunc(combineFunction));
}
/**
* Combines four source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param o4
* the fourth source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Observable extends T4> o4,
Func4 super T1, ? super T2, ? super T3, ? super T4, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3, o4), Functions.fromFunc(combineFunction));
}
/**
* Combines five source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param o4
* the fourth source Observable
* @param o5
* the fifth source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Observable extends T4> o4, Observable extends T5> o5,
Func5 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3, o4, o5), Functions.fromFunc(combineFunction));
}
/**
* Combines six source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param o4
* the fourth source Observable
* @param o5
* the fifth source Observable
* @param o6
* the sixth source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Observable extends T4> o4, Observable extends T5> o5, Observable extends T6> o6,
Func6 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3, o4, o5, o6), Functions.fromFunc(combineFunction));
}
/**
* Combines seven source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param o4
* the fourth source Observable
* @param o5
* the fifth source Observable
* @param o6
* the sixth source Observable
* @param o7
* the seventh source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Observable extends T4> o4, Observable extends T5> o5, Observable extends T6> o6, Observable extends T7> o7,
Func7 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3, o4, o5, o6, o7), Functions.fromFunc(combineFunction));
}
/**
* Combines eight source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param o4
* the fourth source Observable
* @param o5
* the fifth source Observable
* @param o6
* the sixth source Observable
* @param o7
* the seventh source Observable
* @param o8
* the eighth source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Observable extends T4> o4, Observable extends T5> o5, Observable extends T6> o6, Observable extends T7> o7, Observable extends T8> o8,
Func8 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3, o4, o5, o6, o7, o8), Functions.fromFunc(combineFunction));
}
/**
* Combines nine source Observables by emitting an item that aggregates the latest values of each of the
* source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
*
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param o1
* the first source Observable
* @param o2
* the second source Observable
* @param o3
* the third source Observable
* @param o4
* the fourth source Observable
* @param o5
* the fifth source Observable
* @param o6
* the sixth source Observable
* @param o7
* the seventh source Observable
* @param o8
* the eighth source Observable
* @param o9
* the ninth source Observable
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
@SuppressWarnings("unchecked")
public static final Observable combineLatest(Observable extends T1> o1, Observable extends T2> o2, Observable extends T3> o3, Observable extends T4> o4, Observable extends T5> o5, Observable extends T6> o6, Observable extends T7> o7, Observable extends T8> o8,
Observable extends T9> o9,
Func9 super T1, ? super T2, ? super T3, ? super T4, ? super T5, ? super T6, ? super T7, ? super T8, ? super T9, ? extends R> combineFunction) {
return combineLatest(Arrays.asList(o1, o2, o3, o4, o5, o6, o7, o8, o9), Functions.fromFunc(combineFunction));
}
/**
* Combines a list of source Observables by emitting an item that aggregates the latest values of each of
* the source Observables each time an item is received from any of the source Observables, where this
* aggregation is defined by a specified function.
*
* - Scheduler:
* - {@code combineLatest} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the common base type of source values
* @param
* the result type
* @param sources
* the list of source Observables
* @param combineFunction
* the aggregation function used to combine the items emitted by the source Observables
* @return an Observable that emits items that are the result of combining the items emitted by the source
* Observables by means of the given aggregation function
* @see RxJava wiki: combineLatest
* @see MSDN: Observable.CombineLatest
*/
public static final Observable combineLatest(List extends Observable extends T>> sources, FuncN extends R> combineFunction) {
return create(new OnSubscribeCombineLatest(sources, combineFunction));
}
/**
* Returns an Observable that emits the items emitted by each of the Observables emitted by the source
* Observable, one after the other, without interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param observables
* an Observable that emits Observables
* @return an Observable that emits items all of the items emitted by the Observables emitted by
* {@code observables}, one after the other, without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends Observable extends T>> observables) {
return observables.lift(new OperatorConcat());
}
/**
* Returns an Observable that emits the items emitted by two Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @return an Observable that emits items emitted by the two source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2) {
return concat(just(t1, t2));
}
/**
* Returns an Observable that emits the items emitted by three Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @return an Observable that emits items emitted by the three source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3) {
return concat(just(t1, t2, t3));
}
/**
* Returns an Observable that emits the items emitted by four Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @param t4
* an Observable to be concatenated
* @return an Observable that emits items emitted by the four source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4) {
return concat(just(t1, t2, t3, t4));
}
/**
* Returns an Observable that emits the items emitted by five Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @param t4
* an Observable to be concatenated
* @param t5
* an Observable to be concatenated
* @return an Observable that emits items emitted by the five source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5) {
return concat(just(t1, t2, t3, t4, t5));
}
/**
* Returns an Observable that emits the items emitted by six Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @param t4
* an Observable to be concatenated
* @param t5
* an Observable to be concatenated
* @param t6
* an Observable to be concatenated
* @return an Observable that emits items emitted by the six source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6) {
return concat(just(t1, t2, t3, t4, t5, t6));
}
/**
* Returns an Observable that emits the items emitted by seven Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @param t4
* an Observable to be concatenated
* @param t5
* an Observable to be concatenated
* @param t6
* an Observable to be concatenated
* @param t7
* an Observable to be concatenated
* @return an Observable that emits items emitted by the seven source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7) {
return concat(just(t1, t2, t3, t4, t5, t6, t7));
}
/**
* Returns an Observable that emits the items emitted by eight Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @param t4
* an Observable to be concatenated
* @param t5
* an Observable to be concatenated
* @param t6
* an Observable to be concatenated
* @param t7
* an Observable to be concatenated
* @param t8
* an Observable to be concatenated
* @return an Observable that emits items emitted by the eight source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7, Observable extends T> t8) {
return concat(just(t1, t2, t3, t4, t5, t6, t7, t8));
}
/**
* Returns an Observable that emits the items emitted by nine Observables, one after the other, without
* interleaving them.
*
*
*
* - Scheduler:
* - {@code concat} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be concatenated
* @param t2
* an Observable to be concatenated
* @param t3
* an Observable to be concatenated
* @param t4
* an Observable to be concatenated
* @param t5
* an Observable to be concatenated
* @param t6
* an Observable to be concatenated
* @param t7
* an Observable to be concatenated
* @param t8
* an Observable to be concatenated
* @param t9
* an Observable to be concatenated
* @return an Observable that emits items emitted by the nine source Observables, one after the other,
* without interleaving them
* @see RxJava wiki: concat
* @see MSDN: Observable.Concat
*/
public final static Observable concat(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7, Observable extends T> t8, Observable extends T> t9) {
return concat(just(t1, t2, t3, t4, t5, t6, t7, t8, t9));
}
/**
* Returns an Observable that calls an Observable factory to create an Observable for each new Observer
* that subscribes. That is, for each subscriber, the actual Observable that subscriber observes is
* determined by the factory function.
*
*
*
* The defer Observer allows you to defer or delay emitting items from an Observable until such time as an
* Observer subscribes to the Observable. This allows an {@link Observer} to easily obtain updates or a
* refreshed version of the sequence.
*
* - Scheduler:
* - {@code defer} does not operate by default on a particular {@link Scheduler}.
*
*
* @param observableFactory
* the Observable factory function to invoke for each {@link Observer} that subscribes to the
* resulting Observable
* @param
* the type of the items emitted by the Observable
* @return an Observable whose {@link Observer}s' subscriptions trigger an invocation of the given
* Observable factory function
* @see RxJava wiki: defer
* @see MSDN: Observable.Defer
*/
public final static Observable defer(Func0> observableFactory) {
return create(new OnSubscribeDefer(observableFactory));
}
/**
* Returns an Observable that emits no items to the {@link Observer} and immediately invokes its
* {@link Observer#onCompleted onCompleted} method.
*
*
*
* - Scheduler:
* - {@code empty} does not operate by default on a particular {@link Scheduler}.
*
*
* @param
* the type of the items (ostensibly) emitted by the Observable
* @return an Observable that emits no items to the {@link Observer} but immediately invokes the
* {@link Observer}'s {@link Observer#onCompleted() onCompleted} method
* @see RxJava wiki: empty
* @see MSDN: Observable.Empty
*/
public final static Observable empty() {
return from(new ArrayList());
}
/**
* Returns an Observable that emits no items to the {@link Observer} and immediately invokes its
* {@link Observer#onCompleted onCompleted} method on the specified Scheduler.
*
*
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param scheduler
* the Scheduler to use to call the {@link Observer#onCompleted onCompleted} method
* @param
* the type of the items (ostensibly) emitted by the Observable
* @return an Observable that emits no items to the {@link Observer} but immediately invokes the
* {@link Observer}'s {@link Observer#onCompleted() onCompleted} method with the specified
* {@code scheduler}
* @see RxJava wiki: empty
* @see MSDN: Observable.Empty(IScheduler)
* @deprecated use {@link #subscribeOn} to schedule
*/
@Deprecated
public final static Observable empty(Scheduler scheduler) {
return Observable. empty().subscribeOn(scheduler);
}
/**
* Returns an Observable that invokes an {@link Observer}'s {@link Observer#onError onError} method when the
* Observer subscribes to it.
*
*
*
* - Scheduler:
* - {@code error} does not operate by default on a particular {@link Scheduler}.
*
*
* @param exception
* the particular Throwable to pass to {@link Observer#onError onError}
* @param
* the type of the items (ostensibly) emitted by the Observable
* @return an Observable that invokes the {@link Observer}'s {@link Observer#onError onError} method when
* the Observer subscribes to it
* @see RxJava wiki: error
* @see MSDN: Observable.Throw
*/
public final static Observable error(Throwable exception) {
return new ThrowObservable(exception);
}
/**
* Returns an Observable that invokes an {@link Observer}'s {@link Observer#onError onError} method on the
* specified Scheduler.
*
*
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param exception
* the particular Throwable to pass to {@link Observer#onError onError}
* @param scheduler
* the Scheduler on which to call {@link Observer#onError onError}
* @param
* the type of the items (ostensibly) emitted by the Observable
* @return an Observable that invokes the {@link Observer}'s {@link Observer#onError onError} method, on
* the specified Scheduler
* @see RxJava wiki: error
* @see MSDN: Observable.Throw
* @deprecated use {@link #subscribeOn} to schedule
*/
@Deprecated
public final static Observable error(Throwable exception, Scheduler scheduler) {
return Observable. error(exception).subscribeOn(scheduler);
}
/**
* Converts a {@link Future} into an Observable.
*
*
*
* You can convert any object that supports the {@link Future} interface into an Observable that emits the
* return value of the {@link Future#get} method of that object, by passing the object into the {@code from}
* method.
*
* Important note: This Observable is blocking; you cannot unsubscribe from it.
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param future
* the source {@link Future}
* @param
* the type of object that the {@link Future} returns, and also the type of item to be emitted by
* the resulting Observable
* @return an Observable that emits the item from the source {@link Future}
* @see RxJava wiki: from
*/
public final static Observable from(Future extends T> future) {
return create(OnSubscribeToObservableFuture.toObservableFuture(future));
}
/**
* Converts a {@link Future} into an Observable, with a timeout on the Future.
*
*
*
* You can convert any object that supports the {@link Future} interface into an Observable that emits the
* return value of the {@link Future#get} method of that object, by passing the object into the {@code from}
* method.
*
* Important note: This Observable is blocking; you cannot unsubscribe from it.
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param future
* the source {@link Future}
* @param timeout
* the maximum time to wait before calling {@code get}
* @param unit
* the {@link TimeUnit} of the {@code timeout} argument
* @param
* the type of object that the {@link Future} returns, and also the type of item to be emitted by
* the resulting Observable
* @return an Observable that emits the item from the source {@link Future}
* @see RxJava wiki: from
*/
public final static Observable from(Future extends T> future, long timeout, TimeUnit unit) {
return create(OnSubscribeToObservableFuture.toObservableFuture(future, timeout, unit));
}
/**
* Converts a {@link Future}, operating on a specified {@link Scheduler}, into an Observable.
*
*
*
* You can convert any object that supports the {@link Future} interface into an Observable that emits the
* return value of the {@link Future#get} method of that object, by passing the object into the {@code from}
* method.
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param future
* the source {@link Future}
* @param scheduler
* the {@link Scheduler} to wait for the Future on. Use a Scheduler such as
* {@link Schedulers#io()} that can block and wait on the Future
* @param
* the type of object that the {@link Future} returns, and also the type of item to be emitted by
* the resulting Observable
* @return an Observable that emits the item from the source {@link Future}
* @see RxJava wiki: from
*/
public final static Observable from(Future extends T> future, Scheduler scheduler) {
// TODO in a future revision the Scheduler will become important because we'll start polling instead of blocking on the Future
return create(OnSubscribeToObservableFuture.toObservableFuture(future)).subscribeOn(scheduler);
}
/**
* Converts an {@link Iterable} sequence into an Observable that emits the items in the sequence.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param iterable
* the source {@link Iterable} sequence
* @param
* the type of items in the {@link Iterable} sequence and the type of items to be emitted by the
* resulting Observable
* @return an Observable that emits each item in the source {@link Iterable} sequence
* @see RxJava wiki: from
* @see MSDN: Observable.ToObservable
*/
public final static Observable from(Iterable extends T> iterable) {
return create(new OnSubscribeFromIterable(iterable));
}
/**
* Converts an {@link Iterable} sequence into an Observable that operates on the specified Scheduler,
* emitting each item from the sequence.
*
*
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param iterable
* the source {@link Iterable} sequence
* @param scheduler
* the Scheduler on which the Observable is to emit the items of the Iterable
* @param
* the type of items in the {@link Iterable} sequence and the type of items to be emitted by the
* resulting Observable
* @return an Observable that emits each item in the source {@link Iterable} sequence, on the specified
* Scheduler
* @see RxJava wiki: from
* @see MSDN: Observable.ToObservable
* @deprecated use {@link #subscribeOn} to schedule the work
*/
@Deprecated
public final static Observable from(Iterable extends T> iterable, Scheduler scheduler) {
return from(iterable).subscribeOn(scheduler);
}
/**
* Converts an item into an Observable that emits that item.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* the item
* @param
* the type of the item
* @return an Observable that emits the item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
public final static Observable from(T t1) {
return just(t1);
}
/**
* Converts two items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2) {
return from(Arrays.asList(t1, t2));
}
/**
* Converts three items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3) {
return from(Arrays.asList(t1, t2, t3));
}
/**
* Converts four items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4) {
return from(Arrays.asList(t1, t2, t3, t4));
}
/**
* Converts five items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4, T t5) {
return from(Arrays.asList(t1, t2, t3, t4, t5));
}
/**
* Converts six items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4, T t5, T t6) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6));
}
/**
* Converts seven items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4, T t5, T t6, T t7) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7));
}
/**
* Converts eight items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param t8
* eighth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4, T t5, T t6, T t7, T t8) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8));
}
/**
* Converts nine items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param t8
* eighth item
* @param t9
* ninth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4, T t5, T t6, T t7, T t8, T t9) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8, t9));
}
/**
* Converts ten items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param t8
* eighth item
* @param t9
* ninth item
* @param t10
* tenth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: from
* @deprecated use {@link #just} instead
*/
@Deprecated
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable from(T t1, T t2, T t3, T t4, T t5, T t6, T t7, T t8, T t9, T t10) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10));
}
/**
* Converts an Array into an Observable that emits the items in the Array.
*
*
*
* - Scheduler:
* - {@code from} does not operate by default on a particular {@link Scheduler}.
*
*
* @param array
* the source Array
* @param
* the type of items in the Array and the type of items to be emitted by the resulting Observable
* @return an Observable that emits each item in the source Array
* @see RxJava wiki: from
* @see MSDN: Observable.ToObservable
*/
public final static Observable from(T[] array) {
return from(Arrays.asList(array));
}
/**
* Converts an Array into an Observable that emits the items in the Array on a specified Scheduler.
*
*
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param items
* the source Array
* @param scheduler
* the Scheduler on which the Observable emits the items of the Array
* @param
* the type of items in the Array and the type of items to be emitted by the resulting Observable
* @return an Observable that emits each item in the source Array
* @see RxJava wiki: from
* @see MSDN: Observable.ToObservable
* @deprecated use {@link #subscribeOn} to schedule the work
*/
@Deprecated
public final static Observable from(T[] items, Scheduler scheduler) {
return from(Arrays.asList(items), scheduler);
}
/**
* Returns an Observable that emits a sequential number every specified interval of time.
*
*
*
* - Scheduler:
* - {@code interval} operates by default on the {@code computation} {@link Scheduler}.
*
*
* @param interval
* interval size in time units (see below)
* @param unit
* time units to use for the interval size
* @return an Observable that emits a sequential number each time interval
* @see RxJava wiki: interval
* @see MSDN: Observable.Interval
*/
public final static Observable interval(long interval, TimeUnit unit) {
return interval(interval, unit, Schedulers.computation());
}
/**
* Returns an Observable that emits a sequential number every specified interval of time, on a
* specified Scheduler.
*
*
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param interval
* interval size in time units (see below)
* @param unit
* time units to use for the interval size
* @param scheduler
* the Scheduler to use for scheduling the items
* @return an Observable that emits a sequential number each time interval
* @see RxJava wiki: interval
* @see MSDN: Observable.Interval
*/
public final static Observable interval(long interval, TimeUnit unit, Scheduler scheduler) {
return create(new OnSubscribeTimerPeriodically(interval, interval, unit, scheduler));
}
/**
* Returns an Observable that emits a single item and then completes.
*
*
*
* To convert any object into an Observable that emits that object, pass that object into the {@code just}
* method.
*
* This is similar to the {@link #from(java.lang.Object[])} method, except that {@code from} will convert
* an {@link Iterable} object into an Observable that emits each of the items in the Iterable, one at a
* time, while the {@code just} method converts an Iterable into an Observable that emits the entire
* Iterable as a single item.
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param value
* the item to emit
* @param
* the type of that item
* @return an Observable that emits {@code value} as a single item and then completes
* @see RxJava wiki: just
*/
public final static Observable just(final T value) {
return ScalarSynchronousObservable.create(value);
}
/**
* Converts two items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2) {
return from(Arrays.asList(t1, t2));
}
/**
* Converts three items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3) {
return from(Arrays.asList(t1, t2, t3));
}
/**
* Converts four items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4) {
return from(Arrays.asList(t1, t2, t3, t4));
}
/**
* Converts five items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4, T t5) {
return from(Arrays.asList(t1, t2, t3, t4, t5));
}
/**
* Converts six items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4, T t5, T t6) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6));
}
/**
* Converts seven items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4, T t5, T t6, T t7) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7));
}
/**
* Converts eight items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param t8
* eighth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4, T t5, T t6, T t7, T t8) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8));
}
/**
* Converts nine items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param t8
* eighth item
* @param t9
* ninth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4, T t5, T t6, T t7, T t8, T t9) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8, t9));
}
/**
* Converts ten items into an Observable that emits those items.
*
*
*
* - Scheduler:
* - {@code just} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* first item
* @param t2
* second item
* @param t3
* third item
* @param t4
* fourth item
* @param t5
* fifth item
* @param t6
* sixth item
* @param t7
* seventh item
* @param t8
* eighth item
* @param t9
* ninth item
* @param t10
* tenth item
* @param
* the type of these items
* @return an Observable that emits each item
* @see RxJava wiki: just
*/
// suppress unchecked because we are using varargs inside the method
@SuppressWarnings("unchecked")
public final static Observable just(T t1, T t2, T t3, T t4, T t5, T t6, T t7, T t8, T t9, T t10) {
return from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10));
}
/**
* Flattens an Iterable of Observables into one Observable, without any transformation.
*
*
*
* You can combine the items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param sequences
* the Iterable of Observables
* @return an Observable that emits items that are the result of flattening the items emitted by the
* Observables in the Iterable
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
public final static Observable merge(Iterable extends Observable extends T>> sequences) {
return merge(from(sequences));
}
/**
* Flattens an Iterable of Observables into one Observable, without any transformation, while limiting the
* number of concurrent subscriptions to these Observables.
*
*
*
* You can combine the items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param sequences
* the Iterable of Observables
* @param maxConcurrent
* the maximum number of Observables that may be subscribed to concurrently
* @return an Observable that emits items that are the result of flattening the items emitted by the
* Observables in the Iterable
* @throws IllegalArgumentException
* if {@code maxConcurrent} is less than or equal to 0
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
public final static Observable merge(Iterable extends Observable extends T>> sequences, int maxConcurrent) {
return merge(from(sequences), maxConcurrent);
}
/**
* Flattens an Iterable of Observables into one Observable, without any transformation, while limiting the
* number of concurrent subscriptions to these Observables, and subscribing to these Observables on a
* specified Scheduler.
*
*
*
* You can combine the items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param sequences
* the Iterable of Observables
* @param maxConcurrent
* the maximum number of Observables that may be subscribed to concurrently
* @param scheduler
* the Scheduler on which to traverse the Iterable of Observables
* @return an Observable that emits items that are the result of flattening the items emitted by the
* Observables in the Iterable
* @throws IllegalArgumentException
* if {@code maxConcurrent} is less than or equal to 0
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
* @deprecated use {@link #subscribeOn} to schedule
*/
@Deprecated
public final static Observable merge(Iterable extends Observable extends T>> sequences, int maxConcurrent, Scheduler scheduler) {
return merge(from(sequences, scheduler), maxConcurrent);
}
/**
* Flattens an Iterable of Observables into one Observable, without any transformation, subscribing to these
* Observables on a specified Scheduler.
*
*
*
* You can combine the items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param sequences
* the Iterable of Observables
* @param scheduler
* the Scheduler on which to traverse the Iterable of Observables
* @return an Observable that emits items that are the result of flattening the items emitted by the
* Observables in the Iterable
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
* @deprecated use {@link #subscribeOn} to schedule
*/
@Deprecated
public final static Observable merge(Iterable extends Observable extends T>> sequences, Scheduler scheduler) {
return merge(from(sequences, scheduler));
}
/**
* Flattens an Observable that emits Observables into a single Observable that emits the items emitted by
* those Observables, without any transformation.
*
*
*
* You can combine the items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param source
* an Observable that emits Observables
* @return an Observable that emits items that are the result of flattening the Observables emitted by the
* {@code source} Observable
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
public final static Observable merge(Observable extends Observable extends T>> source) {
return source.lift(new OperatorMerge());
}
/**
* Flattens an Observable that emits Observables into a single Observable that emits the items emitted by
* those Observables, without any transformation, while limiting the maximum number of concurrent
* subscriptions to these Observables.
*
*
*
* You can combine the items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param source
* an Observable that emits Observables
* @param maxConcurrent
* the maximum number of Observables that may be subscribed to concurrently
* @return an Observable that emits items that are the result of flattening the Observables emitted by the
* {@code source} Observable
* @throws IllegalArgumentException
* if {@code maxConcurrent} is less than or equal to 0
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
public final static Observable merge(Observable extends Observable extends T>> source, int maxConcurrent) {
return source.lift(new OperatorMergeMaxConcurrent(maxConcurrent));
}
/**
* Flattens two Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2) {
return merge(from(Arrays.asList(t1, t2)));
}
/**
* Flattens three Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3) {
return merge(from(Arrays.asList(t1, t2, t3)));
}
/**
* Flattens four Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4) {
return merge(from(Arrays.asList(t1, t2, t3, t4)));
}
/**
* Flattens five Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5) {
return merge(from(Arrays.asList(t1, t2, t3, t4, t5)));
}
/**
* Flattens six Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6) {
return merge(from(Arrays.asList(t1, t2, t3, t4, t5, t6)));
}
/**
* Flattens seven Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @param t7
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7) {
return merge(from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7)));
}
/**
* Flattens eight Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @param t7
* an Observable to be merged
* @param t8
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7, Observable extends T> t8) {
return merge(from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8)));
}
/**
* Flattens nine Observables into a single Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @param t7
* an Observable to be merged
* @param t8
* an Observable to be merged
* @param t9
* an Observable to be merged
* @return an Observable that emits all of the items emitted by the source Observables
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
@SuppressWarnings("unchecked")
public final static Observable merge(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7, Observable extends T> t8, Observable extends T> t9) {
return merge(from(Arrays.asList(t1, t2, t3, t4, t5, t6, t7, t8, t9)));
}
/**
* Flattens an Array of Observables into one Observable, without any transformation.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - {@code merge} does not operate by default on a particular {@link Scheduler}.
*
*
* @param sequences
* the Array of Observables
* @return an Observable that emits all of the items emitted by the Observables in the Array
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
*/
public final static Observable merge(Observable extends T>[] sequences) {
return merge(from(sequences));
}
/**
* Flattens an Array of Observables into one Observable, without any transformation, traversing the array on
* a specified Scheduler.
*
*
*
* You can combine items emitted by multiple Observables so that they appear as a single Observable, by
* using the {@code merge} method.
*
* - Scheduler:
* - you specify which {@link Scheduler} this operator will use
*
*
* @param sequences
* the Array of Observables
* @param scheduler
* the Scheduler on which to traverse the Array
* @return an Observable that emits all of the items emitted by the Observables in the Array
* @see RxJava wiki: merge
* @see MSDN: Observable.Merge
* @deprecated use {@link #subscribeOn} to schedule
*/
@Deprecated
public final static Observable merge(Observable extends T>[] sequences, Scheduler scheduler) {
return merge(from(sequences, scheduler));
}
/**
* Flattens an Observable that emits Observables into one Observable, in a way that allows an Observer to
* receive all successfully emitted items from all of the source Observables without being interrupted by
* an error notification from one of them.
*
* This behaves like {@link #merge(Observable)} except that if any of the merged Observables notify of an
* error via {@link Observer#onError onError}, {@code mergeDelayError} will refrain from propagating that
* error notification until all of the merged Observables have finished emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param source
* an Observable that emits Observables
* @return an Observable that emits all of the items emitted by the Observables emitted by the
* {@code source} Observable
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends Observable extends T>> source) {
return source.lift(new OperatorMergeDelayError());
}
/**
* Flattens two Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from each of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable)} except that if any of the merged Observables
* notify of an error via {@link Observer#onError onError}, {@code mergeDelayError} will refrain from
* propagating that error notification until all of the merged Observables have finished emitting items.
*
*
*
* Even if both merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the two source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2) {
return mergeDelayError(just(t1, t2));
}
/**
* Flattens three Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable, Observable)} except that if any of the merged
* Observables notify of an error via {@link Observer#onError onError}, {@code mergeDelayError} will refrain
* from propagating that error notification until all of the merged Observables have finished emitting
* items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3) {
return mergeDelayError(just(t1, t2, t3));
}
/**
* Flattens four Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable, Observable, Observable)} except that if any of
* the merged Observables notify of an error via {@link Observer#onError onError}, {@code mergeDelayError}
* will refrain from propagating that error notification until all of the merged Observables have finished
* emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4) {
return mergeDelayError(just(t1, t2, t3, t4));
}
/**
* Flattens five Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable, Observable, Observable, Observable)} except that
* if any of the merged Observables notify of an error via {@link Observer#onError onError},
* {@code mergeDelayError} will refrain from propagating that error notification until all of the merged
* Observables have finished emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5) {
return mergeDelayError(just(t1, t2, t3, t4, t5));
}
/**
* Flattens six Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable, Observable, Observable, Observable, Observable)}
* except that if any of the merged Observables notify of an error via {@link Observer#onError onError},
* {@code mergeDelayError} will refrain from propagating that error notification until all of the merged
* Observables have finished emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6) {
return mergeDelayError(just(t1, t2, t3, t4, t5, t6));
}
/**
* Flattens seven Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like
* {@link #merge(Observable, Observable, Observable, Observable, Observable, Observable, Observable)}
* except that if any of the merged Observables notify of an error via {@link Observer#onError onError},
* {@code mergeDelayError} will refrain from propagating that error notification until all of the merged
* Observables have finished emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @param t7
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7) {
return mergeDelayError(just(t1, t2, t3, t4, t5, t6, t7));
}
/**
* Flattens eight Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable, Observable, Observable, Observable, Observable, Observable, Observable)}
* except that if any of the merged Observables notify of an error via {@link Observer#onError onError},
* {@code mergeDelayError} will refrain from propagating that error notification until all of the merged
* Observables have finished emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @param t7
* an Observable to be merged
* @param t8
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
// suppress because the types are checked by the method signature before using a vararg
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7, Observable extends T> t8) {
return mergeDelayError(just(t1, t2, t3, t4, t5, t6, t7, t8));
}
/**
* Flattens nine Observables into one Observable, in a way that allows an Observer to receive all
* successfully emitted items from all of the source Observables without being interrupted by an error
* notification from one of them.
*
* This behaves like {@link #merge(Observable, Observable, Observable, Observable, Observable, Observable, Observable, Observable, Observable)}
* except that if any of the merged Observables notify of an error via {@link Observer#onError onError},
* {@code mergeDelayError} will refrain from propagating that error notification until all of the merged
* Observables have finished emitting items.
*
*
*
* Even if multiple merged Observables send {@code onError} notifications, {@code mergeDelayError} will only
* invoke the {@code onError} method of its Observers once.
*
* - Scheduler:
* - {@code mergeDelayError} does not operate by default on a particular {@link Scheduler}.
*
*
* @param t1
* an Observable to be merged
* @param t2
* an Observable to be merged
* @param t3
* an Observable to be merged
* @param t4
* an Observable to be merged
* @param t5
* an Observable to be merged
* @param t6
* an Observable to be merged
* @param t7
* an Observable to be merged
* @param t8
* an Observable to be merged
* @param t9
* an Observable to be merged
* @return an Observable that emits all of the items that are emitted by the source Observables
* @see RxJava wiki: mergeDelayError
* @see MSDN: Observable.Merge
*/
public final static Observable mergeDelayError(Observable extends T> t1, Observable extends T> t2, Observable extends T> t3, Observable extends T> t4, Observable extends T> t5, Observable extends T> t6, Observable extends T> t7, Observable extends T> t8, Observable extends T> t9) {
return mergeDelayError(just(t1, t2, t3, t4, t5, t6, t7, t8, t9));
}
/**
* Converts the source {@code Observable} into an {@code Observable>} that emits the
* source Observable as its single emission.
*