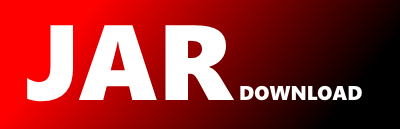
rx.internal.operators.BufferUntilSubscriber Maven / Gradle / Ivy
Show all versions of rxjava-core Show documentation
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.internal.operators;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.atomic.AtomicReferenceFieldUpdater;
import rx.Observer;
import rx.Subscriber;
import rx.functions.Action0;
import rx.observers.EmptyObserver;
import rx.observers.Subscribers;
import rx.subjects.Subject;
import rx.subscriptions.Subscriptions;
/**
* A solution to the "time gap" problem that occurs with {@code groupBy} and {@code pivot}.
*
* This currently has temporary unbounded buffers. It needs to become bounded and then do one of two things:
*
* - blow up and make the user do something about it
* - work with the backpressure solution ... still to be implemented (such as co-routines)
*
* Generally the buffer should be very short lived (milliseconds) and then stops being involved. It can become a
* memory leak though if a {@code GroupedObservable} backed by this class is emitted but never subscribed to
* (such as filtered out). In that case, either a time-bomb to throw away the buffer, or just blowing up and
* making the user do something about it is needed.
*
* For example, to filter out {@code GroupedObservable}s, perhaps they need a silent {@code subscribe()} on them
* to just blackhole the data.
*
* This is an initial start at solving this problem and solves the immediate problem of {@code groupBy} and
* {@code pivot} and trades off the possibility of memory leak for deterministic functionality.
*
* @see the Github issue describing the time gap problem
* @param
* the type of the items to be buffered
*/
public class BufferUntilSubscriber extends Subject {
@SuppressWarnings("rawtypes")
private final static Observer EMPTY_OBSERVER = new EmptyObserver();
/**
* @warn create() undescribed
* @return
*/
public static BufferUntilSubscriber create() {
State state = new State();
return new BufferUntilSubscriber(state);
}
/** The common state. */
static final class State {
volatile Observer super T> observerRef = null;
/** Field updater for observerRef. */
@SuppressWarnings("rawtypes")
static final AtomicReferenceFieldUpdater OBSERVER_UPDATER
= AtomicReferenceFieldUpdater.newUpdater(State.class, Observer.class, "observerRef");
boolean casObserverRef(Observer super T> expected, Observer super T> next) {
return OBSERVER_UPDATER.compareAndSet(this, expected, next);
}
Object guard = new Object();
/* protected by guard */
boolean emitting = false;
final ConcurrentLinkedQueue