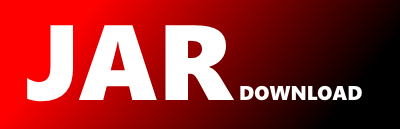
rx.internal.operators.OperatorOnExceptionResumeNextViaObservable Maven / Gradle / Ivy
Show all versions of rxjava-core Show documentation
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package rx.internal.operators;
import rx.Observable;
import rx.Observable.Operator;
import rx.Subscriber;
import rx.exceptions.Exceptions;
import rx.plugins.RxJavaPlugins;
/**
* Instruct an Observable to pass control to another Observable rather than invoking
* onError
if it encounters an error of type {@link java.lang.Exception}.
*
* This differs from {@link Observable#onErrorResumeNext} in that this one does not handle
* {@link java.lang.Throwable} or {@link java.lang.Error} but lets those continue through.
*
*
*
* By default, when an Observable encounters an error that prevents it from emitting the expected
* item to its Observer, the Observable invokes its Observer's onError
method, and
* then quits without invoking any more of its Observer's methods. The onErrorResumeNext operation
* changes this behavior. If you pass an Observable (resumeSequence) to onErrorResumeNext, if the
* source Observable encounters an error, instead of invoking its Observer's onError
* method, it will instead relinquish control to this new Observable, which will invoke the
* Observer's onNext
method if it is able to do so. In such a case, because no
* Observable necessarily invokes onError
, the Observer may never know that an error
* happened.
*
* You can use this to prevent errors from propagating or to supply fallback data should errors be
* encountered.
*
* @param the value type
*/
public final class OperatorOnExceptionResumeNextViaObservable implements Operator {
final Observable extends T> resumeSequence;
public OperatorOnExceptionResumeNextViaObservable(Observable extends T> resumeSequence) {
this.resumeSequence = resumeSequence;
}
@Override
public Subscriber super T> call(final Subscriber super T> child) {
// needs to independently unsubscribe so child can continue with the resume
Subscriber s = new Subscriber() {
private boolean done = false;
@Override
public void onNext(T t) {
if (done) {
return;
}
child.onNext(t);
}
@Override
public void onError(Throwable e) {
if (done) {
Exceptions.throwIfFatal(e);
return;
}
done = true;
if (e instanceof Exception) {
RxJavaPlugins.getInstance().getErrorHandler().handleError(e);
unsubscribe();
resumeSequence.unsafeSubscribe(child);
} else {
child.onError(e);
}
}
@Override
public void onCompleted() {
if (done) {
return;
}
done = true;
child.onCompleted();
}
};
child.add(s);
return s;
}
}