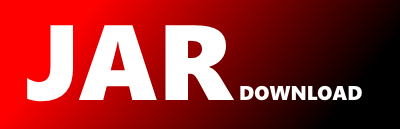
rx.observables.MathObservable Maven / Gradle / Ivy
/**
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package rx.observables;
import java.util.Comparator;
import rx.Observable;
import rx.functions.Func1;
import rx.functions.Functions;
import rx.math.operators.OperatorMinMax;
import rx.math.operators.OperatorSum;
import rx.math.operators.OperatorAverageDouble;
import rx.math.operators.OperatorAverageFloat;
import rx.math.operators.OperatorAverageInteger;
import rx.math.operators.OperatorAverageLong;
public class MathObservable {
private final Observable o;
private MathObservable(Observable o) {
this.o = o;
}
public static MathObservable from(Observable o) {
return new MathObservable(o);
}
/**
* Returns an Observable that emits the average of the Doubles emitted by the source Observable.
*
*
*
* @param source
* source Observable to compute the average of
* @return an Observable that emits a single item: the average of all the Doubles emitted by the source
* Observable
* @see RxJava Wiki: averageDouble()
* @see MSDN: Observable.Average
*/
public final static Observable averageDouble(Observable source) {
return source.lift(new OperatorAverageDouble(Functions.identity()));
}
/**
* Returns an Observable that emits the average of the Floats emitted by the source Observable.
*
*
*
* @param source
* source Observable to compute the average of
* @return an Observable that emits a single item: the average of all the Floats emitted by the source
* Observable
* @see RxJava Wiki: averageFloat()
* @see MSDN: Observable.Average
*/
public final static Observable averageFloat(Observable source) {
return source.lift(new OperatorAverageFloat(Functions.identity()));
}
/**
* Returns an Observable that emits the average of the Integers emitted by the source Observable.
*
*
*
* @param source
* source Observable to compute the average of
* @return an Observable that emits a single item: the average of all the Integers emitted by the source
* Observable
* @throws IllegalArgumentException
* if the source Observable emits no items
* @see RxJava Wiki: averageInteger()
* @see MSDN: Observable.Average
*/
public final static Observable averageInteger(Observable source) {
return source.lift(new OperatorAverageInteger(Functions.identity()));
}
/**
* Returns an Observable that emits the average of the Longs emitted by the source Observable.
*
*
*
* @param source
* source Observable to compute the average of
* @return an Observable that emits a single item: the average of all the Longs emitted by the source
* Observable
* @see RxJava Wiki: averageLong()
* @see MSDN: Observable.Average
*/
public final static Observable averageLong(Observable source) {
return source.lift(new OperatorAverageLong(Functions.identity()));
}
/**
* Returns an Observable that emits the single item emitted by the source Observable with the maximum
* numeric value. If there is more than one item with the same maximum value, it emits the last-emitted of
* these.
*
*
*
* @param source
* an Observable to scan for the maximum emitted item
* @return an Observable that emits this maximum item
* @throws IllegalArgumentException
* if the source is empty
* @see RxJava Wiki: max()
* @see MSDN: Observable.Max
*/
public final static > Observable max(Observable source) {
return OperatorMinMax.max(source);
}
/**
* Returns an Observable that emits the single numerically minimum item emitted by the source Observable.
* If there is more than one such item, it returns the last-emitted one.
*
*
*
* @param source
* an Observable to determine the minimum item of
* @return an Observable that emits the minimum item emitted by the source Observable
* @throws IllegalArgumentException
* if the source is empty
* @see MSDN: Observable.Min
*/
public final static > Observable min(Observable source) {
return OperatorMinMax.min(source);
}
/**
* Returns an Observable that emits the sum of all the Doubles emitted by the source Observable.
*
*
*
* @param source
* the source Observable to compute the sum of
* @return an Observable that emits a single item: the sum of all the Doubles emitted by the source
* Observable
* @see RxJava Wiki: sumDouble()
* @see MSDN: Observable.Sum
*/
public final static Observable sumDouble(Observable source) {
return OperatorSum.sumDoubles(source);
}
/**
* Returns an Observable that emits the sum of all the Floats emitted by the source Observable.
*
*
*
* @param source
* the source Observable to compute the sum of
* @return an Observable that emits a single item: the sum of all the Floats emitted by the source
* Observable
* @see RxJava Wiki: sumFloat()
* @see MSDN: Observable.Sum
*/
public final static Observable sumFloat(Observable source) {
return OperatorSum.sumFloats(source);
}
/**
* Returns an Observable that emits the sum of all the Integers emitted by the source Observable.
*
*
*
* @param source
* source Observable to compute the sum of
* @return an Observable that emits a single item: the sum of all the Integers emitted by the source
* Observable
* @see RxJava Wiki: sumInteger()
* @see MSDN: Observable.Sum
*/
public final static Observable sumInteger(Observable source) {
return OperatorSum.sumIntegers(source);
}
/**
* Returns an Observable that emits the sum of all the Longs emitted by the source Observable.
*
*
*
* @param source
* source Observable to compute the sum of
* @return an Observable that emits a single item: the sum of all the Longs emitted by the
* source Observable
* @see RxJava Wiki: sumLong()
* @see MSDN: Observable.Sum
*/
public final static Observable sumLong(Observable source) {
return OperatorSum.sumLongs(source);
}
/**
* Returns an Observable that transforms items emitted by the source Observable into Doubles by using a
* function you provide and then emits the Double average of the complete sequence of transformed values.
*
*
*
* @param valueExtractor
* the function to transform an item emitted by the source Observable into a Double
* @return an Observable that emits a single item: the Double average of the complete sequence of items
* emitted by the source Observable when transformed into Doubles by the specified function
* @see RxJava Wiki: averageDouble()
* @see MSDN: Observable.Average
*/
public final Observable averageDouble(Func1 super T, Double> valueExtractor) {
return o.lift(new OperatorAverageDouble(valueExtractor));
}
/**
* Returns an Observable that transforms items emitted by the source Observable into Floats by using a
* function you provide and then emits the Float average of the complete sequence of transformed values.
*
*
*
* @param valueExtractor
* the function to transform an item emitted by the source Observable into a Float
* @return an Observable that emits a single item: the Float average of the complete sequence of items
* emitted by the source Observable when transformed into Floats by the specified function
* @see RxJava Wiki: averageFloat()
* @see MSDN: Observable.Average
*/
public final Observable averageFloat(Func1 super T, Float> valueExtractor) {
return o.lift(new OperatorAverageFloat(valueExtractor));
}
/**
* Returns an Observable that transforms items emitted by the source Observable into Integers by using a
* function you provide and then emits the Integer average of the complete sequence of transformed values.
*
*
*
* @param valueExtractor
* the function to transform an item emitted by the source Observable into an Integer
* @return an Observable that emits a single item: the Integer average of the complete sequence of items
* emitted by the source Observable when transformed into Integers by the specified function
* @see RxJava Wiki: averageInteger()
* @see MSDN: Observable.Average
*/
public final Observable averageInteger(Func1 super T, Integer> valueExtractor) {
return o.lift(new OperatorAverageInteger(valueExtractor));
}
/**
* Returns an Observable that transforms items emitted by the source Observable into Longs by using a
* function you provide and then emits the Long average of the complete sequence of transformed values.
*
*
*
* @param valueExtractor
* the function to transform an item emitted by the source Observable into a Long
* @return an Observable that emits a single item: the Long average of the complete sequence of items
* emitted by the source Observable when transformed into Longs by the specified function
* @see RxJava Wiki: averageLong()
* @see MSDN: Observable.Average
*/
public final Observable averageLong(Func1 super T, Long> valueExtractor) {
return o.lift(new OperatorAverageLong(valueExtractor));
}
/**
* Returns an Observable that emits the maximum item emitted by the source Observable, according to the
* specified comparator. If there is more than one item with the same maximum value, it emits the
* last-emitted of these.
*
*
*
* @param comparator
* the comparer used to compare items
* @return an Observable that emits the maximum item emitted by the source Observable, according to the
* specified comparator
* @throws IllegalArgumentException
* if the source is empty
* @see RxJava Wiki: max()
* @see MSDN: Observable.Max
*/
public final Observable max(Comparator super T> comparator) {
return OperatorMinMax.max(o, comparator);
}
/**
* Returns an Observable that emits the minimum item emitted by the source Observable, according to a
* specified comparator. If there is more than one such item, it returns the last-emitted one.
*
*
*
* @param comparator
* the comparer used to compare elements
* @return an Observable that emits the minimum item emitted by the source Observable according to the
* specified comparator
* @throws IllegalArgumentException
* if the source is empty
* @see RxJava Wiki: min()
* @see MSDN: Observable.Min
*/
public final Observable min(Comparator super T> comparator) {
return OperatorMinMax.min(o, comparator);
}
/**
* Returns an Observable that extracts a Double from each of the items emitted by the source Observable via
* a function you specify, and then emits the sum of these Doubles.
*
*
*
* @param valueExtractor
* the function to extract a Double from each item emitted by the source Observable
* @return an Observable that emits the Double sum of the Double values corresponding to the items emitted
* by the source Observable as transformed by the provided function
* @see RxJava Wiki: sumDouble()
* @see MSDN: Observable.Sum
*/
public final Observable sumDouble(Func1 super T, Double> valueExtractor) {
return OperatorSum.sumAtLeastOneDoubles(o.map(valueExtractor));
}
/**
* Returns an Observable that extracts a Float from each of the items emitted by the source Observable via
* a function you specify, and then emits the sum of these Floats.
*
*
*
* @param valueExtractor
* the function to extract a Float from each item emitted by the source Observable
* @return an Observable that emits the Float sum of the Float values corresponding to the items emitted by
* the source Observable as transformed by the provided function
* @see RxJava Wiki: sumFloat()
* @see MSDN: Observable.Sum
*/
public final Observable sumFloat(Func1 super T, Float> valueExtractor) {
return OperatorSum.sumAtLeastOneFloats(o.map(valueExtractor));
}
/**
* Returns an Observable that extracts an Integer from each of the items emitted by the source Observable
* via a function you specify, and then emits the sum of these Integers.
*
*
*
* @param valueExtractor
* the function to extract an Integer from each item emitted by the source Observable
* @return an Observable that emits the Integer sum of the Integer values corresponding to the items emitted
* by the source Observable as transformed by the provided function
* @see RxJava Wiki: sumInteger()
* @see MSDN: Observable.Sum
*/
public final Observable sumInteger(Func1 super T, Integer> valueExtractor) {
return OperatorSum.sumAtLeastOneIntegers(o.map(valueExtractor));
}
/**
* Returns an Observable that extracts a Long from each of the items emitted by the source Observable via a
* function you specify, and then emits the sum of these Longs.
*
*
*
* @param valueExtractor
* the function to extract a Long from each item emitted by the source Observable
* @return an Observable that emits the Long sum of the Long values corresponding to the items emitted by
* the source Observable as transformed by the provided function
* @see RxJava Wiki: sumLong()
* @see MSDN: Observable.Sum
*/
public final Observable sumLong(Func1 super T, Long> valueExtractor) {
return OperatorSum.sumAtLeastOneLongs(o.map(valueExtractor));
}
}