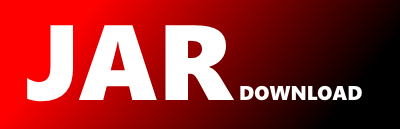
io.reactivex.netty.examples.http.chunk.HttpChunkServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rx-netty-examples Show documentation
Show all versions of rx-netty-examples Show documentation
rx-netty-examples developed by Netflix
The newest version!
/*
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.reactivex.netty.examples.http.chunk;
import io.netty.buffer.ByteBuf;
import io.reactivex.netty.RxNetty;
import io.reactivex.netty.protocol.http.server.HttpServer;
import io.reactivex.netty.protocol.http.server.HttpServerRequest;
import io.reactivex.netty.protocol.http.server.HttpServerResponse;
import io.reactivex.netty.protocol.http.server.RequestHandler;
import rx.Observable;
import rx.functions.Action0;
import rx.functions.Func1;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* @author Tomasz Bak
*/
public class HttpChunkServer {
static final int DEFAULT_PORT = 8103;
private final int port;
private final String textFile;
public HttpChunkServer(int port, String textFile) {
this.port = port;
this.textFile = textFile;
}
public HttpServer createServer() {
HttpServer server = RxNetty.createHttpServer(port, new RequestHandler() {
@Override
public Observable handle(HttpServerRequest request, final HttpServerResponse response) {
try {
final Reader fileReader = new BufferedReader(new FileReader(textFile));
return createFileObservable(fileReader)
.flatMap(new Func1>() {
@Override
public Observable call(String text) {
return response.writeStringAndFlush(text);
}
}).finallyDo(new ReaderCloseAction(fileReader));
} catch (IOException e) {
return Observable.error(e);
}
}
});
System.out.println("HTTP chunk server started...");
return server;
}
private static Observable createFileObservable(final Reader reader) {
Iterable iterable = new Iterable() {
private final char[] charBuf = new char[16];
private int lastCount;
@Override
public Iterator iterator() {
return new Iterator() {
@Override
public boolean hasNext() {
try {
return lastCount > 0 || (lastCount = reader.read(charBuf)) > 0;
} catch (IOException e) {
lastCount = 0;
return false;
}
}
@Override
public String next() {
if (hasNext()) {
String next = new String(charBuf, 0, lastCount);
lastCount = 0;
return next;
}
throw new NoSuchElementException("no more data to return");
}
@Override
public void remove() {
// IGNORE
}
};
}
};
return Observable.from(iterable);
}
static class ReaderCloseAction implements Action0 {
private final Reader fileReader;
ReaderCloseAction(Reader fileReader) {
this.fileReader = fileReader;
}
@Override
public void call() {
try {
fileReader.close();
} catch (IOException e) {
// IGNORE
}
}
}
public static void main(String[] args) {
if (args.length < 1) {
System.err.println("ERROR: give text file name");
return;
}
String textFile = args[0];
new HttpChunkServer(DEFAULT_PORT, textFile).createServer().startAndWait();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy