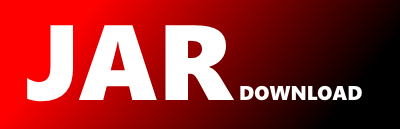
io.reactivex.netty.protocol.text.sse.ServerSentEventDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rx-netty Show documentation
Show all versions of rx-netty Show documentation
rx-netty developed by Netflix
/*
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.reactivex.netty.protocol.text.sse;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.ReplayingDecoder;
import java.util.List;
/**
* An decoder for server-sent event. It does not record retry or last event ID. Otherwise, it
* follows the same interpretation logic as documented here: Event Stream
* Interpretation
*/
public class ServerSentEventDecoder extends ReplayingDecoder {
private final MessageBuffer eventBuffer;
public ServerSentEventDecoder() {
super(State.NEW_LINE);
eventBuffer = new MessageBuffer();
}
private static boolean isLineDelimiter(char c) {
return c == '\r' || c == '\n';
}
private static class MessageBuffer {
private boolean eventTypePresent;
private final StringBuilder eventType = new StringBuilder();
private final StringBuilder eventData = new StringBuilder();
private boolean eventIdPresent;
private final StringBuilder eventId = new StringBuilder();
private MessageBuffer() {
reset();
}
public MessageBuffer setEventType(String eventType) {
this.eventType.setLength(0);
this.eventType.append(eventType);
eventTypePresent = true;
return this;
}
public MessageBuffer appendEventData(String eventData) {
if(this.eventData.length() > 0) {
this.eventData.append('\n');
}
this.eventData.append(eventData);
return this;
}
public MessageBuffer setEventId(String id) {
eventId.setLength(0);
eventId.append(id);
eventIdPresent = true;
return this;
}
public ServerSentEvent toMessage() {
ServerSentEvent message = new ServerSentEvent(
eventIdPresent ? eventId.toString() : null,
eventTypePresent ? eventType.toString() : null,
eventData.toString()
);
reset();
return message;
}
public void reset() {
eventIdPresent = false;
eventId.setLength(0);
eventTypePresent = false;
eventType.setLength(0);
eventData.setLength(0);
}
}
private boolean eventStarted;
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy