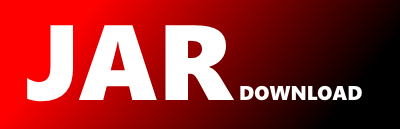
com.netflix.zeno.diff.history.DiffHistoricalState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netflix-zeno Show documentation
Show all versions of netflix-zeno Show documentation
netflix-zeno developed by Netflix
/*
*
* Copyright 2013 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.netflix.zeno.diff.history;
import com.netflix.zeno.util.collections.impl.OpenAddressingArraySet;
import com.netflix.zeno.util.collections.impl.OpenAddressingHashMap;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Represents a historical set of changes in a version of a FastBlobStateEngine
*
* @author dkoszewnik
*
*/
public class DiffHistoricalState {
private final String version;
private final Map> typeStates;
public DiffHistoricalState(String version) {
this.version = version;
this.typeStates = new ConcurrentHashMap>();
}
public String getVersion() {
return version;
}
@SuppressWarnings("unchecked")
public DiffHistoricalTypeStategetMap(String objectType) {
return (DiffHistoricalTypeState)typeStates.get(objectType);
}
public void addTypeState(String typeName, Map from, Map to) {
typeStates.put(typeName, createTypeState(from, to));
}
private DiffHistoricalTypeState createTypeState(Map from, Map to) {
int newCounter = 0;
int diffCounter = 0;
int deleteCounter = 0;
for(K key : from.keySet()) {
V toValue = to.get(key);
if(toValue == null) {
deleteCounter++;
} else {
V fromValue = from.get(key);
if(fromValue != toValue) {
diffCounter++;
}
}
}
for(K key : to.keySet()) {
if(!from.containsKey(key)) {
newCounter++;
}
}
OpenAddressingArraySet newSet = new OpenAddressingArraySet();
OpenAddressingHashMap diffMap = new OpenAddressingHashMap();
OpenAddressingHashMap deleteMap = new OpenAddressingHashMap();
newSet.builderInit(newCounter);
diffMap.builderInit(diffCounter);
deleteMap.builderInit(deleteCounter);
newCounter = diffCounter = deleteCounter = 0;
for(K key : from.keySet()) {
V fromValue = from.get(key);
V toValue = to.get(key);
if(toValue == null) {
deleteMap.builderPut(deleteCounter++, key, fromValue);
} else {
if(fromValue != toValue) {
diffMap.builderPut(diffCounter++, key, fromValue);
}
}
}
for(K key : to.keySet()) {
if(!from.containsKey(key)) {
newSet.builderSet(newCounter++, key);
}
}
newSet.builderFinish();
diffMap.builderFinish();
deleteMap.builderFinish();
return new DiffHistoricalTypeState(newSet, diffMap, deleteMap);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy