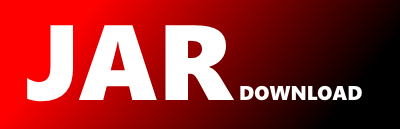
com.netgrif.application.engine.business.PostalCodeService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-engine Show documentation
Show all versions of application-engine Show documentation
System provides workflow management functions including user, role and data management.
package com.netgrif.application.engine.business;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
@Service
public class PostalCodeService implements IPostalCodeService {
@Autowired
private PostalCodeRepository repository;
@Override
public void savePostalCodes(Collection codes) {
List savedCodes = repository.saveAll(codes);
if (savedCodes.isEmpty()) {
throw new IllegalArgumentException("Could not save given postal codes");
}
}
@Override
public void createPostalCode(String code, String city) {
repository.save(new PostalCode(code.replaceAll("\\s", "").trim(), city.trim()));
}
@Override
public void savePostalCode(PostalCode postalCode) {
repository.save(postalCode);
}
@Override
public List findAllByCode(String code) {
if (code == null)
return new LinkedList<>();
return repository.findAllByCode(code.replaceAll("\\s", "").trim());
}
@Override
public List findAllByCity(String city) {
if (city == null)
return new LinkedList<>();
return repository.findAllByCity(city.trim());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy