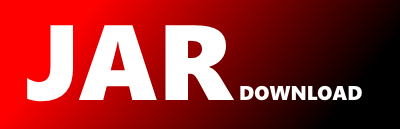
com.netgrif.application.engine.workflow.service.MongoSearchService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-engine Show documentation
Show all versions of application-engine Show documentation
System provides workflow management functions including user, role and data management.
package com.netgrif.application.engine.workflow.service;
import com.netgrif.application.engine.auth.domain.IUser;
import com.netgrif.application.engine.auth.service.interfaces.IUserService;
import com.querydsl.core.BooleanBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageImpl;
import org.springframework.data.domain.Pageable;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.query.BasicQuery;
import org.springframework.data.mongodb.core.query.Query;
import org.springframework.stereotype.Service;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.*;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Predicate;
@Service
public class MongoSearchService {
private static final Logger log = LoggerFactory.getLogger(MongoSearchService.class.getName());
private static final String ERROR_KEY = "ERROR";
@Autowired
private IUserService userService;
@Autowired
private MongoTemplate mongoTemplate;
private Class tClass;
public Page search(Map searchRequest, Pageable pageable, Class clazz) {
try {
this.tClass = clazz;
return executeQuery(buildQuery(resolveRequest(searchRequest)), pageable);
} catch (IllegalQueryException e) {
log.error("Searching failed: ", e);
return new PageImpl<>(new ArrayList<>(), pageable, 0);
}
}
protected Map resolveRequest(Map request) {
Map queryParts = new LinkedHashMap<>();
boolean match = request.entrySet().stream().allMatch((Map.Entry entry) -> {
try {
Method method = this.getClass().getMethod(entry.getKey() + "Query", Object.class);
// log.info("Resolved attribute of " + tClass.getSimpleName() + ": " + entry.getKey());
Object part = method.invoke(this, entry.getValue());
if (part != null) //TODO 23.7.2017 throw exception when cannot build query
queryParts.put(entry.getKey(), part);
return true;
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
log.error("Resolving request failed: ", e);
queryParts.put(ERROR_KEY, "Parameter " + entry.getKey() + " is not supported in " + tClass.getSimpleName() + " search!");
return false;
}
});
return queryParts;
}
public String buildQuery(Map queryParts) throws IllegalQueryException {
StringBuilder builder = new StringBuilder();
builder.append("{");
boolean result = queryParts.entrySet().stream().allMatch(entry -> {
if (entry.getKey().equals(ERROR_KEY)) return false;
if (((String) entry.getValue()).endsWith(":")) {
queryParts.put(ERROR_KEY, "Query attribute " + entry.getKey() + " has wrong value " + entry.getValue());
return false;
}
// log.info("Query: " + entry.getValue());
builder.append(entry.getValue());
builder.append(",");
return true;
});
if (!result)
throw new IllegalQueryException((String) (queryParts.get(ERROR_KEY)));
if (builder.length() > 1)
builder.deleteCharAt(builder.length() - 1);
builder.append("}");
return builder.toString();
}
protected Page executeQuery(String queryString, Pageable pageable) {
Query query = new BasicQuery(queryString).with(pageable);
log.info("Executing search query: " + queryString);
return new PageImpl<>(mongoTemplate.find(query, tClass),
pageable,
mongoTemplate.count(new BasicQuery(queryString, "{_id:1}"), tClass));
}
// **************************
// * Query building methods *
// **************************
public String idQuery(Object obj) {
Map> builder = new HashMap<>();
builder.put(ArrayList.class, o -> in((List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy