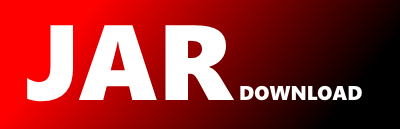
com.netgrif.application.engine.importer.model.Data Maven / Gradle / Ivy
Show all versions of application-engine Show documentation
package com.netgrif.application.engine.importer.model;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for data complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="data">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="title" type="{}i18nStringType"/>
* <element name="placeholder" type="{}i18nStringType" minOccurs="0"/>
* <element name="desc" type="{}i18nStringType" minOccurs="0"/>
* <choice>
* <element name="values" type="{}i18nStringTypeWithExpression" maxOccurs="unbounded" minOccurs="0"/>
* <element name="options" type="{}options" minOccurs="0"/>
* </choice>
* <choice>
* <element name="valid" type="{}valid" maxOccurs="unbounded" minOccurs="0"/>
* <element name="validations" type="{}validations" minOccurs="0"/>
* </choice>
* <choice>
* <element name="init" type="{}init" minOccurs="0"/>
* <element name="inits" type="{}inits" minOccurs="0"/>
* </choice>
* <element name="format" type="{}format" minOccurs="0"/>
* <choice>
* <element name="view" type="{}fieldView" minOccurs="0"/>
* </choice>
* <element name="component" type="{}component" minOccurs="0"/>
* <element name="encryption" type="{}encryption" minOccurs="0"/>
* <choice>
* <element name="action" type="{}action" maxOccurs="unbounded" minOccurs="0"/>
* <element name="event" type="{}dataEvent" maxOccurs="unbounded" minOccurs="0"/>
* </choice>
* <element name="actionRef" type="{}actionRef" maxOccurs="unbounded" minOccurs="0"/>
* <element name="documentRef" type="{}documentRef" minOccurs="0"/>
* <element name="storage" type="{}storage" minOccurs="0"/>
* <element name="remote" type="{}remote" minOccurs="0"/>
* <element name="length" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="allowedNets" type="{}allowedNets" minOccurs="0"/>
* </sequence>
* <attribute name="type" use="required" type="{}data_type" />
* <attribute name="immediate" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "data", propOrder = {
"id",
"title",
"placeholder",
"desc",
"values",
"options",
"valid",
"validations",
"init",
"inits",
"format",
"view",
"component",
"encryption",
"action",
"event",
"actionRef",
"documentRef",
"storage",
"remote",
"length",
"allowedNets"
})
public class Data {
@XmlElement(required = true)
protected String id;
@XmlElement(required = true)
protected I18NStringType title;
protected I18NStringType placeholder;
protected I18NStringType desc;
protected List values;
protected Options options;
protected List valid;
protected Validations validations;
protected Init init;
protected Inits inits;
protected Format format;
protected FieldView view;
protected Component component;
protected Encryption encryption;
protected List action;
protected List event;
protected List actionRef;
protected DocumentRef documentRef;
protected Storage storage;
protected String remote;
protected Integer length;
protected AllowedNets allowedNets;
@XmlAttribute(name = "type", required = true)
protected DataType type;
@XmlAttribute(name = "immediate")
protected Boolean immediate;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link I18NStringType }
*
*/
public I18NStringType getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link I18NStringType }
*
*/
public void setTitle(I18NStringType value) {
this.title = value;
}
/**
* Gets the value of the placeholder property.
*
* @return
* possible object is
* {@link I18NStringType }
*
*/
public I18NStringType getPlaceholder() {
return placeholder;
}
/**
* Sets the value of the placeholder property.
*
* @param value
* allowed object is
* {@link I18NStringType }
*
*/
public void setPlaceholder(I18NStringType value) {
this.placeholder = value;
}
/**
* Gets the value of the desc property.
*
* @return
* possible object is
* {@link I18NStringType }
*
*/
public I18NStringType getDesc() {
return desc;
}
/**
* Sets the value of the desc property.
*
* @param value
* allowed object is
* {@link I18NStringType }
*
*/
public void setDesc(I18NStringType value) {
this.desc = value;
}
/**
* Gets the value of the values property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the values property.
*
*
* For example, to add a new item, do as follows:
*
* getValues().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link I18NStringTypeWithExpression }
*
*
*/
public List getValues() {
if (values == null) {
values = new ArrayList();
}
return this.values;
}
/**
* Gets the value of the options property.
*
* @return
* possible object is
* {@link Options }
*
*/
public Options getOptions() {
return options;
}
/**
* Sets the value of the options property.
*
* @param value
* allowed object is
* {@link Options }
*
*/
public void setOptions(Options value) {
this.options = value;
}
/**
* Gets the value of the valid property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the valid property.
*
*
* For example, to add a new item, do as follows:
*
* getValid().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Valid }
*
*
*/
public List getValid() {
if (valid == null) {
valid = new ArrayList();
}
return this.valid;
}
/**
* Gets the value of the validations property.
*
* @return
* possible object is
* {@link Validations }
*
*/
public Validations getValidations() {
return validations;
}
/**
* Sets the value of the validations property.
*
* @param value
* allowed object is
* {@link Validations }
*
*/
public void setValidations(Validations value) {
this.validations = value;
}
/**
* Gets the value of the init property.
*
* @return
* possible object is
* {@link Init }
*
*/
public Init getInit() {
return init;
}
/**
* Sets the value of the init property.
*
* @param value
* allowed object is
* {@link Init }
*
*/
public void setInit(Init value) {
this.init = value;
}
/**
* Gets the value of the inits property.
*
* @return
* possible object is
* {@link Inits }
*
*/
public Inits getInits() {
return inits;
}
/**
* Sets the value of the inits property.
*
* @param value
* allowed object is
* {@link Inits }
*
*/
public void setInits(Inits value) {
this.inits = value;
}
/**
* Gets the value of the format property.
*
* @return
* possible object is
* {@link Format }
*
*/
public Format getFormat() {
return format;
}
/**
* Sets the value of the format property.
*
* @param value
* allowed object is
* {@link Format }
*
*/
public void setFormat(Format value) {
this.format = value;
}
/**
* Gets the value of the view property.
*
* @return
* possible object is
* {@link FieldView }
*
*/
public FieldView getView() {
return view;
}
/**
* Sets the value of the view property.
*
* @param value
* allowed object is
* {@link FieldView }
*
*/
public void setView(FieldView value) {
this.view = value;
}
/**
* Gets the value of the component property.
*
* @return
* possible object is
* {@link Component }
*
*/
public Component getComponent() {
return component;
}
/**
* Sets the value of the component property.
*
* @param value
* allowed object is
* {@link Component }
*
*/
public void setComponent(Component value) {
this.component = value;
}
/**
* Gets the value of the encryption property.
*
* @return
* possible object is
* {@link Encryption }
*
*/
public Encryption getEncryption() {
return encryption;
}
/**
* Sets the value of the encryption property.
*
* @param value
* allowed object is
* {@link Encryption }
*
*/
public void setEncryption(Encryption value) {
this.encryption = value;
}
/**
* Gets the value of the action property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the action property.
*
*
* For example, to add a new item, do as follows:
*
* getAction().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Action }
*
*
*/
public List getAction() {
if (action == null) {
action = new ArrayList();
}
return this.action;
}
/**
* Gets the value of the event property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the event property.
*
*
* For example, to add a new item, do as follows:
*
* getEvent().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DataEvent }
*
*
*/
public List getEvent() {
if (event == null) {
event = new ArrayList();
}
return this.event;
}
/**
* Gets the value of the actionRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the actionRef property.
*
*
* For example, to add a new item, do as follows:
*
* getActionRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ActionRef }
*
*
*/
public List getActionRef() {
if (actionRef == null) {
actionRef = new ArrayList();
}
return this.actionRef;
}
/**
* Gets the value of the documentRef property.
*
* @return
* possible object is
* {@link DocumentRef }
*
*/
public DocumentRef getDocumentRef() {
return documentRef;
}
/**
* Sets the value of the documentRef property.
*
* @param value
* allowed object is
* {@link DocumentRef }
*
*/
public void setDocumentRef(DocumentRef value) {
this.documentRef = value;
}
/**
* Gets the value of the storage property.
*
* @return
* possible object is
* {@link Storage }
*
*/
public Storage getStorage() {
return storage;
}
/**
* Sets the value of the storage property.
*
* @param value
* allowed object is
* {@link Storage }
*
*/
public void setStorage(Storage value) {
this.storage = value;
}
/**
* Gets the value of the remote property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRemote() {
return remote;
}
/**
* Sets the value of the remote property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRemote(String value) {
this.remote = value;
}
/**
* Gets the value of the length property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getLength() {
return length;
}
/**
* Sets the value of the length property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setLength(Integer value) {
this.length = value;
}
/**
* Gets the value of the allowedNets property.
*
* @return
* possible object is
* {@link AllowedNets }
*
*/
public AllowedNets getAllowedNets() {
return allowedNets;
}
/**
* Sets the value of the allowedNets property.
*
* @param value
* allowed object is
* {@link AllowedNets }
*
*/
public void setAllowedNets(AllowedNets value) {
this.allowedNets = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link DataType }
*
*/
public DataType getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link DataType }
*
*/
public void setType(DataType value) {
this.type = value;
}
/**
* Gets the value of the immediate property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isImmediate() {
return immediate;
}
/**
* Sets the value of the immediate property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setImmediate(Boolean value) {
this.immediate = value;
}
}