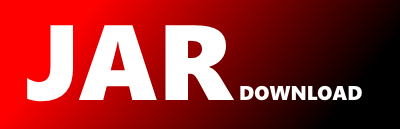
com.netgrif.application.engine.importer.model.ObjectFactory Maven / Gradle / Ivy
Show all versions of application-engine Show documentation
package com.netgrif.application.engine.importer.model;
import javax.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.netgrif.application.engine.importer.model package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.netgrif.application.engine.importer.model
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Document }
*
*/
public Document createDocument() {
return new Document();
}
/**
* Create an instance of {@link DocumentType }
*
*/
public DocumentType createDocumentType() {
return new DocumentType();
}
/**
* Create an instance of {@link I18NStringType }
*
*/
public I18NStringType createI18NStringType() {
return new I18NStringType();
}
/**
* Create an instance of {@link Tags }
*
*/
public Tags createTags() {
return new Tags();
}
/**
* Create an instance of {@link I18NStringTypeWithExpression }
*
*/
public I18NStringTypeWithExpression createI18NStringTypeWithExpression() {
return new I18NStringTypeWithExpression();
}
/**
* Create an instance of {@link CaseRoleRef }
*
*/
public CaseRoleRef createCaseRoleRef() {
return new CaseRoleRef();
}
/**
* Create an instance of {@link CaseUserRef }
*
*/
public CaseUserRef createCaseUserRef() {
return new CaseUserRef();
}
/**
* Create an instance of {@link ProcessEvents }
*
*/
public ProcessEvents createProcessEvents() {
return new ProcessEvents();
}
/**
* Create an instance of {@link CaseEvents }
*
*/
public CaseEvents createCaseEvents() {
return new CaseEvents();
}
/**
* Create an instance of {@link Transaction }
*
*/
public Transaction createTransaction() {
return new Transaction();
}
/**
* Create an instance of {@link Role }
*
*/
public Role createRole() {
return new Role();
}
/**
* Create an instance of {@link Function }
*
*/
public Function createFunction() {
return new Function();
}
/**
* Create an instance of {@link Data }
*
*/
public Data createData() {
return new Data();
}
/**
* Create an instance of {@link Mapping }
*
*/
public Mapping createMapping() {
return new Mapping();
}
/**
* Create an instance of {@link I18N }
*
*/
public I18N createI18N() {
return new I18N();
}
/**
* Create an instance of {@link Transition }
*
*/
public Transition createTransition() {
return new Transition();
}
/**
* Create an instance of {@link Place }
*
*/
public Place createPlace() {
return new Place();
}
/**
* Create an instance of {@link Arc }
*
*/
public Arc createArc() {
return new Arc();
}
/**
* Create an instance of {@link TransitionLayout }
*
*/
public TransitionLayout createTransitionLayout() {
return new TransitionLayout();
}
/**
* Create an instance of {@link Valid }
*
*/
public Valid createValid() {
return new Valid();
}
/**
* Create an instance of {@link Breakpoint }
*
*/
public Breakpoint createBreakpoint() {
return new Breakpoint();
}
/**
* Create an instance of {@link Options }
*
*/
public Options createOptions() {
return new Options();
}
/**
* Create an instance of {@link Inits }
*
*/
public Inits createInits() {
return new Inits();
}
/**
* Create an instance of {@link Expression }
*
*/
public Expression createExpression() {
return new Expression();
}
/**
* Create an instance of {@link Component }
*
*/
public Component createComponent() {
return new Component();
}
/**
* Create an instance of {@link Option }
*
*/
public Option createOption() {
return new Option();
}
/**
* Create an instance of {@link Property }
*
*/
public Property createProperty() {
return new Property();
}
/**
* Create an instance of {@link Properties }
*
*/
public Properties createProperties() {
return new Properties();
}
/**
* Create an instance of {@link Tag }
*
*/
public Tag createTag() {
return new Tag();
}
/**
* Create an instance of {@link Icons }
*
*/
public Icons createIcons() {
return new Icons();
}
/**
* Create an instance of {@link Icon }
*
*/
public Icon createIcon() {
return new Icon();
}
/**
* Create an instance of {@link AllowedNets }
*
*/
public AllowedNets createAllowedNets() {
return new AllowedNets();
}
/**
* Create an instance of {@link Logic }
*
*/
public Logic createLogic() {
return new Logic();
}
/**
* Create an instance of {@link CaseLogic }
*
*/
public CaseLogic createCaseLogic() {
return new CaseLogic();
}
/**
* Create an instance of {@link TransactionRef }
*
*/
public TransactionRef createTransactionRef() {
return new TransactionRef();
}
/**
* Create an instance of {@link RoleRef }
*
*/
public RoleRef createRoleRef() {
return new RoleRef();
}
/**
* Create an instance of {@link UserRef }
*
*/
public UserRef createUserRef() {
return new UserRef();
}
/**
* Create an instance of {@link PermissionRef }
*
*/
public PermissionRef createPermissionRef() {
return new PermissionRef();
}
/**
* Create an instance of {@link CasePermissionRef }
*
*/
public CasePermissionRef createCasePermissionRef() {
return new CasePermissionRef();
}
/**
* Create an instance of {@link DataRef }
*
*/
public DataRef createDataRef() {
return new DataRef();
}
/**
* Create an instance of {@link Layout }
*
*/
public Layout createLayout() {
return new Layout();
}
/**
* Create an instance of {@link AssignedUser }
*
*/
public AssignedUser createAssignedUser() {
return new AssignedUser();
}
/**
* Create an instance of {@link DataGroup }
*
*/
public DataGroup createDataGroup() {
return new DataGroup();
}
/**
* Create an instance of {@link Action }
*
*/
public Action createAction() {
return new Action();
}
/**
* Create an instance of {@link Validations }
*
*/
public Validations createValidations() {
return new Validations();
}
/**
* Create an instance of {@link Validation }
*
*/
public Validation createValidation() {
return new Validation();
}
/**
* Create an instance of {@link Arguments }
*
*/
public Arguments createArguments() {
return new Arguments();
}
/**
* Create an instance of {@link Argument }
*
*/
public Argument createArgument() {
return new Argument();
}
/**
* Create an instance of {@link Init }
*
*/
public Init createInit() {
return new Init();
}
/**
* Create an instance of {@link Storage }
*
*/
public Storage createStorage() {
return new Storage();
}
/**
* Create an instance of {@link Trigger }
*
*/
public Trigger createTrigger() {
return new Trigger();
}
/**
* Create an instance of {@link DocumentRef }
*
*/
public DocumentRef createDocumentRef() {
return new DocumentRef();
}
/**
* Create an instance of {@link Encryption }
*
*/
public Encryption createEncryption() {
return new Encryption();
}
/**
* Create an instance of {@link Event }
*
*/
public Event createEvent() {
return new Event();
}
/**
* Create an instance of {@link BaseEvent }
*
*/
public BaseEvent createBaseEvent() {
return new BaseEvent();
}
/**
* Create an instance of {@link DataEvent }
*
*/
public DataEvent createDataEvent() {
return new DataEvent();
}
/**
* Create an instance of {@link CaseEvent }
*
*/
public CaseEvent createCaseEvent() {
return new CaseEvent();
}
/**
* Create an instance of {@link ProcessEvent }
*
*/
public ProcessEvent createProcessEvent() {
return new ProcessEvent();
}
/**
* Create an instance of {@link Actions }
*
*/
public Actions createActions() {
return new Actions();
}
/**
* Create an instance of {@link ActionRef }
*
*/
public ActionRef createActionRef() {
return new ActionRef();
}
/**
* Create an instance of {@link FieldView }
*
*/
public FieldView createFieldView() {
return new FieldView();
}
/**
* Create an instance of {@link BooleanImageView }
*
*/
public BooleanImageView createBooleanImageView() {
return new BooleanImageView();
}
/**
* Create an instance of {@link Format }
*
*/
public Format createFormat() {
return new Format();
}
/**
* Create an instance of {@link Currency }
*
*/
public Currency createCurrency() {
return new Currency();
}
}