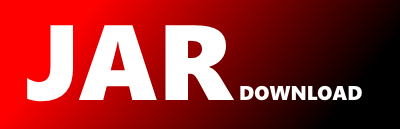
com.netgrif.application.engine.importer.model.Transition Maven / Gradle / Ivy
Show all versions of application-engine Show documentation
package com.netgrif.application.engine.importer.model;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for transition complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="transition">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="x" type="{}nonNegativeInteger"/>
* <element name="y" type="{}nonNegativeInteger"/>
* <element name="label" type="{}i18nStringType"/>
* <element name="tags" type="{}tags" minOccurs="0"/>
* <element name="layout" type="{}transitionLayout" minOccurs="0"/>
* <element name="icon" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="priority" type="{}nonNegativeInteger" minOccurs="0"/>
* <element name="assignPolicy" type="{}assignPolicy" minOccurs="0"/>
* <element name="dataFocusPolicy" type="{}dataFocusPolicy" minOccurs="0"/>
* <element name="finishPolicy" type="{}finishPolicy" minOccurs="0"/>
* <element name="trigger" type="{}trigger" maxOccurs="unbounded" minOccurs="0"/>
* <element name="transactionRef" type="{}transactionRef" minOccurs="0"/>
* <element name="roleRef" type="{}roleRef" maxOccurs="unbounded" minOccurs="0"/>
* <choice minOccurs="0">
* <element name="usersRef" type="{}userRef" maxOccurs="unbounded"/>
* <element name="userRef" type="{}userRef" maxOccurs="unbounded"/>
* </choice>
* <element name="assignedUser" type="{}assignedUser" minOccurs="0"/>
* <element name="dataRef" type="{}dataRef" maxOccurs="unbounded" minOccurs="0"/>
* <element name="dataGroup" type="{}dataGroup" maxOccurs="unbounded" minOccurs="0"/>
* <element name="event" type="{}event" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "transition", propOrder = {
"id",
"x",
"y",
"label",
"tags",
"layout",
"icon",
"priority",
"assignPolicy",
"dataFocusPolicy",
"finishPolicy",
"trigger",
"transactionRef",
"roleRef",
"usersRef",
"userRef",
"assignedUser",
"dataRef",
"dataGroup",
"event"
})
public class Transition {
@XmlElement(required = true)
protected String id;
protected int x;
protected int y;
@XmlElement(required = true)
protected I18NStringType label;
protected Tags tags;
protected TransitionLayout layout;
protected String icon;
protected Integer priority;
@XmlSchemaType(name = "string")
protected AssignPolicy assignPolicy;
@XmlSchemaType(name = "string")
protected DataFocusPolicy dataFocusPolicy;
@XmlSchemaType(name = "string")
protected FinishPolicy finishPolicy;
protected List trigger;
protected TransactionRef transactionRef;
protected List roleRef;
protected List usersRef;
protected List userRef;
protected AssignedUser assignedUser;
protected List dataRef;
protected List dataGroup;
protected List event;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the x property.
*
*/
public int getX() {
return x;
}
/**
* Sets the value of the x property.
*
*/
public void setX(int value) {
this.x = value;
}
/**
* Gets the value of the y property.
*
*/
public int getY() {
return y;
}
/**
* Sets the value of the y property.
*
*/
public void setY(int value) {
this.y = value;
}
/**
* Gets the value of the label property.
*
* @return
* possible object is
* {@link I18NStringType }
*
*/
public I18NStringType getLabel() {
return label;
}
/**
* Sets the value of the label property.
*
* @param value
* allowed object is
* {@link I18NStringType }
*
*/
public void setLabel(I18NStringType value) {
this.label = value;
}
/**
* Gets the value of the tags property.
*
* @return
* possible object is
* {@link Tags }
*
*/
public Tags getTags() {
return tags;
}
/**
* Sets the value of the tags property.
*
* @param value
* allowed object is
* {@link Tags }
*
*/
public void setTags(Tags value) {
this.tags = value;
}
/**
* Gets the value of the layout property.
*
* @return
* possible object is
* {@link TransitionLayout }
*
*/
public TransitionLayout getLayout() {
return layout;
}
/**
* Sets the value of the layout property.
*
* @param value
* allowed object is
* {@link TransitionLayout }
*
*/
public void setLayout(TransitionLayout value) {
this.layout = value;
}
/**
* Gets the value of the icon property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIcon() {
return icon;
}
/**
* Sets the value of the icon property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIcon(String value) {
this.icon = value;
}
/**
* Gets the value of the priority property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getPriority() {
return priority;
}
/**
* Sets the value of the priority property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setPriority(Integer value) {
this.priority = value;
}
/**
* Gets the value of the assignPolicy property.
*
* @return
* possible object is
* {@link AssignPolicy }
*
*/
public AssignPolicy getAssignPolicy() {
return assignPolicy;
}
/**
* Sets the value of the assignPolicy property.
*
* @param value
* allowed object is
* {@link AssignPolicy }
*
*/
public void setAssignPolicy(AssignPolicy value) {
this.assignPolicy = value;
}
/**
* Gets the value of the dataFocusPolicy property.
*
* @return
* possible object is
* {@link DataFocusPolicy }
*
*/
public DataFocusPolicy getDataFocusPolicy() {
return dataFocusPolicy;
}
/**
* Sets the value of the dataFocusPolicy property.
*
* @param value
* allowed object is
* {@link DataFocusPolicy }
*
*/
public void setDataFocusPolicy(DataFocusPolicy value) {
this.dataFocusPolicy = value;
}
/**
* Gets the value of the finishPolicy property.
*
* @return
* possible object is
* {@link FinishPolicy }
*
*/
public FinishPolicy getFinishPolicy() {
return finishPolicy;
}
/**
* Sets the value of the finishPolicy property.
*
* @param value
* allowed object is
* {@link FinishPolicy }
*
*/
public void setFinishPolicy(FinishPolicy value) {
this.finishPolicy = value;
}
/**
* Gets the value of the trigger property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the trigger property.
*
*
* For example, to add a new item, do as follows:
*
* getTrigger().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Trigger }
*
*
*/
public List getTrigger() {
if (trigger == null) {
trigger = new ArrayList();
}
return this.trigger;
}
/**
* Gets the value of the transactionRef property.
*
* @return
* possible object is
* {@link TransactionRef }
*
*/
public TransactionRef getTransactionRef() {
return transactionRef;
}
/**
* Sets the value of the transactionRef property.
*
* @param value
* allowed object is
* {@link TransactionRef }
*
*/
public void setTransactionRef(TransactionRef value) {
this.transactionRef = value;
}
/**
* Gets the value of the roleRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the roleRef property.
*
*
* For example, to add a new item, do as follows:
*
* getRoleRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RoleRef }
*
*
*/
public List getRoleRef() {
if (roleRef == null) {
roleRef = new ArrayList();
}
return this.roleRef;
}
/**
* Gets the value of the usersRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the usersRef property.
*
*
* For example, to add a new item, do as follows:
*
* getUsersRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link UserRef }
*
*
*/
public List getUsersRef() {
if (usersRef == null) {
usersRef = new ArrayList();
}
return this.usersRef;
}
/**
* Gets the value of the userRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the userRef property.
*
*
* For example, to add a new item, do as follows:
*
* getUserRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link UserRef }
*
*
*/
public List getUserRef() {
if (userRef == null) {
userRef = new ArrayList();
}
return this.userRef;
}
/**
* Gets the value of the assignedUser property.
*
* @return
* possible object is
* {@link AssignedUser }
*
*/
public AssignedUser getAssignedUser() {
return assignedUser;
}
/**
* Sets the value of the assignedUser property.
*
* @param value
* allowed object is
* {@link AssignedUser }
*
*/
public void setAssignedUser(AssignedUser value) {
this.assignedUser = value;
}
/**
* Gets the value of the dataRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dataRef property.
*
*
* For example, to add a new item, do as follows:
*
* getDataRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DataRef }
*
*
*/
public List getDataRef() {
if (dataRef == null) {
dataRef = new ArrayList();
}
return this.dataRef;
}
/**
* Gets the value of the dataGroup property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dataGroup property.
*
*
* For example, to add a new item, do as follows:
*
* getDataGroup().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DataGroup }
*
*
*/
public List getDataGroup() {
if (dataGroup == null) {
dataGroup = new ArrayList();
}
return this.dataGroup;
}
/**
* Gets the value of the event property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the event property.
*
*
* For example, to add a new item, do as follows:
*
* getEvent().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Event }
*
*
*/
public List getEvent() {
if (event == null) {
event = new ArrayList();
}
return this.event;
}
}