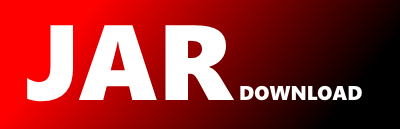
com.netgrif.application.engine.petrinet.domain.PetriNet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of application-engine Show documentation
Show all versions of application-engine Show documentation
System provides workflow management functions including user, role and data management.
package com.netgrif.application.engine.petrinet.domain;
import com.netgrif.application.engine.auth.domain.Author;
import com.netgrif.application.engine.petrinet.domain.arcs.Arc;
import com.netgrif.application.engine.petrinet.domain.arcs.reference.Referencable;
import com.netgrif.application.engine.petrinet.domain.arcs.reference.Type;
import com.netgrif.application.engine.petrinet.domain.dataset.Field;
import com.netgrif.application.engine.petrinet.domain.dataset.logic.action.Action;
import com.netgrif.application.engine.petrinet.domain.dataset.logic.action.runner.Expression;
import com.netgrif.application.engine.petrinet.domain.events.CaseEvent;
import com.netgrif.application.engine.petrinet.domain.events.CaseEventType;
import com.netgrif.application.engine.petrinet.domain.events.ProcessEvent;
import com.netgrif.application.engine.petrinet.domain.events.ProcessEventType;
import com.netgrif.application.engine.petrinet.domain.roles.ProcessRole;
import com.netgrif.application.engine.petrinet.domain.version.Version;
import com.netgrif.application.engine.workflow.domain.DataField;
import lombok.Getter;
import lombok.Setter;
import org.bson.types.ObjectId;
import org.springframework.data.annotation.Transient;
import org.springframework.data.mongodb.core.mapping.DBRef;
import org.springframework.data.mongodb.core.mapping.Document;
import java.time.LocalDateTime;
import java.util.*;
import java.util.stream.Collectors;
@Document
public class PetriNet extends PetriNetObject {
@Getter
@Setter
private String identifier; //combination of identifier and version must be unique ... maybe use @CompoundIndex?
@Getter
@Setter
private String uriNodeId;
@Getter
private I18nString title;
@Getter
@Setter
private boolean defaultRoleEnabled;
@Getter
@Setter
private boolean anonymousRoleEnabled;
@Getter
@Setter
private I18nString defaultCaseName;
@Getter
@Setter
private Expression defaultCaseNameExpression;
@Getter
@Setter
private String initials;
@Getter
@Setter
private String icon;
// TODO: 18. 3. 2017 replace with Spring auditing
@Getter
@Setter
private LocalDateTime creationDate;
@Getter
@Setter
private Version version;
@Getter
@Setter
private Author author;
@org.springframework.data.mongodb.core.mapping.Field("places")
@Getter
@Setter
private LinkedHashMap places;
@org.springframework.data.mongodb.core.mapping.Field("transitions")
@Getter
@Setter
private LinkedHashMap transitions;
@org.springframework.data.mongodb.core.mapping.Field("arcs")
@Getter
@Setter
private LinkedHashMap> arcs;//todo: import id
@org.springframework.data.mongodb.core.mapping.Field("dataset")
@Getter
@Setter
private LinkedHashMap dataSet;
@org.springframework.data.mongodb.core.mapping.Field("roles")
@DBRef
@Getter
@Setter
private LinkedHashMap roles;
@org.springframework.data.mongodb.core.mapping.Field("transactions")
@Getter
@Setter
private LinkedHashMap transactions;//todo: import id
@Getter
@Setter
private Map processEvents;
@Getter
@Setter
private Map caseEvents;
@Getter
@Setter
private Map> permissions;
@Getter
@Setter
private List negativeViewRoles;
@Getter
@Setter
private Map> userRefs;
@Getter
@Setter
private List functions;
@Transient
private boolean initialized;
@Getter
@Setter
private String importXmlPath;
@Getter
@Setter
private Map tags;
public PetriNet() {
this._id = new ObjectId();
this.identifier = "Default";
this.initials = "";
this.title = new I18nString("");
this.importId = "";
this.version = new Version();
defaultCaseName = new I18nString("");
initialized = false;
creationDate = LocalDateTime.now();
places = new LinkedHashMap<>();
transitions = new LinkedHashMap<>();
arcs = new LinkedHashMap<>();
dataSet = new LinkedHashMap<>();
roles = new LinkedHashMap<>();
negativeViewRoles = new LinkedList<>();
transactions = new LinkedHashMap<>();
processEvents = new LinkedHashMap<>();
caseEvents = new LinkedHashMap<>();
permissions = new HashMap<>();
userRefs = new HashMap<>();
functions = new LinkedList<>();
tags = new HashMap<>();
}
public void addPlace(Place place) {
this.places.put(place.getStringId(), place);
}
public void addTransition(Transition transition) {
this.transitions.put(transition.getStringId(), transition);
}
public void addRole(ProcessRole role) {
this.roles.put(role.getStringId(), role);
}
public void addPermission(String roleId, Map permissions) {
if (this.permissions.containsKey(roleId) && this.permissions.get(roleId) != null) {
this.permissions.get(roleId).putAll(permissions);
} else {
this.permissions.put(roleId, permissions);
}
}
public void addNegativeViewRole(String roleId) {
negativeViewRoles.add(roleId);
}
public void addFunction(Function function) {
functions.add(function);
}
public void addUserPermission(String usersRefId, Map permissions) {
if (this.userRefs.containsKey(usersRefId) && this.userRefs.get(usersRefId) != null) {
this.userRefs.get(usersRefId).putAll(permissions);
} else {
this.userRefs.put(usersRefId, permissions);
}
}
public List getArcsOfTransition(Transition transition) {
return getArcsOfTransition(transition.getStringId());
}
public List getArcsOfTransition(String transitionId) {
if (arcs.containsKey(transitionId)) {
return arcs.get(transitionId);
}
return new LinkedList<>();
}
public void addDataSetField(Field field) {
this.dataSet.put(field.getStringId(), field);
}
public boolean isNotInitialized() {
return !initialized;
}
public void addArc(Arc arc) {
String transitionId = arc.getTransition().getStringId();
if (arcs.containsKey(transitionId)) {
arcs.get(transitionId).add(arc);
} else {
List arcList = new LinkedList<>();
arcList.add(arc);
arcs.put(transitionId, arcList);
}
}
public Node getNode(String importId) {
if (places.containsKey(importId)) {
return getPlace(importId);
}
if (transitions.containsKey(importId)) {
return getTransition(importId);
}
return null;
}
public Optional getField(String id) {
return Optional.ofNullable(dataSet.get(id));
}
public Place getPlace(String id) {
return places.get(id);
}
public Transition getTransition(String id) {
return transitions.get(id);
}
public void initializeArcs() {
arcs.values().forEach(list -> list.forEach(arc -> {
arc.setSource(getNode(arc.getSourceId()));
arc.setDestination(getNode(arc.getDestinationId()));
}));
initialized = true;
}
public void initializeTokens(Map activePlaces) {
places.values().forEach(place -> place.setTokens(activePlaces.getOrDefault(place.getStringId(), 0)));
}
public void initializeArcs(Map dataSet) {
arcs.values()
.stream()
.flatMap(List::stream)
.filter(arc -> arc.getReference() != null)
.forEach(arc -> {
String referenceId = arc.getReference().getReference();
arc.getReference().setReferencable(getArcReference(referenceId, arc.getReference().getType(), dataSet));
});
}
private Referencable getArcReference(String referenceId, Type type, Map dataSet) {
if (type == Type.PLACE) {
return places.get(referenceId);
} else {
return dataSet.get(referenceId);
}
}
public Map getActivePlaces() {
Map activePlaces = new HashMap<>();
for (Place place : places.values()) {
if (place.getTokens() > 0) {
activePlaces.put(place.getStringId(), place.getTokens());
}
}
return activePlaces;
}
public void addTransaction(Transaction transaction) {
this.transactions.put(transaction.getStringId(), transaction);
}
public Transaction getTransactionByTransition(Transition transition) {
return transactions.values().stream()
.filter(transaction ->
transaction.getTransitions().contains(transition.getStringId())
).findAny().orElse(null);
}
public List getImmediateFields() {
return this.dataSet.values().stream().filter(Field::isImmediate).collect(Collectors.toList());
}
public boolean isDisplayableInAnyTransition(String fieldId) {
return transitions.values().stream().parallel().anyMatch(trans -> trans.isDisplayable(fieldId));
}
public void incrementVersion(VersionType type) {
this.version.increment(type);
}
@Override
public String toString() {
return title.toString();
}
public void setTitle(I18nString title) {
this.title = title;
}
public void setTitle(String title) {
setTitle(new I18nString(title));
}
public String getTranslatedDefaultCaseName(Locale locale) {
if (defaultCaseName == null) {
return "";
}
return defaultCaseName.getTranslation(locale);
}
public String getTranslatedTitle(Locale locale) {
if (title == null) {
return "";
}
return title.getTranslation(locale);
}
public List getFunctions(FunctionScope scope) {
return functions.stream().filter(function -> function.getScope().equals(scope)).collect(Collectors.toList());
}
public List getPreCreateActions() {
return getPreCaseActions(CaseEventType.CREATE);
}
public List getPostCreateActions() {
return getPostCaseActions(CaseEventType.CREATE);
}
public List getPreDeleteActions() {
return getPreCaseActions(CaseEventType.DELETE);
}
public List getPostDeleteActions() {
return getPostCaseActions(CaseEventType.DELETE);
}
public List getPreUploadActions() {
return getPreProcessActions(ProcessEventType.UPLOAD);
}
public List getPostUploadActions() {
return getPostProcessActions(ProcessEventType.UPLOAD);
}
private List getPreCaseActions(CaseEventType type) {
if (caseEvents.containsKey(type))
return caseEvents.get(type).getPreActions();
return new LinkedList<>();
}
private List getPostCaseActions(CaseEventType type) {
if (caseEvents.containsKey(type))
return caseEvents.get(type).getPostActions();
return new LinkedList<>();
}
private List getPreProcessActions(ProcessEventType type) {
if (processEvents.containsKey(type))
return processEvents.get(type).getPreActions();
return new LinkedList<>();
}
private List getPostProcessActions(ProcessEventType type) {
if (processEvents.containsKey(type))
return processEvents.get(type).getPostActions();
return new LinkedList<>();
}
public boolean hasDynamicCaseName() {
return defaultCaseNameExpression != null;
}
@Override
public String getStringId() {
return _id.toString();
}
public PetriNet clone() {
PetriNet clone = new PetriNet();
clone.setIdentifier(this.identifier);
clone.setUriNodeId(this.uriNodeId);
clone.setInitials(this.initials);
clone.setTitle(this.title.clone());
clone.setDefaultRoleEnabled(this.defaultRoleEnabled);
clone.setDefaultCaseName(this.defaultCaseName == null ? null : this.defaultCaseName.clone());
clone.setDefaultCaseNameExpression(this.defaultCaseNameExpression == null ? null : this.defaultCaseNameExpression.clone());
clone.setIcon(this.icon);
clone.setCreationDate(this.creationDate);
clone.setVersion(this.version == null ? null : this.version.clone());
clone.setAuthor(this.author == null ? null : this.author.clone());
clone.setTransitions(this.transitions == null ? null : this.transitions.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone(), (v1, v2) -> v1, LinkedHashMap::new)));
clone.setRoles(this.roles == null ? null : this.roles.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone(), (v1, v2) -> v1, LinkedHashMap::new)));
clone.setTransactions(this.transactions == null ? null : this.transactions.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone(), (v1, v2) -> v1, LinkedHashMap::new)));
clone.setImportXmlPath(this.importXmlPath);
clone.setImportId(this.importId);
clone.setObjectId(this._id);
clone.setDataSet(this.dataSet.entrySet()
.stream()
.collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone(), (x,y)->y, LinkedHashMap::new))
);
clone.setPlaces(this.places.entrySet()
.stream()
.collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone(), (x,y)->y, LinkedHashMap::new))
);
clone.setArcs(this.arcs.entrySet()
.stream()
.collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().stream()
.map(Arc::clone)
.collect(Collectors.toList()), (x,y)->y, LinkedHashMap::new))
);
clone.initializeArcs();
clone.setCaseEvents(this.caseEvents == null ? null : this.caseEvents.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone())));
clone.setProcessEvents(this.processEvents == null ? null : this.processEvents.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> e.getValue().clone())));
clone.setPermissions(this.permissions == null ? null : this.permissions.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> new HashMap<>(e.getValue()))));
clone.setUserRefs(this.userRefs == null ? null : this.userRefs.entrySet().stream().collect(Collectors.toMap(Map.Entry::getKey, e -> new HashMap<>(e.getValue()))));
this.getNegativeViewRoles().forEach(clone::addNegativeViewRole);
this.getFunctions().forEach(clone::addFunction);
clone.setTags(new HashMap<>(this.tags));
return clone;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy