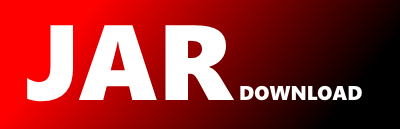
com.netopyr.reduxfx.component.impl.ComponentDriver Maven / Gradle / Ivy
package com.netopyr.reduxfx.component.impl;
import com.netopyr.reduxfx.component.ComponentBase;
import com.netopyr.reduxfx.component.command.FireEventCommand;
import com.netopyr.reduxfx.component.command.IntegerChangedCommand;
import com.netopyr.reduxfx.component.command.ObjectChangedCommand;
import com.netopyr.reduxfx.driver.Driver;
import com.netopyr.reduxfx.updater.Command;
import io.reactivex.Flowable;
import io.reactivex.processors.FlowableProcessor;
import io.reactivex.processors.PublishProcessor;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.ReadOnlyIntegerProperty;
import javafx.beans.property.ReadOnlyObjectProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.event.Event;
import javafx.event.EventHandler;
import org.reactivestreams.Publisher;
import org.reactivestreams.Subscriber;
import java.util.Objects;
import java.util.function.BiConsumer;
public class ComponentDriver implements Driver {
private static final String BEAN_MUST_NOT_BE_NULL = "Bean must not be null";
private static final String NAME_MUST_NOT_BE_NULL = "Name must not be null";
private final FlowableProcessor commandProcessor = PublishProcessor.create();
private final FlowableProcessor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy