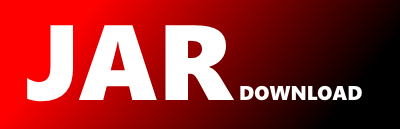
com.netopyr.reduxfx.driver.http.HttpDriver Maven / Gradle / Ivy
package com.netopyr.reduxfx.driver.http;
import com.netopyr.reduxfx.driver.Driver;
import com.netopyr.reduxfx.driver.http.command.HttpGetCommand;
import com.netopyr.reduxfx.driver.http.command.HttpPutCommand;
import com.netopyr.reduxfx.updater.Command;
import io.reactivex.processors.FlowableProcessor;
import io.reactivex.processors.PublishProcessor;
import io.vavr.control.Try;
import okhttp3.Headers;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import org.reactivestreams.Publisher;
import org.reactivestreams.Subscriber;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.atomic.AtomicInteger;
@SuppressWarnings("unused")
public class HttpDriver implements Driver {
private static final MediaType JSON = MediaType.parse("application/json; charset=utf-8");
private static final AtomicInteger THREAD_COUNTER = new AtomicInteger();
private static final ThreadFactory THREAD_FACTORY = runnable -> {
final Thread thread = new Thread(runnable, "HttpDriver-" + THREAD_COUNTER.incrementAndGet());
thread.setDaemon(true);
return thread;
};
private final OkHttpClient client;
private final ExecutorService executorService = Executors.newCachedThreadPool(THREAD_FACTORY);
private final FlowableProcessor commandSubscriber = PublishProcessor.create();
private final FlowableProcessor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy