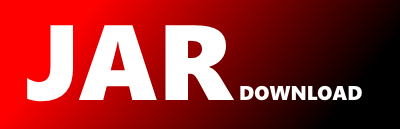
com.netopyr.reduxfx.vscenegraph.VNode Maven / Gradle / Ivy
package com.netopyr.reduxfx.vscenegraph;
import com.netopyr.reduxfx.vscenegraph.event.VEventHandler;
import com.netopyr.reduxfx.vscenegraph.event.VEventType;
import com.netopyr.reduxfx.vscenegraph.property.VProperty;
import io.vavr.collection.Array;
import io.vavr.collection.Map;
import io.vavr.control.Option;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Objects;
public class VNode {
private static final Logger LOG = LoggerFactory.getLogger(VNode.class);
private final Class> nodeClass;
private final Map> childrenMap;
private final Map> singleChildMap;
private final Map properties;
private final Map eventHandlers;
@SuppressWarnings("unchecked")
public VNode(Class> nodeClass,
Map> children,
Map> singleChildMap,
Map properties,
Map eventHandlers) {
this.nodeClass = Objects.requireNonNull(nodeClass, "NodeClass must not be null");
this.childrenMap = Objects.requireNonNull(children, "ChildrenMap must not be null");
this.singleChildMap = Objects.requireNonNull(singleChildMap, "SingleChildMap must not be null");
this.properties = Objects.requireNonNull(properties, "Properties must not be null");
this.eventHandlers = Objects.requireNonNull(eventHandlers, "EventHandlers must not be null");
}
public Option
© 2015 - 2025 Weber Informatics LLC | Privacy Policy