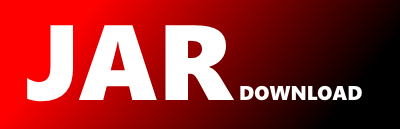
com.netopyr.reduxfx.vscenegraph.impl.differ.patches.AppendPatch Maven / Gradle / Ivy
package com.netopyr.reduxfx.vscenegraph.impl.differ.patches;
import com.netopyr.reduxfx.vscenegraph.VNode;
import io.vavr.collection.Vector;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.Objects;
public class AppendPatch extends Patch {
private final VNode newNode;
public AppendPatch(Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy