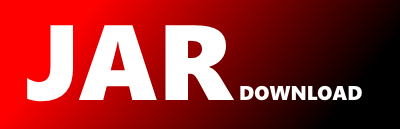
com.netopyr.reduxfx.vscenegraph.impl.differ.patches.AttributesPatch Maven / Gradle / Ivy
package com.netopyr.reduxfx.vscenegraph.impl.differ.patches;
import com.netopyr.reduxfx.vscenegraph.event.VEventHandler;
import com.netopyr.reduxfx.vscenegraph.event.VEventType;
import com.netopyr.reduxfx.vscenegraph.property.VProperty;
import io.vavr.collection.HashMap;
import io.vavr.collection.Map;
import io.vavr.collection.Vector;
import io.vavr.control.Option;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.Objects;
public class AttributesPatch extends Patch {
private final Map properties;
private final Map> eventHandlers;
public AttributesPatch(
Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy