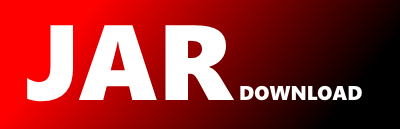
com.networknt.eventuate.kafka.consumer.TopicPartitionOffsets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eventuate-server-kafka Show documentation
Show all versions of eventuate-server-kafka Show documentation
A module that is responsible for Kafka communication
package com.networknt.eventuate.kafka.consumer;
import java.util.*;
import java.util.stream.Collectors;
/**
* Tracks the offsets for a TopicPartition that are being processed or have been processed
*/
public class TopicPartitionOffsets {
/**
* offsets that are being processed
*/
private SortedSet unprocessed = new TreeSet<>();
/**
* offsets that have been processed
*/
private Set processed = new HashSet<>();
@Override
public String toString() {
return "TopicPartitionOffsets{" +
"unprocessed=" + unprocessed +
", processed=" + processed +
'}';
}
public void noteUnprocessed(long offset) {
unprocessed.add(offset);
}
public void noteProcessed(long offset) {
processed.add(offset);
}
/**
* @return large of all offsets that have been processed and can be committed
*/
Optional offsetToCommit() {
Optional result = Optional.empty();
for (long x : unprocessed) {
if (processed.contains(x))
result = Optional.of(x);
else
break;
}
return result;
}
public void noteOffsetCommitted(long offset) {
unprocessed = new TreeSet<>(unprocessed.stream().filter(x -> x >= offset).collect(Collectors.toList()));
processed = processed.stream().filter(x -> x >= offset).collect(Collectors.toSet());
}
public Set getPending() {
Set result = new HashSet<>(unprocessed);
result.removeAll(processed);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy