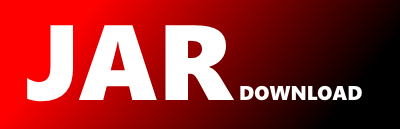
com.networknt.schema.ValidationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-schema-validator Show documentation
Show all versions of json-schema-validator Show documentation
A json schema validator that supports draft v4, v6, v7, v2019-09 and v2020-12
/*
* Copyright (c) 2016 Network New Technologies Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.networknt.schema;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import com.fasterxml.jackson.databind.JsonNode;
import com.networknt.schema.SpecVersion.VersionFlag;
public class ValidationContext {
private final JsonMetaSchema metaSchema;
private final JsonSchemaFactory jsonSchemaFactory;
private final SchemaValidatorsConfig config;
private final ConcurrentMap schemaReferences;
private final ConcurrentMap schemaResources;
private final ConcurrentMap dynamicAnchors;
public ValidationContext(JsonMetaSchema metaSchema,
JsonSchemaFactory jsonSchemaFactory, SchemaValidatorsConfig config) {
this(metaSchema, jsonSchemaFactory, config, new ConcurrentHashMap<>(), new ConcurrentHashMap<>(), new ConcurrentHashMap<>());
}
public ValidationContext(JsonMetaSchema metaSchema, JsonSchemaFactory jsonSchemaFactory,
SchemaValidatorsConfig config, ConcurrentMap schemaReferences,
ConcurrentMap schemaResources, ConcurrentMap dynamicAnchors) {
if (metaSchema == null) {
throw new IllegalArgumentException("JsonMetaSchema must not be null");
}
if (jsonSchemaFactory == null) {
throw new IllegalArgumentException("JsonSchemaFactory must not be null");
}
this.metaSchema = metaSchema;
this.jsonSchemaFactory = jsonSchemaFactory;
this.config = config == null ? new SchemaValidatorsConfig() : config;
this.schemaReferences = schemaReferences;
this.schemaResources = schemaResources;
this.dynamicAnchors = dynamicAnchors;
}
public JsonSchema newSchema(SchemaLocation schemaLocation, JsonNodePath evaluationPath, JsonNode schemaNode, JsonSchema parentSchema) {
return getJsonSchemaFactory().create(this, schemaLocation, evaluationPath, schemaNode, parentSchema);
}
public JsonValidator newValidator(SchemaLocation schemaLocation, JsonNodePath evaluationPath,
String keyword /* keyword */, JsonNode schemaNode, JsonSchema parentSchema) {
return this.metaSchema.newValidator(this, schemaLocation, evaluationPath, keyword, schemaNode, parentSchema);
}
public String resolveSchemaId(JsonNode schemaNode) {
return this.metaSchema.readId(schemaNode);
}
public JsonSchemaFactory getJsonSchemaFactory() {
return this.jsonSchemaFactory;
}
public SchemaValidatorsConfig getConfig() {
return this.config;
}
/**
* Gets the schema references identified by the ref uri.
*
* @return the schema references
*/
public ConcurrentMap getSchemaReferences() {
return this.schemaReferences;
}
/**
* Gets the schema resources identified by id.
*
* @return the schema resources
*/
public ConcurrentMap getSchemaResources() {
return this.schemaResources;
}
/**
* Gets the dynamic anchors.
*
* @return the dynamic anchors
*/
public ConcurrentMap getDynamicAnchors() {
return this.dynamicAnchors;
}
public JsonMetaSchema getMetaSchema() {
return this.metaSchema;
}
public Optional activeDialect() {
String metaSchema = getMetaSchema().getUri();
return SpecVersionDetector.detectOptionalVersion(metaSchema);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy