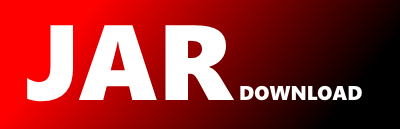
com.networknt.schema.output.OutputUnitData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-schema-validator Show documentation
Show all versions of json-schema-validator Show documentation
A json schema validator that supports draft v4, v6, v7, v2019-09 and v2020-12
/*
* Copyright (c) 2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.networknt.schema.output;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.networknt.schema.ExecutionContext;
import com.networknt.schema.SchemaLocation;
import com.networknt.schema.ValidationMessage;
import com.networknt.schema.annotation.JsonNodeAnnotation;
/**
* Output Unit Data.
*/
public class OutputUnitData {
private final Map valid = new LinkedHashMap<>();
private final Map> errors = new LinkedHashMap<>();
private final Map> annotations = new LinkedHashMap<>();
private final Map> droppedAnnotations = new LinkedHashMap<>();
public Map getValid() {
return valid;
}
public Map> getErrors() {
return errors;
}
public Map> getAnnotations() {
return annotations;
}
public Map> getDroppedAnnotations() {
return droppedAnnotations;
}
public static String formatMessage(String message) {
int index = message.indexOf(":");
if (index != -1) {
int length = message.length();
while (index + 1 < length) {
if (message.charAt(index + 1) == ' ') {
index++;
} else {
break;
}
}
return message.substring(index + 1);
}
return message;
}
@SuppressWarnings("unchecked")
public static OutputUnitData from(Set validationMessages, ExecutionContext executionContext) {
OutputUnitData data = new OutputUnitData();
Map valid = data.valid;
Map> errors = data.errors;
Map> annotations = data.annotations;
Map> droppedAnnotations = data.droppedAnnotations;
for (ValidationMessage assertion : validationMessages) {
SchemaLocation assertionSchemaLocation = new SchemaLocation(assertion.getSchemaLocation().getAbsoluteIri(),
assertion.getSchemaLocation().getFragment().getParent());
OutputUnitKey key = new OutputUnitKey(assertion.getEvaluationPath().getParent(),
assertionSchemaLocation, assertion.getInstanceLocation());
valid.put(key, false);
Map errorMap = errors.computeIfAbsent(key, k -> new LinkedHashMap<>());
Object value = errorMap.get(assertion.getType());
if (value == null) {
errorMap.put(assertion.getType(), formatMessage(assertion.getMessage()));
} else {
// Existing error, make it into a list
if (value instanceof List) {
((List) value).add(formatMessage(assertion.getMessage()));
} else {
List values = new ArrayList<>();
values.add(value.toString());
values.add(formatMessage(assertion.getMessage()));
errorMap.put(assertion.getType(), values);
}
}
}
for (List annotationsResult : executionContext.getAnnotations().asMap().values()) {
for (JsonNodeAnnotation annotation : annotationsResult) {
// As some annotations are required for computation, filter those that are not
// required for reporting
if (executionContext.getExecutionConfig().getAnnotationCollectionFilter()
.test(annotation.getKeyword())) {
SchemaLocation annotationSchemaLocation = new SchemaLocation(
annotation.getSchemaLocation().getAbsoluteIri(),
annotation.getSchemaLocation().getFragment().getParent());
OutputUnitKey key = new OutputUnitKey(annotation.getEvaluationPath().getParent(),
annotationSchemaLocation, annotation.getInstanceLocation());
boolean validResult = executionContext.getResults().isValid(annotation.getInstanceLocation(),
annotation.getEvaluationPath());
valid.put(key, validResult);
if (validResult) {
// annotations
Map annotationMap = annotations.computeIfAbsent(key,
k -> new LinkedHashMap<>());
annotationMap.put(annotation.getKeyword(), annotation.getValue());
} else {
// dropped annotations
Map droppedAnnotationMap = droppedAnnotations.computeIfAbsent(key,
k -> new LinkedHashMap<>());
droppedAnnotationMap.put(annotation.getKeyword(), annotation.getValue());
}
}
}
}
return data;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy