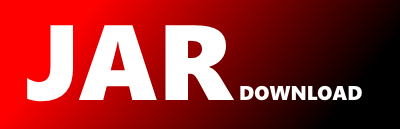
templates.graphql.serverYml Maven / Gradle / Ivy
package templates.graphql;
import java.io.IOException;
import com.fizzed.rocker.ForIterator;
import com.fizzed.rocker.RenderingException;
import com.fizzed.rocker.RockerContent;
import com.fizzed.rocker.RockerOutput;
import com.fizzed.rocker.runtime.DefaultRockerTemplate;
import com.fizzed.rocker.runtime.PlainTextUnloadedClassLoader;
/*
* Auto generated code to render template templates/graphql/serverYml.rocker.raw
* Do not edit this file. Changes will eventually be overwritten by Rocker parser!
*/
@SuppressWarnings("unused")
public class serverYml extends com.fizzed.rocker.runtime.DefaultRockerModel {
static public com.fizzed.rocker.ContentType getContentType() { return com.fizzed.rocker.ContentType.RAW; }
static public String getTemplateName() { return "serverYml.rocker.raw"; }
static public String getTemplatePackageName() { return "templates.graphql"; }
static public String getHeaderHash() { return "-165946214"; }
static public long getModifiedAt() { return 1646166277519L; }
static public String[] getArgumentNames() { return new String[] { "serviceId", "enableHttp", "httpPort", "enableHttps", "httpsPort", "enableHttp2", "enableRegistry", "version" }; }
// argument @ [1:2]
private String serviceId;
// argument @ [1:2]
private Boolean enableHttp;
// argument @ [1:2]
private String httpPort;
// argument @ [1:2]
private Boolean enableHttps;
// argument @ [1:2]
private String httpsPort;
// argument @ [1:2]
private Boolean enableHttp2;
// argument @ [1:2]
private Boolean enableRegistry;
// argument @ [1:2]
private String version;
public serverYml serviceId(String serviceId) {
this.serviceId = serviceId;
return this;
}
public String serviceId() {
return this.serviceId;
}
public serverYml enableHttp(Boolean enableHttp) {
this.enableHttp = enableHttp;
return this;
}
public Boolean enableHttp() {
return this.enableHttp;
}
public serverYml httpPort(String httpPort) {
this.httpPort = httpPort;
return this;
}
public String httpPort() {
return this.httpPort;
}
public serverYml enableHttps(Boolean enableHttps) {
this.enableHttps = enableHttps;
return this;
}
public Boolean enableHttps() {
return this.enableHttps;
}
public serverYml httpsPort(String httpsPort) {
this.httpsPort = httpsPort;
return this;
}
public String httpsPort() {
return this.httpsPort;
}
public serverYml enableHttp2(Boolean enableHttp2) {
this.enableHttp2 = enableHttp2;
return this;
}
public Boolean enableHttp2() {
return this.enableHttp2;
}
public serverYml enableRegistry(Boolean enableRegistry) {
this.enableRegistry = enableRegistry;
return this;
}
public Boolean enableRegistry() {
return this.enableRegistry;
}
public serverYml version(String version) {
this.version = version;
return this;
}
public String version() {
return this.version;
}
static public serverYml template(String serviceId, Boolean enableHttp, String httpPort, Boolean enableHttps, String httpsPort, Boolean enableHttp2, Boolean enableRegistry, String version) {
return new serverYml()
.serviceId(serviceId)
.enableHttp(enableHttp)
.httpPort(httpPort)
.enableHttps(enableHttps)
.httpsPort(httpsPort)
.enableHttp2(enableHttp2)
.enableRegistry(enableRegistry)
.version(version);
}
@Override
protected DefaultRockerTemplate buildTemplate() throws RenderingException {
// optimized for convenience (runtime auto reloading enabled if rocker.reloading=true)
return com.fizzed.rocker.runtime.RockerRuntime.getInstance().getBootstrap().template(this.getClass(), this);
}
static public class Template extends com.fizzed.rocker.runtime.DefaultRockerTemplate {
// \n# Server configuration\n---\n# This is the default binding address if the service is dockerized.\nip: ${server.ip:0.0.0.0}\n\n# Http port if enableHttp is true. It will be ignored if dynamicPort is true.\nhttpPort: ${server.httpPort:
static private final byte[] PLAIN_TEXT_0_0;
// }\n\n# Enable HTTP should be false by default. It should be only used for testing with clients or tools\n# that don't support https or very hard to import the certificate. Otherwise, https should be used.\n# When enableHttp, you must set enableHttps to false, otherwise, this flag will be ignored. There is\n# only one protocol will be used for the server at anytime. If both http and https are true, only\n# https listener will be created and the server will bind to https port only.\nenableHttp:...
static private final byte[] PLAIN_TEXT_1_0;
// }\n\n# Https port if enableHttps is true. It will be ignored if dynamicPort is true.\nhttpsPort: ${server.httpsPort:
static private final byte[] PLAIN_TEXT_2_0;
// }\n\n# Enable HTTPS should be true on official environment and most dev environments.\nenableHttps: ${server.enableHttps:
static private final byte[] PLAIN_TEXT_3_0;
// }\n\n# Http/2 is enabled by default for better performance and it works with the client module\n# Please note that HTTP/2 only works with HTTPS.\nenableHttp2: ${server.enableHttp2:
static private final byte[] PLAIN_TEXT_4_0;
// }\n\n# Keystore file name in config folder.\nkeystoreName: ${server.keystoreName:server.keystore}\n\n# Keystore password\nkeystorePass: ${server.keystorePass:password}\n\n# Private key password\nkeyPass: ${server.keyPass:password}\n\n# Flag that indicate if two way TLS is enabled. Not recommended in docker container.\nenableTwoWayTls: ${server.enableTwoWayTls:false}\n\n# Truststore file name in config folder.\ntruststoreName: ${server.truststoreName:server.truststore}\n\n# Truststore password...
static private final byte[] PLAIN_TEXT_5_0;
// }\n\n# Flag to enable self service registration. This should be turned on on official test and production. And\n# dyanmicPort should be enabled if any orchestration tool is used like Kubernetes.\nenableRegistry: ${server.enableRegistry:
static private final byte[] PLAIN_TEXT_6_0;
// }\n\n# When enableRegistry is true and the registry/discovery service is not reachable. Stop the server or continue\n# starting the server. When your global registry is not setup as high availability and only for monitoring, you\n# can set it true. If you are using it for global service discovery, leave it with false.\nstartOnRegistryFailure: ${server.startOnRegistryFailure:false}\n\n# Dynamic port is used in situation that multiple services will be deployed on the same host and normally\n# y...
static private final byte[] PLAIN_TEXT_7_0;
// }\n\n# Shutdown gracefully wait period in milliseconds\n# In this period, it allows the in-flight requests to complete but new requests are not allowed. It needs to be set\n# based on the slowest request possible.\nshutdownGracefulPeriod: ${server.shutdownGracefulPeriod:2000}\n\n# -----------------------------------------------------------------------------------------------------------\n# The following parameters are for advanced users to fine tune the service in a container environment. Ple...
static private final byte[] PLAIN_TEXT_8_0;
static {
PlainTextUnloadedClassLoader loader = PlainTextUnloadedClassLoader.tryLoad(serverYml.class.getClassLoader(), serverYml.class.getName() + "$PlainText", "UTF-8");
PLAIN_TEXT_0_0 = loader.tryGet("PLAIN_TEXT_0_0");
PLAIN_TEXT_1_0 = loader.tryGet("PLAIN_TEXT_1_0");
PLAIN_TEXT_2_0 = loader.tryGet("PLAIN_TEXT_2_0");
PLAIN_TEXT_3_0 = loader.tryGet("PLAIN_TEXT_3_0");
PLAIN_TEXT_4_0 = loader.tryGet("PLAIN_TEXT_4_0");
PLAIN_TEXT_5_0 = loader.tryGet("PLAIN_TEXT_5_0");
PLAIN_TEXT_6_0 = loader.tryGet("PLAIN_TEXT_6_0");
PLAIN_TEXT_7_0 = loader.tryGet("PLAIN_TEXT_7_0");
PLAIN_TEXT_8_0 = loader.tryGet("PLAIN_TEXT_8_0");
}
// argument @ [1:2]
protected final String serviceId;
// argument @ [1:2]
protected final Boolean enableHttp;
// argument @ [1:2]
protected final String httpPort;
// argument @ [1:2]
protected final Boolean enableHttps;
// argument @ [1:2]
protected final String httpsPort;
// argument @ [1:2]
protected final Boolean enableHttp2;
// argument @ [1:2]
protected final Boolean enableRegistry;
// argument @ [1:2]
protected final String version;
public Template(serverYml model) {
super(model);
__internal.setCharset("UTF-8");
__internal.setContentType(getContentType());
__internal.setTemplateName(getTemplateName());
__internal.setTemplatePackageName(getTemplatePackageName());
this.serviceId = model.serviceId();
this.enableHttp = model.enableHttp();
this.httpPort = model.httpPort();
this.enableHttps = model.enableHttps();
this.httpsPort = model.httpsPort();
this.enableHttp2 = model.enableHttp2();
this.enableRegistry = model.enableRegistry();
this.version = model.version();
}
@Override
protected void __doRender() throws IOException, RenderingException {
// PlainText @ [1:162]
__internal.aboutToExecutePosInTemplate(1, 162);
__internal.writeValue(PLAIN_TEXT_0_0);
// ValueExpression @ [8:29]
__internal.aboutToExecutePosInTemplate(8, 29);
__internal.renderValue(httpPort, false);
// PlainText @ [8:12]
__internal.aboutToExecutePosInTemplate(8, 12);
__internal.writeValue(PLAIN_TEXT_1_0);
// ValueExpression @ [15:33]
__internal.aboutToExecutePosInTemplate(15, 33);
__internal.renderValue(enableHttp, false);
// PlainText @ [15:14]
__internal.aboutToExecutePosInTemplate(15, 14);
__internal.writeValue(PLAIN_TEXT_2_0);
// ValueExpression @ [18:31]
__internal.aboutToExecutePosInTemplate(18, 31);
__internal.renderValue(httpsPort, false);
// PlainText @ [18:13]
__internal.aboutToExecutePosInTemplate(18, 13);
__internal.writeValue(PLAIN_TEXT_3_0);
// ValueExpression @ [21:35]
__internal.aboutToExecutePosInTemplate(21, 35);
__internal.renderValue(enableHttps, false);
// PlainText @ [21:15]
__internal.aboutToExecutePosInTemplate(21, 15);
__internal.writeValue(PLAIN_TEXT_4_0);
// ValueExpression @ [25:35]
__internal.aboutToExecutePosInTemplate(25, 35);
__internal.renderValue(enableHttp2, false);
// PlainText @ [25:15]
__internal.aboutToExecutePosInTemplate(25, 15);
__internal.writeValue(PLAIN_TEXT_5_0);
// ValueExpression @ [52:31]
__internal.aboutToExecutePosInTemplate(52, 31);
__internal.renderValue(serviceId, false);
// PlainText @ [52:13]
__internal.aboutToExecutePosInTemplate(52, 13);
__internal.writeValue(PLAIN_TEXT_6_0);
// ValueExpression @ [56:41]
__internal.aboutToExecutePosInTemplate(56, 41);
__internal.renderValue(enableRegistry, false);
// PlainText @ [56:18]
__internal.aboutToExecutePosInTemplate(56, 18);
__internal.writeValue(PLAIN_TEXT_7_0);
// ValueExpression @ [84:35]
__internal.aboutToExecutePosInTemplate(84, 35);
__internal.renderValue(version, false);
// PlainText @ [84:15]
__internal.aboutToExecutePosInTemplate(84, 15);
__internal.writeValue(PLAIN_TEXT_8_0);
}
}
private static class PlainText {
static private final String PLAIN_TEXT_0_0 = "\n# Server configuration\n---\n# This is the default binding address if the service is dockerized.\nip: ${server.ip:0.0.0.0}\n\n# Http port if enableHttp is true. It will be ignored if dynamicPort is true.\nhttpPort: ${server.httpPort:";
static private final String PLAIN_TEXT_1_0 = "}\n\n# Enable HTTP should be false by default. It should be only used for testing with clients or tools\n# that don't support https or very hard to import the certificate. Otherwise, https should be used.\n# When enableHttp, you must set enableHttps to false, otherwise, this flag will be ignored. There is\n# only one protocol will be used for the server at anytime. If both http and https are true, only\n# https listener will be created and the server will bind to https port only.\nenableHttp: ${server.enableHttp:";
static private final String PLAIN_TEXT_2_0 = "}\n\n# Https port if enableHttps is true. It will be ignored if dynamicPort is true.\nhttpsPort: ${server.httpsPort:";
static private final String PLAIN_TEXT_3_0 = "}\n\n# Enable HTTPS should be true on official environment and most dev environments.\nenableHttps: ${server.enableHttps:";
static private final String PLAIN_TEXT_4_0 = "}\n\n# Http/2 is enabled by default for better performance and it works with the client module\n# Please note that HTTP/2 only works with HTTPS.\nenableHttp2: ${server.enableHttp2:";
static private final String PLAIN_TEXT_5_0 = "}\n\n# Keystore file name in config folder.\nkeystoreName: ${server.keystoreName:server.keystore}\n\n# Keystore password\nkeystorePass: ${server.keystorePass:password}\n\n# Private key password\nkeyPass: ${server.keyPass:password}\n\n# Flag that indicate if two way TLS is enabled. Not recommended in docker container.\nenableTwoWayTls: ${server.enableTwoWayTls:false}\n\n# Truststore file name in config folder.\ntruststoreName: ${server.truststoreName:server.truststore}\n\n# Truststore password\ntruststorePass: ${server.truststorePass:password}\n\n# Bootstrap truststore name used to connect to the light-config-server if it is used.\nbootstrapStoreName: ${server.bootstrapStoreName:bootstrap.truststore}\n\n# Bootstrap truststore password\nbootstrapStorePass: ${server.bootstrapStorePass:password}\n\n# Unique service identifier. Used in service registration and discovery etc.\nserviceId: ${server.serviceId:";
static private final String PLAIN_TEXT_6_0 = "}\n\n# Flag to enable self service registration. This should be turned on on official test and production. And\n# dyanmicPort should be enabled if any orchestration tool is used like Kubernetes.\nenableRegistry: ${server.enableRegistry:";
static private final String PLAIN_TEXT_7_0 = "}\n\n# When enableRegistry is true and the registry/discovery service is not reachable. Stop the server or continue\n# starting the server. When your global registry is not setup as high availability and only for monitoring, you\n# can set it true. If you are using it for global service discovery, leave it with false.\nstartOnRegistryFailure: ${server.startOnRegistryFailure:false}\n\n# Dynamic port is used in situation that multiple services will be deployed on the same host and normally\n# you will have enableRegistry set to true so that other services can find the dynamic port service. When\n# deployed to Kubernetes cluster, the Pod must be annotated as hostNetwork: true\ndynamicPort: ${server.dynamicPort:false}\n\n# Minimum port range. This define a range for the dynamic allocated ports so that it is easier to setup\n# firewall rule to enable this range. Default 2400 to 2500 block has 100 port numbers and should be\n# enough for most cases unless you are using a big bare metal box as Kubernetes node that can run 1000s pods\nminPort: ${server.minPort:2400}\n\n# Maximum port rang. The range can be customized to adopt your network security policy and can be increased or\n# reduced to ease firewall rules.\nmaxPort: ${server.maxPort:2500}\n\n# environment tag that will be registered on consul to support multiple instances per env for testing.\n# https://github.com/networknt/light-doc/blob/master/docs/content/design/env-segregation.md\n# This tag should only be set for testing env, not production. The production certification process will enforce it.\n# environment: ${server.environment:test1}\n\n# Build Number, to be set by teams for auditing or tracing purposes. \n# Allows teams to audit the value and set it according to their release management process\nbuildNumber: ${server.buildNumber:";
static private final String PLAIN_TEXT_8_0 = "}\n\n# Shutdown gracefully wait period in milliseconds\n# In this period, it allows the in-flight requests to complete but new requests are not allowed. It needs to be set\n# based on the slowest request possible.\nshutdownGracefulPeriod: ${server.shutdownGracefulPeriod:2000}\n\n# -----------------------------------------------------------------------------------------------------------\n# The following parameters are for advanced users to fine tune the service in a container environment. Please leave\n# these values default if you do not understand. For more info, visit https://doc.networknt.com/concern/server/\n\n# Unique service name. Used in microservice to associate a given name to a service with configuration\n# or as a key within the configuration of a particular domain\n# serviceName: ${server.serviceName:petstore}\n\n# Buffer size of undertow server. Default to 16K\n# bufferSize: ${server.bufferSize:16384}\n\n# Number of IO thread. Default to number of processor * 2\n# ioThreads: ${server.ioThreads:4}\n\n# Number of worker threads. Default to 200 and it can be reduced to save memory usage in a container with only one cpu\n# workerThreads: ${server.workerThreads:200}\n\n# Backlog size. Default to 10000\n# backlog: ${server.backlog:10000}\n\n# Flag to set UndertowOptions.ALWAYS_SET_DATE\n# alwaysSetDate: ${server.alwaysSetDate:false}\n\n# Server string used to mark the server. Default to L for light-4j.\n# serverString: ${server.serverString:L}\n\n# Flag to set UndertowOptions.ALLOW_UNESCAPED_CHARACTERS_IN_URL. Default to false.\n# Please note that this option widens the attack surface and attacker can potentially access your filesystem.\n# This should only be used on an internal server and never be used on a server accessed from the Internet.\n# allowUnescapedCharactersInUrl: ${server.allowUnescapedCharactersInUrl:false}\n\n# Set the max transfer file size for uploading files. Default to 1000000 which is 1 MB.\n# maxTransferFileSize: ${server.maxTransferFileSize:1000000}\n";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy