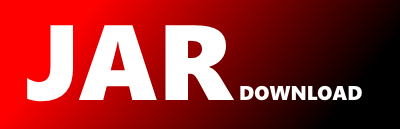
com.networknt.oas.jsonoverlay.ChildListOverlay Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-parser Show documentation
Show all versions of openapi-parser Show documentation
A light-weight, fast OpenAPI 3.0 parser and validator
/*******************************************************************************
* Copyright (c) 2017 ModelSolv, Inc. and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* ModelSolv, Inc. - initial API and implementation and/or initial documentation
*******************************************************************************/
package com.networknt.oas.jsonoverlay;
import com.fasterxml.jackson.core.JsonPointer;
import com.fasterxml.jackson.databind.JsonNode;
import java.util.Collection;
public class ChildListOverlay> extends ChildOverlay, ListOverlay> {
private ListOverlay listOverlay;
public ChildListOverlay(String path, JsonNode json, JsonOverlay> parent,
OverlayFactory, ListOverlay> factory, ReferenceRegistry refReg) {
super(path, json, parent, factory, refReg);
setListOverlay();
}
public ChildListOverlay(String path, Collection value, JsonOverlay> parent,
OverlayFactory, ListOverlay> factory, ReferenceRegistry refReg) {
super(path, value, parent, factory, refReg);
setListOverlay();
}
private void setListOverlay() {
@SuppressWarnings("unchecked")
ListOverlay castOverlay = (ListOverlay) overlay;
this.listOverlay = castOverlay;
}
@Override
protected boolean matchesPath(JsonPointer path) {
if (super.matchesPath(path)) {
int index = super.tailPath(path).getMatchingIndex();
return index >= 0;
} else {
return false;
}
}
public V get(int index) {
return listOverlay.get(index);
}
public void set(int index, V value) {
listOverlay.set(index, value);
}
public void add(V value) {
listOverlay.add(value);
}
public void insert(int index, V value) {
listOverlay.insert(index, value);
}
public void remove(int index) {
listOverlay.remove(index);
}
public int size() {
return listOverlay.size();
}
public ChildOverlay getChild(int index) {
@SuppressWarnings("unchecked")
ChildOverlay child = (ChildOverlay) listOverlay.getOverlay(index);
return child;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy