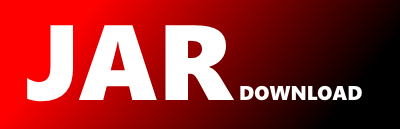
com.networknt.oas.jsonoverlay.JsonPointerTrie Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-parser Show documentation
Show all versions of openapi-parser Show documentation
A light-weight, fast OpenAPI 3.0 parser and validator
/*******************************************************************************
* Copyright (c) 2017 ModelSolv, Inc. and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* ModelSolv, Inc. - initial API and implementation and/or initial documentation
*******************************************************************************/
package com.networknt.oas.jsonoverlay;
import com.fasterxml.jackson.core.JsonPointer;
import org.apache.commons.lang3.tuple.Pair;
import java.util.HashMap;
import java.util.Map;
import java.util.regex.Pattern;
public class JsonPointerTrie {
private Map patternMap = new HashMap<>();
private Map> children = new HashMap<>();
public void add(JsonPointer path, Pattern pattern, T value) {
if (path.matches()) {
// note: pattern = null works with HashMap and is how a path that terminates in
// this object will be handled. When pattern is not null, a nonempty path will
// have its head segment matched against non-null patterns to choose the value.
patternMap.put(pattern, value);
} else {
String childKey = path.getMatchingProperty();
if (!children.containsKey(childKey)) {
children.put(childKey, new JsonPointerTrie());
}
children.get(childKey).add(path.tail(), pattern, value);
}
}
public Pair find(JsonPointer path) {
if (path.matches()) {
return Pair.of(patternMap.get(null), path);
} else {
String key = path.getMatchingProperty();
if (children.containsKey(key)) {
return children.get(key).find(path.tail());
}
// see if any non-null pattern matches key, return our T value with the same
// JsonPointer. This case addresses objects that implenent maps with
// pattern-constrained keys. We resolve to that map-like object, and the
// remaining path starts with a key into that map.
for (Pattern pat : patternMap.keySet()) {
if (pat != null && pat.matcher(key).matches()) {
return Pair.of(patternMap.get(pat), path);
}
}
// no non-null patterns matched - return our null-pattern value, with the
// remaining path to be applied to it.
return Pair.of(patternMap.get(null), path);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy